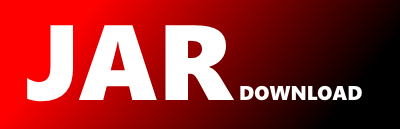
org.ow2.weblab.content.FolderContentManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of content-manager Show documentation
Show all versions of content-manager Show documentation
Use this component to manage native contents.
DO NOT USE IT FOR TEXT UNDER WINDOWS
The newest version!
/**
* WEBLAB: Service oriented integration platform for media mining and intelligence applications
*
* Copyright (C) 2004 - 2009 EADS DEFENCE AND SECURITY SYSTEMS
*
* This library is free software; you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License along with this
* library; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth
* Floor, Boston, MA 02110-1301 USA
*/
package org.ow2.weblab.content;
import java.io.File;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.logging.LogFactory;
import org.weblab_project.core.exception.WebLabCheckedException;
import org.weblab_project.core.exception.WebLabUncheckedException;
import org.weblab_project.core.helper.RDFHelperFactory;
import org.weblab_project.core.helper.ResourceHelper;
import org.weblab_project.core.model.Resource;
import org.weblab_project.core.model.content.Content;
import org.weblab_project.core.ontologies.WebLab;
import org.weblab_project.core.properties.PropertiesLoader;
import org.weblab_project.core.uri.WebLabRI;
/**
*/
public abstract class FolderContentManager {
protected String propertyKey = "folderpath";
protected String propertyFileName = "content.properties";
protected int bufferSize = 10000;
protected File folder;
/**
* The implementation of {@link ResourceHelper} to be used.
*/
public static final String SIMPLE_RESOURCE_RDF_HELPER = "org.weblab_project.core.helper.impl.JenaSingleResourceHelper";
/**
* Constructor
*
* @param folderPath
* The path to the content folder.
* @throws WebLabUncheckedException
*/
protected FolderContentManager(final String folderPath)
throws WebLabUncheckedException {
super();
this.folder = new File(folderPath);
if (!FolderContentManager.checkFolder(this.folder)) {
throw new WebLabUncheckedException("Folder '"
+ this.folder.getAbsolutePath()
+ "' is not a valid folder path");
}
LogFactory.getLog(this.getClass()).debug(
"Content provider uses folder : "
+ this.folder.getAbsolutePath());
}
/**
* Checks if a folder
is right configured.
*
* @param folder
* The file to check existence.
* @return false if folder
is not configured.
*/
protected static boolean checkFolder(final File folder) {
if (folder.exists()) {
if (!folder.isDirectory()) {
return false;
}
return true;
}
return folder.mkdirs();
}
/**
* Uses to get a file from a WebLabRI from a content manager. Manage weblab
* and file schemes.
*
* @param uri
* a valid WLRI.
* @return the file corresponding to the WLRI.
*/
protected File getFileFromURI(final WebLabRI uri) {
File newFile = null;
/*
* file scheme
*/
if (uri.toString().startsWith("file")) {
URI realUri = null;
try {
realUri = new URI(uri.toString());
} catch (URISyntaxException e) {
throw new RuntimeException(
"GRAVE, WebLabURI must be a valid URI");
}
newFile = new File(realUri);
LogFactory.getLog(this.getClass()).debug(
"Get file from FS : " + newFile.getAbsolutePath());
/*
* weblab scheme
*/
} else {
LogFactory.getLog(this.getClass()).debug(
"File path : " + this.folder.getAbsolutePath() + "/"
+ uri.toString().hashCode());
newFile = new File(this.folder.getAbsolutePath() + "/"
+ uri.toString().hashCode());
}
if (!newFile.getParentFile().exists()
&& !newFile.getParentFile().mkdirs()) {
LogFactory.getLog(this.getClass()).warn(
"Unable to create file. It may throw exception later.");
}
return newFile;
}
/**
* Returns the native file corresponding to the WLRI.
*
* @param uri
* WLRI which identify the native file.
* @return a native file.
*/
public File getFileFromWLRi(final String uri) {
return this.getFileFromURI(new WebLabRI(uri));
}
/**
* Extract the {@link WebLab#HAS_NATIVE_CONTENT} object of res
* to get the file in the FolderContentManager
.
*
* @param res
* The resource to extract native content.
* @return The file representing the native content of the resource.
* @throws WebLabCheckedException
*/
public File getNativeFileFromResource(final Resource res)
throws WebLabCheckedException {
return this.getFileFromResourceAndPredicate(res,
WebLab.HAS_NATIVE_CONTENT);
}
/**
* Extract the {@link WebLab#HAS_NORMALISED_CONTENT} object of
* res
to get the file in the FolderContentManager
* .
*
* @param res
* The resource to extract normalised content.
* @return The file representing the normalised content of the resource.
* @throws WebLabCheckedException
*/
public File getNormalisedFileFromResource(final Resource res)
throws WebLabCheckedException {
return this.getFileFromResourceAndPredicate(res,
WebLab.HAS_NORMALISED_CONTENT);
}
/**
* Extract the pred
object of res
to get the file
* in the FolderContentManager
.
*
* @param res
* The resource to extract content.
* @param pred
* The predicate used to find content URI in res annotations.
* @return The file representing the content of the resource.
* @throws WebLabCheckedException
*/
public File getFileFromResourceAndPredicate(final Resource res,
final String pred) throws WebLabCheckedException {
ResourceHelper rh = RDFHelperFactory.getSpecificResourceHelper(res,
FolderContentManager.SIMPLE_RESOURCE_RDF_HELPER);
List obj = rh.getRessOnPredSubj(res.getUri(), pred);
File file;
if (obj.size() <= 0) {
throw new WebLabCheckedException("No statement having '" + pred
+ "' as predicate found on resource '" + res.getUri()
+ "'.");
} else if (obj.size() == 1) {
try {
file = this.getFileFromWLRi(obj.get(0));
} catch (final Exception e) {
throw new WebLabCheckedException(
"Unable to retrieve file from predicate '" + pred
+ "' and resource '" + res.getUri() + "'.", e);
}
} else {
try {
file = this.getFileFromWLRi(obj.get(0));
} catch (final Exception e) {
throw new WebLabCheckedException("Unable to retrieve file "
+ "from predicate '" + pred + "' and resource '"
+ res.getUri() + "'.", e);
}
LogFactory.getLog(this.getClass()).warn(
"Two statements having '" + pred + "' as predicate "
+ "found on resource '" + res.getUri()
+ "'. The fist one was used.");
LogFactory.getLog(this.getClass()).debug(obj);
}
if (!file.exists() || !file.isFile() || !file.canRead()) {
throw new WebLabCheckedException("Unable to retrieve file "
+ "from predicate '" + pred + "' and resource '"
+ res.getUri() + "'; File '" + file.getPath()
+ "' does not exist, is not a file or is not accessible.");
}
return file;
}
/**
* Uses this to automatically get a folder path. Load it from a property
* file.
*
* @param propertyPath
* path to the property file.
* @param propertyValue
* name of the key in the property file.
* @param defaultValue
* value returned if unable to get one.
* @return the path to the file repository folder.
*/
protected static String getFolderValue(final String propertyPath,
final String propertyValue, final String defaultValue) {
final String path;
Set props = new HashSet();
props.add(propertyValue);
Map map;
try {
map = PropertiesLoader.loadProperties(propertyPath, props);
} catch (WebLabUncheckedException wlue) {
map = new HashMap(0);
}
if (map.containsKey(propertyValue)) {
path = map.get(propertyValue);
} else {
LogFactory.getLog(FolderContentManager.class).warn(
"Unable to load '" + propertyValue + "' from file '"
+ propertyPath + "'.");
path = defaultValue;
}
LogFactory.getLog(FolderContentManager.class).debug(
"Loaded : '" + path + "' as content folder.");
return path;
}
/**
* Return a Content using the contentUri, an offset and a limit.
*
* @param contentUri
* a valid WLRI which identify a Content in this "repository".
* @param offset
* beginning part of the data.
* @param limit
* maximum size of the data.
* @return a Content object containing data from offset to limit.
* @throws WebLabCheckedException
* most of time an access problem.
*/
public abstract Content getContent(final String contentUri,
final int offset, final int limit) throws WebLabCheckedException;
/**
* Save the content.
*
* @param content
* The content to be saved.
* @throws WebLabCheckedException
* most of time an access problem.
*/
public abstract void saveContent(final Content content)
throws WebLabCheckedException;
/**
* @return The folder.
*/
public File getFolder() {
return this.folder;
}
/**
* @param folder
* The folder to set.
*/
public void setFolder(final File folder) {
this.folder = folder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy