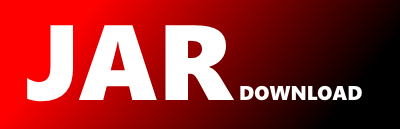
org.ow2.weblab.content.text.TextFolderContentManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of content-manager Show documentation
Show all versions of content-manager Show documentation
Use this component to manage native contents.
DO NOT USE IT FOR TEXT UNDER WINDOWS
The newest version!
/**
* WEBLAB: Service oriented integration platform for media mining and intelligence applications
*
* Copyright (C) 2004 - 2009 EADS DEFENCE AND SECURITY SYSTEMS
*
* This library is free software; you can redistribute it and/or modify it under the terms of
* the GNU Lesser General Public License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License along with this
* library; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth
* Floor, Boston, MA 02110-1301 USA
*/
package org.ow2.weblab.content.text;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.logging.LogFactory;
import org.ow2.weblab.content.FolderContentManager;
import org.weblab_project.core.exception.WebLabCheckedException;
import org.weblab_project.core.exception.WebLabUncheckedException;
import org.weblab_project.core.model.content.Content;
import org.weblab_project.core.model.content.TextContent;
/**
*/
public class TextFolderContentManager extends FolderContentManager {
static Map map = new HashMap();
static final String CHARSET = "UTF-8";
protected TextFolderContentManager(final String folder) throws WebLabUncheckedException {
super(folder);
}
/**
* Return a Content using the contentUri, an offset and a limit
*
* @param contentUri
* a valid WLRI which identify a Content in this "repository"
* @param offset
* beginning part of the text
* @param limit
* maximum size of the text
* @return a Content object containing with the good text part
* @throws WebLabCheckedException
* most of time an access problem
*/
@Override
public TextContent getContent(final String contentUri, final int offset, final int limit) throws WebLabCheckedException {
TextContent ret = new TextContent();
ret.setUri(contentUri);
char[] fakeBuf = new char[offset];
char[] buf = new char[limit];
final int newLimit;
try {
File file = getFileFromWLRi(contentUri);
FileInputStream fis = new FileInputStream(file);
InputStreamReader isr = new InputStreamReader(fis, CHARSET);
try {
final int readed = isr.read(fakeBuf);
if (readed < offset) {
throw new IOException("Unable to move the offset to " + offset + " chars, only " + readed + " available.");
}
newLimit = isr.read(buf);
} finally {
try {
fis.close();
} catch (final IOException ioe) {
LogFactory.getLog(this.getClass()).warn("Unable to close reader.", ioe);
}
}
} catch (final IOException ioe) {
throw new WebLabCheckedException("Unable to get content.", ioe);
}
ret.setUri(ret.getUri());
ret.setOffset(offset);
ret.setLimit(newLimit);
ret.setData(new String(buf));
return ret;
}
/**
* Add new file to the content manager (copy it to the internal repository)
*
* @param toBeSaved
* file to copy
* @param uri
* identify the future content
* @throws WebLabCheckedException
*/
public void saveString(final String toBeSaved, final String uri) throws WebLabCheckedException {
/*
* create the file and its folders
*/
File file = this.getFileFromWLRi(uri);
if (file.exists()) {
LogFactory.getLog(this.getClass()).warn("Try to assign a string to an existing " + "file. Older file deleted.");
}
try {
FileOutputStream fos = new FileOutputStream(file);
OutputStreamWriter osw = new OutputStreamWriter(fos, CHARSET);
try {
osw.write(toBeSaved);
osw.flush();
} finally {
try {
fos.close();
} catch (final IOException ioe) {
LogFactory.getLog(this.getClass()).warn("Unable to close writer.", ioe);
}
}
} catch (final IOException ioe) {
throw new WebLabCheckedException("Unable to write content.", ioe);
}
}
/**
* Save the Content object as a part of a file.
*
* @param content
* Content to be saved
* @throws WebLabCheckedException
*/
@Override
public void saveContent(final Content content) throws WebLabCheckedException {
if (!(content instanceof TextContent)) {
throw new WebLabCheckedException("Content '" + content.getUri() + "' is not an instance of TextContent of but a '" + content.getClass().getName() + "'.");
}
TextContent textContent = (TextContent) content;
int bufSize = 1024;
File file = getFileFromWLRi(textContent.getUri());
StringBuffer buf = new StringBuffer();
char[] tab = new char[bufSize];
try {
FileInputStream fis = new FileInputStream(file);
InputStreamReader isr = new InputStreamReader(fis, CHARSET);
try {
int readed = isr.read(tab);
while (readed > 0) {
buf.append(tab, 0, readed);
readed = isr.read(tab);
}
} finally {
try {
fis.close();
} catch (final IOException ioe) {
LogFactory.getLog(this.getClass()).warn("Unable to close reader.");
}
}
} catch (final IOException ioe) {
throw new WebLabCheckedException("Unable to read the content to save.", ioe);
}
String begin = buf.substring(0, textContent.getOffset()) + textContent.getData();
final String end = buf.substring(textContent.getOffset() + textContent.getData().length());
if (end != null) {
begin += end;
}
this.saveString(begin, content.getUri());
}
/**
* @param folderPath
* The path to the folder.
* @return The TextFolderContentManager
* @throws WebLabUncheckedException
*/
public static TextFolderContentManager getInstance(final String folderPath) throws WebLabUncheckedException {
TextFolderContentManager textFolderContentManager = TextFolderContentManager.map.get(folderPath);
if (textFolderContentManager == null) {
textFolderContentManager = new TextFolderContentManager(folderPath);
TextFolderContentManager.map.put(folderPath, textFolderContentManager);
}
return textFolderContentManager;
}
/**
* @return The TextFolderContentManager
* @throws WebLabUncheckedException
*/
public static TextFolderContentManager getInstance() throws WebLabUncheckedException {
return TextFolderContentManager.getInstance(FolderContentManager.getFolderValue("content.properties", "textcontentpath", "textcontent"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy