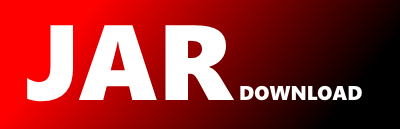
org.ow2.weblab.content.binary.BinaryFolderContentManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of content-manager Show documentation
Show all versions of content-manager Show documentation
Use this component to manage native contents.
DO NOT USE IT FOR TEXT UNDER WINDOWS
The newest version!
/**
* WEBLAB: Service oriented integration platform for media mining and intelligence applications
*
* Copyright (C) 2004 - 2009 EADS DEFENCE AND SECURITY SYSTEMS
*
* This library is free software; you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License along with this
* library; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth
* Floor, Boston, MA 02110-1301 USA
*/
package org.ow2.weblab.content.binary;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.logging.LogFactory;
import org.ow2.weblab.content.FolderContentManager;
import org.weblab_project.core.exception.WebLabCheckedException;
import org.weblab_project.core.exception.WebLabUncheckedException;
import org.weblab_project.core.model.content.BinaryContent;
import org.weblab_project.core.model.content.Content;
import org.weblab_project.core.uri.WebLabRI;
/**
*/
public class BinaryFolderContentManager extends FolderContentManager {
static Map map = new HashMap();
protected BinaryFolderContentManager(final String folderPath) throws WebLabUncheckedException {
super(folderPath);
}
/**
* Return a Content using the contentUri, an offset and a limit
*
* @param contentUri
* a valid WLRI which identify a Content in this "repository"
* @param offset
* beginning part of the file
* @param limit
* maximum size of the part
* @return a Content object containing with data writes
* @throws WebLabCheckedException
* most of time an access problem
*/
@Override
public BinaryContent getContent(final String contentUri, final int offset, final int limit) throws WebLabCheckedException {
WebLabRI wlRi = new WebLabRI(contentUri);
BinaryContent ret = new BinaryContent();
ret.setUri(contentUri);
File file = this.getFileFromURI(wlRi);
/*
* if limit = 0, read all the file
*/
int effectiveLimit = limit;
if (effectiveLimit == 0) {
effectiveLimit = (int) file.length();
}
FileInputStream fs = null;
byte[] tab = new byte[effectiveLimit];
try {
fs = new FileInputStream(file);
if (offset == fs.skip(offset)) {
ret.setLimit(fs.read(tab));
} else {
throw new WebLabCheckedException("Unable to skip " + "the right number of bytes.");
}
} catch (final IOException ioe) {
throw new WebLabCheckedException("Unable to access file.", ioe);
} finally {
if (fs != null) {
try {
fs.close();
} catch (final IOException ioe) {
LogFactory.getLog(this.getClass()).warn("Error when closing stream.", ioe);
}
}
}
ret.setUri(ret.getUri());
ret.setData(tab);
ret.setOffset(offset);
return ret;
}
/**
* @param file
* Input file
* @param newFile
* Output file
* @throws WebLabCheckedException
* If an IOException occurs.
*/
protected void copyFile(final File file, final File newFile) throws WebLabCheckedException {
byte[] tab = new byte[this.bufferSize];
try {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream(file));
try {
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(newFile, true));
try {
int readed = bis.read(tab);
while (readed != -1) {
bos.write(tab, 0, readed);
readed = bis.read(tab);
}
} finally {
try {
bos.close();
} catch (final IOException ioe) {
LogFactory.getLog(this.getClass()).warn("Unable to close stream.", ioe);
}
}
} finally {
try {
bis.close();
} catch (final IOException ioe) {
LogFactory.getLog(this.getClass()).warn("Unable to close stream.", ioe);
}
}
} catch (final IOException ioe) {
throw new WebLabCheckedException("Unable to copy file.", ioe);
}
}
/**
* Add new file to the content manager (copy it to the internal repository)
*
* @param file
* file to copy
* @param uri
* identify the future content
* @throws WebLabCheckedException
*/
public void saveFile(final File file, final String uri) throws WebLabCheckedException {
/*
* create the file and its folders
*/
File newFile = this.getFileFromWLRi(uri);
if (!newFile.getParentFile().exists() && !newFile.getParentFile().mkdirs()) {
LogFactory.getLog(this.getClass()).warn("Unable to create parent directory.");
}
this.copyFile(file, newFile);
}
/*
* (non-Javadoc)
*
* @see org.ow2.weblab.content.FolderContentManager#saveContent(org.weblab_project.core.model.content.Content)
*/
@Override
public void saveContent(final Content content) throws WebLabCheckedException {
if (!(content instanceof BinaryContent)) {
throw new WebLabCheckedException("Content '" + content.getUri() + "' is not an instance of BinaryContent of but a '" + content.getClass().getName() + "'.");
}
BinaryContent binaryContent = (BinaryContent) content;
WebLabRI wlRi = new WebLabRI(binaryContent.getUri());
File file = this.getFileFromURI(wlRi);
if (!file.getParentFile().exists() && !file.getParentFile().mkdirs()) {
LogFactory.getLog(this.getClass()).warn("Unable to create parent directory.");
}
try {
RandomAccessFile raf = new RandomAccessFile(file, "rw");
try {
raf.seek(binaryContent.getOffset());
/*
* if limit = 0, write all the data
*/
if (binaryContent.getLimit() == 0) {
binaryContent.setLimit(binaryContent.getData().length);
}
raf.write(binaryContent.getData(), 0, binaryContent.getLimit());
} finally {
try {
raf.close();
} catch (final IOException ioe) {
throw new WebLabCheckedException("Unable to close stream.", ioe);
}
}
} catch (final IOException ioe) {
throw new WebLabCheckedException("A I/O exception occurs.", ioe);
}
}
/**
* @param folderPath
* The path to the folder.
* @return The BinaryFolderContentManager
* @throws WebLabUncheckedException
*/
public static BinaryFolderContentManager getInstance(String folderPath) throws WebLabUncheckedException {
BinaryFolderContentManager binaryFolderContentManager = BinaryFolderContentManager.map.get(folderPath);
if (binaryFolderContentManager == null) {
binaryFolderContentManager = new BinaryFolderContentManager(folderPath);
BinaryFolderContentManager.map.put(folderPath, binaryFolderContentManager);
}
return binaryFolderContentManager;
}
/**
* @return The BinaryFolderContentManager
* @throws WebLabUncheckedException
*/
public static BinaryFolderContentManager getInstance() throws WebLabUncheckedException {
return BinaryFolderContentManager.getInstance(FolderContentManager.getFolderValue("content.properties", "binarycontentpath", "binarycontent"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy