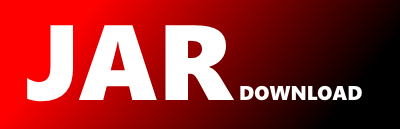
org.ow2.weblab.service.gate.GateConfiguratorService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gate-extraction Show documentation
Show all versions of gate-extraction Show documentation
Gate based component, that can process the Text units to extract informations using Gate's tools (such as grammars, gazetteers, tokenizer or POS Taggers).
This project contains two versions, a simple component and webservice one.
/**
* WEBLAB: Service oriented integration platform for media mining and intelligence applications
*
* Copyright (C) 2004 - 2009 EADS DEFENCE AND SECURITY SYSTEMS
*
* This library is free software; you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License along with this
* library; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth
* Floor, Boston, MA 02110-1301 USA
*/
package org.ow2.weblab.service.gate;
import java.io.File;
import java.util.List;
import javax.jws.WebService;
import org.apache.commons.logging.LogFactory;
import org.weblab_project.core.helper.PoKHelper;
import org.weblab_project.core.helper.RDFHelperFactory;
import org.weblab_project.core.ontologies.WebLab;
import org.weblab_project.services.configurable.Configurable;
import org.weblab_project.services.configurable.ConfigureException;
import org.weblab_project.services.configurable.ResetConfigurationException;
import org.weblab_project.services.configurable.types.ConfigureArgs;
import org.weblab_project.services.configurable.types.ConfigureReturn;
import org.weblab_project.services.configurable.types.ResetConfigurationArgs;
import org.weblab_project.services.configurable.types.ResetConfigurationReturn;
import org.weblab_project.services.exception.WebLabException;
@WebService(endpointInterface = "org.weblab_project.services.configurable.Configurable")
public class GateConfiguratorService implements Configurable {
public static String DEFAULT_SERVICE_URI = "http://weblab-project.org/webservices/gateservice";
public static final String GAPP_FILE_PATH_PROPERTY = WebLab.PROCESSING_PROPERTY_NAMESPACE + "gate" + "/gappFilePath";
private String serviceURI;
/**
* Default constructor, using DEFAULT_SERVICE_URI as serviceURI
*/
public GateConfiguratorService() {
this(DEFAULT_SERVICE_URI);
}
/**
* @param serviceURI
* The serviceURI to be set
*/
public GateConfiguratorService(final String serviceURI) {
super();
this.serviceURI = serviceURI;
}
@Override
public ConfigureReturn configure(ConfigureArgs args) throws ConfigureException {
LogFactory.getLog(this.getClass()).info("Configure method called.");
final PoKHelper helper = this.checkArgs(args);
final List gappFilePaths = helper.getLitsOnPredSubj(this.serviceURI, GAPP_FILE_PATH_PROPERTY);
if (gappFilePaths.isEmpty()) {
throw new ConfigureException("PieceOfKnowledge of used for configuration does not contains required statement: (S,P,O) = ('" + this.serviceURI + "', '" + GAPP_FILE_PATH_PROPERTY
+ "', PathToGappFile)", this.createInvalidParameterWLE());
}
if (gappFilePaths.size() > 1) {
LogFactory.getLog(this.getClass()).warn("More than one statement (S,P,O) = ('" + this.serviceURI + "', '" + GAPP_FILE_PATH_PROPERTY + "', PathToGappFile) found in configuration pok.");
}
final String gappFilePath = gappFilePaths.get(0);
this.checkFile(gappFilePath);
Configuration.getInstance().setGateApplicationStateFileName(args.getUsageContext().getUri(), gappFilePath);
LogFactory.getLog(this.getClass()).info("GappFile affected : " + gappFilePath);
return new ConfigureReturn();
}
private void checkFile(final String gappFilePath) throws ConfigureException {
File file = new File(gappFilePath);
if (!file.exists()) {
throw new ConfigureException("GappFile '" + file.getAbsolutePath() + "' does not exists.", this.createInsufficientResourcesWLE());
}
if (!file.isFile()) {
throw new ConfigureException("GappFile '" + file.getAbsolutePath() + "' is not a file.", this.createInsufficientResourcesWLE());
}
if (!file.canRead()) {
throw new ConfigureException("GappFile '" + file.getAbsolutePath() + "' cannot be read.", this.createInsufficientResourcesWLE());
}
}
private PoKHelper checkArgs(ConfigureArgs args) throws ConfigureException {
if (args == null) {
throw new ConfigureException("ConfigureArgs was null", this.createInvalidParameterWLE());
}
if (args.getUsageContext() == null) {
throw new ConfigureException("UsageContext was null", this.createInvalidParameterWLE());
}
if (args.getConfiguration() == null) {
throw new ConfigureException("Configuration was null", this.createInvalidParameterWLE());
}
if (args.getUsageContext().getUri() == null) {
throw new ConfigureException("URI of UsageContext was null", this.createInvalidParameterWLE());
}
return RDFHelperFactory.getPoKHelper(args.getConfiguration());
}
private WebLabException createInvalidParameterWLE() {
WebLabException wle = new WebLabException();
wle.setErrorId("E1");
wle.setErrorMessage("Invalid parameter");
return wle;
}
private WebLabException createInsufficientResourcesWLE() {
WebLabException wle = new WebLabException();
wle.setErrorId("E2");
wle.setErrorMessage("Insufficient resources");
return wle;
}
@Override
public ResetConfigurationReturn resetConfiguration(ResetConfigurationArgs args) throws ResetConfigurationException {
LogFactory.getLog(this.getClass()).info("ResetConfiguration method called.");
if (args == null || args.getUsageContext() == null || args.getUsageContext().getUri() == null) {
WebLabException wle = new WebLabException();
wle.setErrorId("E1");
wle.setErrorMessage("Invalid parameter");
throw new ResetConfigurationException("ResetConfigurationArgs was invalid (either it self, usageContext, it's uri or configuration was null", wle);
}
Configuration.getInstance().resetConfiguration(args.getUsageContext().getUri());
return new ResetConfigurationReturn();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy