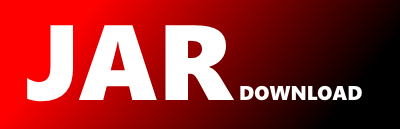
org.ow2.weblab.components.client.WebLabClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weblab-client Show documentation
Show all versions of weblab-client Show documentation
This library aims to provide a central access point to the services exposed on the ESB
without forcing each part of the system to know the actual location of the ESB and the names
of the exposed endpoints.
For this sake it allows to map service URI (ie referring to service in webLab taxonomy)
to the actual exposed URL on the ESB within the context of a specific project. The library
and the configuration should be added in portal lib in order to be central and available to
any portlets.
The mapping is done in a simple spring config file "webLabClient.xml" which must be accessible
in JAVA classpath.
The newest version!
/**
* WEBLAB: Service oriented integration platform for media mining and intelligence applications
*
* Copyright (C) 2004 - 2011 CASSIDIAN an EADS Company
*
* This library is free software; you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License along with this
* library; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth
* Floor, Boston, MA 02110-1301 USA
*/
package org.ow2.weblab.components.client;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import javax.xml.namespace.QName;
import javax.xml.ws.BindingProvider;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.ow2.weblab.components.client.config.ServiceConfigMap;
import org.ow2.weblab.components.client.config.loader.ConfigLoader;
import org.ow2.weblab.core.extended.exception.WebLabCheckedException;
import org.ow2.weblab.core.extended.exception.WebLabUncheckedException;
import org.ow2.weblab.core.model.Resource;
import org.ow2.weblab.core.services.Analyser;
import org.ow2.weblab.core.services.Analyser_Service;
import org.ow2.weblab.core.services.Configurable;
import org.ow2.weblab.core.services.Configurable_Service;
import org.ow2.weblab.core.services.Indexer;
import org.ow2.weblab.core.services.Indexer_Service;
import org.ow2.weblab.core.services.QueueManager;
import org.ow2.weblab.core.services.QueueManager_Service;
import org.ow2.weblab.core.services.ReportProvider;
import org.ow2.weblab.core.services.ReportProvider_Service;
import org.ow2.weblab.core.services.ResourceContainer;
import org.ow2.weblab.core.services.ResourceContainer_Service;
import org.ow2.weblab.core.services.Searcher;
import org.ow2.weblab.core.services.Searcher_Service;
import org.ow2.weblab.core.services.SourceReader;
import org.ow2.weblab.core.services.SourceReader_Service;
import org.ow2.weblab.core.services.Trainable;
import org.ow2.weblab.core.services.Trainable_Service;
/**
* This class implements the proposal made here :
* http://weblab-project.org/index
* .php?title=WebLab_user_interfaces/Service_Access_From_WebLab_Portlets
*
* It objectives is to provide a unique access point to back-end services and
* avoid service location configuration on portlet level. Thus portlets can use
* this class to instanciate one generic service given a specific service URI
* that identify the service function in WebLab service taxonomy.
*
* It is assumed that service URLs follow the simple syntax rules : / For instance, for a Searcher :
* http://weblab3:8181/lire-engine/searcher For a Configurable service :
* http://weblab3:8181/open-search-connector/configurable Only the last part,
* "/searcher" or "/configurable", is important.
*
* Note: userURI and usageContext are passed as argument but are not used so
* far. Later this class may implement a common security level to alter portlets
* access to services based on user and context. thus these cannot be null.
*
* @author gdupont - WebLab team - CASSIDIAN, an EADS company
* @date 2011
*/
public class WebLabClient {
private static final Log logger = LogFactory.getLog(WebLabClient.class);
private static final String WEBLAB_WSDL_FILENAME = "services/WebLab.wsdl";
private static URL webLabWSDL;
private static ServiceConfigMap config;
private static Map uri2Service;
private static void checkAndinit() {
if (webLabWSDL == null) {
// load path to WebLab WSDL
ClassLoader cl = Resource.class.getClassLoader();
if (cl == null) {
throw new WebLabUncheckedException("Cannot instanciate class loader from WebLabClient.");
}
webLabWSDL = cl.getResource(WEBLAB_WSDL_FILENAME);
if (webLabWSDL == null) {
throw new WebLabUncheckedException("Cannot find the Weblab WSDL : [" + WEBLAB_WSDL_FILENAME + "].");
}
}
if (uri2Service == null) {
uri2Service = new HashMap();
// load service URI/URL config
ConfigLoader instance = ConfigLoader.getInstance();
if (instance == null) {
throw new WebLabUncheckedException("Cannot instanciate ConfigLoader from WebLabClient.");
}
config = instance.loadConfig();
// instanciate weblab service
try {
for (Entry entry : config.entrySet()) {
addService(entry.getKey(), entry.getValue());
}
} catch (WebLabCheckedException e) {
throw new WebLabUncheckedException("WzebLab client configuration failed. " + e.getMessage(), e);
}
}
logger.debug("WebLab client ready.");
}
/**
* Get the map that links service String to URL and thus real location.
* Watch out, this is only for the bad guys since this could mess up with
* the whole configuration
*
* @return the map of String to URL as String (that's just simpler than
* messing with java String and URL)
*/
protected static Map getServiceurURLMap() {
checkAndinit();
return config;
}
/**
* Check that param are not null or throw an Exception.
*
* @param userURI
* @param usageContext
* @param serviceURI
* @throws WebLabCheckedException
*/
private static void checkParam(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkAndinit();
if (userURI == null) {
throw new WebLabCheckedException("User URI cannot be null.");
}
if (usageContext == null) {
throw new WebLabCheckedException("Usage context cannot be null.");
}
if (serviceURI == null) {
throw new WebLabCheckedException("Service URI cannot be null.");
}
}
/**
* Set the URL of a web service client.
*
* @param port
* @param newAddress
* @throws WebLabCheckedException
*/
private static void setEndpointAddress(final Object port, final String newAddress) throws WebLabCheckedException {
if (!(port instanceof BindingProvider)) {
throw new WebLabCheckedException("Object: " + port + " doesn't appear to be a valid port. May be a webservice frfamewxork conflict.");
}
final Map context = ((BindingProvider) port).getRequestContext();
context.put(BindingProvider.ENDPOINT_ADDRESS_PROPERTY, newAddress);
}
/**
* Get service local Name from its QName and set the first letter to lowar
* case.
*
* @param serviceQname
* @return
*/
private static String getServiceLocalName(QName serviceQname) {
String localName = serviceQname.getLocalPart();
String first = localName.substring(0, 1).toLowerCase();
return first + localName.substring(1);
}
/**
* Create the WebLabService object from an URI and the URL.
*
* @param serviceURI
* @return
* @throws WebLabCheckedException
*/
private static WebLabService getService(String serviceURI) throws WebLabCheckedException {
if (!config.containsKey(serviceURI.toString()) || !uri2Service.containsKey(serviceURI.toString())) {
throw new WebLabCheckedException("The service [" + serviceURI + "] is not defined in WebLab client configuration.");
}
logger.debug("Getting service [" + serviceURI + "].");
WebLabService service = uri2Service.get(serviceURI.toString());
return service;
}
/**
* Get the location of WebLab WSDL
*
* @return the URL of the WSDL
*/
public static URL getWeblabwsdl() {
checkAndinit();
return webLabWSDL;
}
/**
* Add a service in the WebLab client configuration
*
* @param uri
* @param urlString
* @throws WebLabCheckedException
* is the URL of the new service is invalid.
*/
public static void addService(String uri, String urlString) throws WebLabCheckedException {
checkAndinit();
try {
if (!urlString.endsWith("/")) {
urlString += "/";
}
// check URL
new URL(urlString);
} catch (MalformedURLException e) {
throw new WebLabCheckedException("URL " + uri + " is invalid: " + e.getMessage(), e);
}
uri2Service.put(uri, new WebLabService(uri, urlString));
logger.info("Adding service [" + uri + "].");
}
public static Analyser getAnalyser(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(Analyser_Service.SERVICE) == null) {
Analyser client = new Analyser_Service(webLabWSDL).getAnalyserPort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(Analyser_Service.SERVICE));
service.addClient(Analyser_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] Analyser interface client @" + service.getBaseUrl() + getServiceLocalName(Analyser_Service.SERVICE));
return (Analyser) service.getClient(Analyser_Service.SERVICE);
}
public static Configurable getConfigurable(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(Configurable_Service.SERVICE) == null) {
Configurable client = new Configurable_Service(webLabWSDL).getConfigurablePort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(Configurable_Service.SERVICE));
service.addClient(Configurable_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] Configurable interface client @" + service.getBaseUrl()
+ getServiceLocalName(Configurable_Service.SERVICE));
return (Configurable) service.getClient(Configurable_Service.SERVICE);
}
public static Indexer getIndexer(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(Indexer_Service.SERVICE) == null) {
Indexer client = new Indexer_Service(webLabWSDL).getIndexerPort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(Indexer_Service.SERVICE));
service.addClient(Indexer_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] Indexer interface client @" + service.getBaseUrl() + getServiceLocalName(Indexer_Service.SERVICE));
return (Indexer) service.getClient(Indexer_Service.SERVICE);
}
public static QueueManager getQueueManager(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(QueueManager_Service.SERVICE) == null) {
QueueManager client = new QueueManager_Service(webLabWSDL).getQueueManagerPort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(QueueManager_Service.SERVICE));
service.addClient(QueueManager_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] QueueManager interface client @" + service.getBaseUrl()
+ getServiceLocalName(QueueManager_Service.SERVICE));
return (QueueManager) service.getClient(QueueManager_Service.SERVICE);
}
public static ReportProvider getReportProvider(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(ReportProvider_Service.SERVICE) == null) {
ReportProvider client = new ReportProvider_Service(webLabWSDL).getReportProviderPort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(ReportProvider_Service.SERVICE));
service.addClient(ReportProvider_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] QueueManager interface client @" + service.getBaseUrl()
+ getServiceLocalName(QueueManager_Service.SERVICE));
return (ReportProvider) service.getClient(ReportProvider_Service.SERVICE);
}
public static ResourceContainer getResourceContainer(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(ResourceContainer_Service.SERVICE) == null) {
ResourceContainer client = new ResourceContainer_Service(webLabWSDL).getResourceContainerPort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(ResourceContainer_Service.SERVICE));
service.addClient(ResourceContainer_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] ResourceContainer interface client @" + service.getBaseUrl()
+ getServiceLocalName(ResourceContainer_Service.SERVICE));
return (ResourceContainer) service.getClient(ReportProvider_Service.SERVICE);
}
public static Searcher getSearcher(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(Searcher_Service.SERVICE) == null) {
Searcher client = new Searcher_Service(webLabWSDL).getSearcherPort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(Searcher_Service.SERVICE));
service.addClient(Searcher_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] Searcher interface client @" + service.getBaseUrl() + getServiceLocalName(Searcher_Service.SERVICE));
return (Searcher) service.getClient(Searcher_Service.SERVICE);
}
public static SourceReader getSourceReader(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(SourceReader_Service.SERVICE) == null) {
SourceReader client = new SourceReader_Service(webLabWSDL).getSourceReaderPort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(SourceReader_Service.SERVICE));
service.addClient(SourceReader_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] SourceReader interface client @" + service.getBaseUrl()
+ getServiceLocalName(SourceReader_Service.SERVICE));
return (SourceReader) service.getClient(SourceReader_Service.SERVICE);
}
public static Trainable getTrainable(String userURI, String usageContext, String serviceURI) throws WebLabCheckedException {
checkParam(userURI, usageContext, serviceURI);
WebLabService service = getService(serviceURI);
if (service.getClient(Trainable_Service.SERVICE) == null) {
Trainable client = new Trainable_Service(webLabWSDL).getTrainablePort();
setEndpointAddress(client, service.getBaseUrl() + getServiceLocalName(Trainable_Service.SERVICE));
service.addClient(Trainable_Service.SERVICE, client);
}
logger.debug("Getting service [" + serviceURI + "] Trainable interface client @" + service.getBaseUrl()
+ getServiceLocalName(Trainable_Service.SERVICE));
return (Trainable) service.getClient(Trainable_Service.SERVICE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy