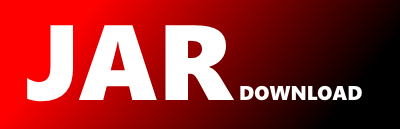
org.ow2.weblab.content.impl.webdav.WebDAVContentManager Maven / Gradle / Ivy
/**
* WEBLAB: Service oriented integration platform for media mining and intelligence applications
*
* Copyright (C) 2004 - 2011 CASSIDIAN an EADS Company
*
* This library is free software; you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License along with this
* library; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth
* Floor, Boston, MA 02110-1301 USA
*/
package org.ow2.weblab.content.impl.webdav;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.net.URISyntaxException;
import org.apache.commons.httpclient.Credentials;
import org.apache.commons.httpclient.HostConfiguration;
import org.apache.commons.httpclient.HttpClient;
import org.apache.commons.httpclient.HttpConnectionManager;
import org.apache.commons.httpclient.HttpException;
import org.apache.commons.httpclient.MultiThreadedHttpConnectionManager;
import org.apache.commons.httpclient.UsernamePasswordCredentials;
import org.apache.commons.httpclient.auth.AuthScope;
import org.apache.commons.httpclient.methods.GetMethod;
import org.apache.commons.httpclient.methods.InputStreamRequestEntity;
import org.apache.commons.httpclient.params.HttpConnectionManagerParams;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.IOUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.jackrabbit.webdav.DavException;
import org.apache.jackrabbit.webdav.client.methods.PutMethod;
import org.ow2.weblab.content.api.ContentManager;
import org.ow2.weblab.content.api.ContentReader;
import org.ow2.weblab.content.api.ContentWriter;
import org.ow2.weblab.core.extended.exception.WebLabCheckedException;
import org.ow2.weblab.core.extended.exception.WebLabNotYetImplementedException;
import org.ow2.weblab.core.extended.exception.WebLabUncheckedException;
import org.ow2.weblab.core.model.Resource;
import org.ow2.weblab.rdf.Value;
import org.purl.dc.elements.DublinCoreAnnotator;
public class WebDAVContentManager implements ContentReader, ContentWriter {
public Log logger = LogFactory.getLog(WebDAVContentManager.class);
public static final String WEBDAV_PROPERTY_FILE = "webdavContentManager.properties";
public static final String WEBDAV_TMP_FOLDER_PATH = "webdav.tmpFolder";
public static final String WEBDAV_HOST = "webdav.host";
public static final String WEBDAV_TIMEOUT = "webdav.timeout";
public static final String WEBDAV_MAXHOSTCONNECTIONS = "webdav.maxHostConnections";
public static final String WEBDAV_PASSWORD = "webdav.password";
public static final String WEBDAV_USERNAME = "webdav.username";
public static final String PREFIX = "weblab-";
public static final String SUFFIX = ".content";
public static int cpt = 0;
final private String webdavHost;
private final int webdavTimeOut;
private final int webdavMaxHostConnections;
private final String webdavUserName;
private final String webdavPassword;
final private File tmpFolder;
public WebDAVContentManager() {
this.tmpFolder = new File(ContentManager.getPropertyValue(WebDAVContentManager.WEBDAV_PROPERTY_FILE, WebDAVContentManager.WEBDAV_TMP_FOLDER_PATH,
"/tmp"));
try {
FileUtils.forceMkdir(this.tmpFolder);
} catch (final IOException ioe) {
throw new WebLabUncheckedException("Unable to create folder [" + this.tmpFolder + "].", ioe);
}
if (!this.tmpFolder.exists()) {
throw new WebLabUncheckedException("Content folder [" + this.tmpFolder + "] does not exists or is not readable.");
}
if (!this.tmpFolder.canWrite()) {
throw new WebLabUncheckedException("Cannot write in content folder [" + this.tmpFolder + "].");
}
if (this.tmpFolder.isFile()) {
throw new WebLabUncheckedException("Content folder [" + this.tmpFolder + "] is a File instead of a directory.");
}
this.logger.info(WebDAVContentManager.WEBDAV_TMP_FOLDER_PATH + "=" + this.tmpFolder.getAbsolutePath());
final String host = ContentManager.getPropertyValue(WebDAVContentManager.WEBDAV_PROPERTY_FILE, WebDAVContentManager.WEBDAV_HOST, null);
if (!host.endsWith("/")) {
this.webdavHost = host + '/';
} else {
this.webdavHost = host;
}
this.logger.info(WebDAVContentManager.WEBDAV_HOST + "=" + this.webdavHost);
this.webdavTimeOut = Integer.parseInt(ContentManager.getPropertyValue(WebDAVContentManager.WEBDAV_PROPERTY_FILE, WebDAVContentManager.WEBDAV_TIMEOUT,
Integer.toString(30000)));
this.webdavMaxHostConnections = Integer.parseInt(ContentManager.getPropertyValue(WebDAVContentManager.WEBDAV_PROPERTY_FILE,
WebDAVContentManager.WEBDAV_MAXHOSTCONNECTIONS, Integer.toString(20)));
this.webdavUserName = ContentManager.getPropertyValue(WebDAVContentManager.WEBDAV_PROPERTY_FILE, WebDAVContentManager.WEBDAV_PASSWORD, null);
this.webdavPassword = ContentManager.getPropertyValue(WebDAVContentManager.WEBDAV_PROPERTY_FILE, WebDAVContentManager.WEBDAV_USERNAME, null);
this.logger.info(WebDAVContentManager.WEBDAV_USERNAME + "=" + this.webdavUserName + " and " + WebDAVContentManager.WEBDAV_PASSWORD + " is set.");
if (this.webdavHost == null) {
throw new WebLabUncheckedException("Cannot initiate WebDAV host: host value is null");
}
if ((this.webdavUserName == null) || (this.webdavPassword == null)) {
throw new WebLabUncheckedException("Cannot initiate WebDAV host: user and/or password value is null");
}
}
private HttpClient initWebDAVClient() {
final HostConfiguration hostConfig = new HostConfiguration();
hostConfig.setHost(this.webdavHost);
final HttpConnectionManager connectionManager = new MultiThreadedHttpConnectionManager();
final HttpConnectionManagerParams params = new HttpConnectionManagerParams();
params.setMaxConnectionsPerHost(hostConfig, this.webdavMaxHostConnections);
params.setSoTimeout(this.webdavTimeOut);
connectionManager.setParams(params);
final HttpClient clnt = new HttpClient(connectionManager);
final Credentials creds = new UsernamePasswordCredentials(this.webdavUserName, this.webdavPassword);
clnt.getState().setCredentials(AuthScope.ANY, creds);
clnt.setHostConfiguration(hostConfig);
return clnt;
}
@Override
public synchronized URI writeContent(final InputStream content, final Resource resource) throws WebLabCheckedException {
this.logger.debug("Saving content on WebDAV host [" + this.webdavHost + "]");
final HttpClient client = this.initWebDAVClient();
final URI destURI;
synchronized (WebDAVContentManager.class) {
destURI = URI.create(this.webdavHost + WebDAVContentManager.PREFIX + WebDAVContentManager.cpt++ + '@' + System.currentTimeMillis()
+ WebDAVContentManager.SUFFIX);
}
this.logger.debug("Destination URI on WebDAV host [" + destURI + "]");
final PutMethod put = new PutMethod(destURI.toString());
if (resource != null) {
// getting MIME type from the resource using annotations
final DublinCoreAnnotator annotator = new DublinCoreAnnotator(resource);
final Value mime = annotator.readFormat();
// add content type to the PUT request header
if (mime!= null && mime.hasValue()){
this.logger.debug("Content mime type [" + mime.firstTypedValue() + "]");
put.setRequestHeader("content-type", mime.firstTypedValue());
}
}
put.setRequestEntity(new InputStreamRequestEntity(content));
try {
client.executeMethod(put);
put.checkSuccess();
return new URI(put.getURI().getURI());
} catch (final URISyntaxException urie) {
throw new WebLabCheckedException("Cannot create a valid from the WebDav", urie);
} catch (final HttpException httpe) {
throw new WebLabCheckedException("Cannot save content: PUT method failure on WebDAV server [" + this.webdavHost + "]: "+httpe.getMessage(), httpe);
} catch (final IOException ioe) {
throw new WebLabCheckedException("Cannot save content: I/O error while writing content on WebDAV server [" + this.webdavHost + "]: "+ioe.getMessage(), ioe);
} catch (final DavException de) {
throw new WebLabCheckedException("WebDAV error while saving on [" + destURI + "]: "+de.getMessage(), de);
} finally {
IOUtils.closeQuietly(content);
}
}
@Override
public URI writeExposedContent(final InputStream content, final Resource resource) throws WebLabCheckedException {
throw new WebLabNotYetImplementedException("writeExposedContent method not yet implemented...");
// TODO Auto-generated method stub
}
@Override
public synchronized File readContent(final URI destUri) throws WebLabCheckedException {
if (!destUri.toString().contains(this.webdavHost)) {
throw new WebLabCheckedException("Cannot read content [" + destUri + "]. The host is unknown.");
}
this.logger.debug("Getting content [" + destUri + "] from WebDAV.");
final HttpClient client = this.initWebDAVClient();
final File out;
try {
out = File.createTempFile("webDAV", ".content", this.tmpFolder);
} catch (final IOException ioe) {
throw new WebLabCheckedException("Unable to create temp file in " + this.tmpFolder + " for " + destUri, ioe);
}
final GetMethod get = new GetMethod(destUri.toString());
try {
client.executeMethod(get);
if (get.getStatusCode() != 200) {
throw new WebLabCheckedException("Cannot read content: GET method failure for content [" + destUri + "] : " + get.getStatusCode() + " '"
+ get.getStatusText() + "'");
}
final InputStream inStream = get.getResponseBodyAsStream();
try {
FileUtils.copyInputStreamToFile(inStream, out);
} finally {
IOUtils.closeQuietly(inStream);
}
} catch (final HttpException e) {
throw new WebLabCheckedException(
"Cannot read content: GET method failure for content [" + destUri + "] on WebDAV server [" + this.webdavHost + "]", e);
} catch (final IOException e) {
throw new WebLabCheckedException("Cannot read content: I/O error while copying [" + destUri + "] from WebDAV server [" + this.webdavHost
+ "] to local File [" + out + "].", e);
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy