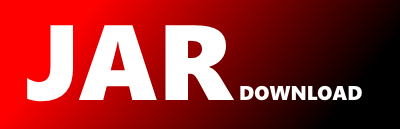
org.ow2.util.maven.plugin.rmic.SunRmicProxy Maven / Gradle / Ivy
/**
* OW2 Util
* Copyright (C) 2007 Bull S.A.S.
* Contact: [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307
* USA
*
* --------------------------------------------------------------------------
* $Id: SunRmicProxy.java 4389 2008-12-15 13:48:57Z alitokmen $
* --------------------------------------------------------------------------
*/
/*
* Copyright 2005-2006 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ow2.util.maven.plugin.rmic;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.lang.reflect.Constructor;
import java.lang.reflect.Method;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.ArrayList;
import java.util.List;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.Project;
import org.apache.tools.ant.taskdefs.LogOutputStream;
import org.apache.tools.ant.taskdefs.rmic.DefaultRmicAdapter;
import org.apache.tools.ant.types.Commandline;
/**
* The implementation of the rmic for SUN's JDK.
* @author Gael Lalire
*/
public class SunRmicProxy extends DefaultRmicAdapter {
/**
* Name of the rmic class.
*/
public static final String RMIC_CLASSNAME = "sun.rmi.rmic.Main";
/**
* name of the executable.
*/
public static final String RMIC_EXECUTABLE = "rmic";
/**
* Error message to use with the sun rmic is not the classpath.
*/
public static final String ERROR_NO_RMIC_ON_CLASSPATH = "Cannot use SUN rmic, as it is not "
+ "available. A common solution is to " + "set the environment variable " + "JAVA_HOME or CLASSPATH.";
/**
* Error message to use when there is an error starting the sun rmic
* compiler.
*/
public static final String ERROR_RMIC_FAILED = "Error starting SUN rmic: ";
/**
* Run the rmic compiler.
*
* @return true if the compilation succeeded
* @throws BuildException on error
*/
public boolean execute() throws BuildException {
getRmic().log("Using SUN rmic compiler", Project.MSG_VERBOSE);
Commandline cmd = setupRmicCommand();
// Create an instance of the rmic, redirecting output to
// the project log
LogOutputStream logstr = new LogOutputStream(getRmic(), Project.MSG_WARN);
try {
Class> c = Class.forName(RMIC_CLASSNAME, true, getClassLoaderForComSunPackage());
Constructor> cons = c.getConstructor(new Class[] {OutputStream.class, String.class});
Object rmic = cons.newInstance(new Object[] {logstr, "rmic"});
Method doRmic = c.getMethod("compile", new Class[] {String[].class});
Boolean ok = (Boolean) doRmic.invoke(rmic, (new Object[] {cmd.getArguments()}));
return ok.booleanValue(); // avoid exception
} catch (ClassNotFoundException ex) {
throw new BuildException(ERROR_NO_RMIC_ON_CLASSPATH, getRmic().getLocation());
} catch (Exception ex) {
if (ex instanceof BuildException) {
throw (BuildException) ex;
} else {
throw new BuildException(ERROR_RMIC_FAILED, ex, getRmic().getLocation());
}
} finally {
try {
logstr.close();
} catch (IOException e) {
throw new BuildException(e);
}
}
}
/**
* Construct a ClassLoader which can load com.sun package.
* @return the ClassLoader to use.
*/
private ClassLoader getClassLoaderForComSunPackage() {
File[] ftab = new File[] {
new File(System.getProperty("java.home") + File.separator + ".." + File.separator + "lib" + File.separator
+ "tools.jar"),
new File(System.getProperty("java.home") + File.separator + ".." + File.separator + "lib" + File.separator
+ "classes.zip"), new File("tools.jar"), new File("classes.zip")
};
ClassLoader currentClassLoader = this.getClass().getClassLoader();
try {
Class.forName(RMIC_CLASSNAME, true, currentClassLoader);
return currentClassLoader;
} catch (ClassNotFoundException e) {
int i = 0;
while (i < ftab.length) {
File f = ftab[i];
if (f.exists()) {
try {
URLClassLoader ucl = new URLClassLoader(new URL[] {f.toURL()}, currentClassLoader);
Class.forName(RMIC_CLASSNAME, true, ucl);
return ucl;
} catch (MalformedURLException mue) {
getRmic().log("Bad url (should not append) : " + mue, Project.MSG_ERR);
} catch (ClassNotFoundException cnfe) {
getRmic().log("Unable to find rmic class with current loader (check next if exists)", Project.MSG_DEBUG);
}
}
i++;
}
return currentClassLoader;
}
}
/**
* Strip out all -J args from the command list.
*
* @param compilerArgs
* the original compiler arguments
* @return the filtered set.
*/
protected String[] preprocessCompilerArgs(final String[] compilerArgs) {
int len = compilerArgs.length;
List args = new ArrayList(len);
for (int i = 0; i < len; i++) {
String arg = compilerArgs[i];
if (!arg.startsWith("-J")) {
args.add(arg);
} else {
getRmic().log("Dropping " + arg + " from compiler arguments");
}
}
int count = args.size();
return args.toArray(new String[count]);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy