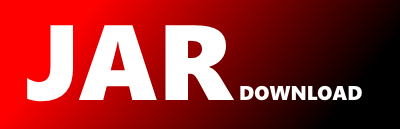
org.xlcloud.service.heat.parsers.utils.JsonObjectWrapper Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service.heat.parsers.utils;
import java.util.Collection;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.xlcloud.service.heat.parsers.utils.iterable.JsonPair;
import org.xlcloud.service.heat.template.commons.HeatTemplateValue;
import org.xlcloud.service.heat.template.fields.JsonKey;
/**
* Defines the interface of a JSON object wrapper.
*
* @author Krzysztof Szafrański, AMG.net
*/
public interface JsonObjectWrapper extends Iterable {
/**
* @return wrapped json object
*/
JSONObject toJsonObject();
/**
* Returns json object wrapped with new {@link JsonObjectWrapper}.
* If the current json object does not contain json object with the given key, then {@link JSONException} is thrown.
*
* @param key - key of the requested element
* @return wrapper for the json object
* @throws JSONException
*/
JsonObjectWrapper getWrapper(JsonKey key) throws JSONException;
JSONObject get(JsonKey key) throws JSONException;
/**
* Returns string with the given key
* If the current json object does not contain string with the given key, then {@link JSONException} is thrown.
*
* @param key - key of the requested element
* @return requested string
* @throws JSONException
*/
String getString(JsonKey key) throws JSONException;
/**
* Returns string with the given key
* If the current json object does not contain string with the given key, then empty string is returned
* @param key - key of the requested element
* @return requested string
*/
String optStringNull(String key);
/**
* Returns string with the given key
* If the current json object does not contain string with the given key, then null is returned
* @param key - key of the requested element
* @return requested string
*/
String optStringNull(JsonKey key);
/**
* Returns json array with the given key
* If the current json object does not contain json array with the given key, then {@link JSONException} is thrown.
*
* @param key - key of the requested element
* @return json array
* @throws JSONException
*/
JSONArray getArray(JsonKey key) throws JSONException;
/**
* It gets the json array with the given key and converts to the list of strings.
* If the current json object does not contain json array with the given key, then {@link JSONException} is thrown.
*
* @param key - key of the requested element
* @return requested list of strings
* @throws JSONException
*/
List requiredArray(JsonKey key) throws JSONException;
/**
* It gets the json array with the given key and converts to the list of strings.
* If the current json object does not contain json array with the given key, then null is returned.
*
* @param key - key of the requested element
* @return requested list of strings (or null)
* @throws JSONException
*/
List optArrayNull(JsonKey key) throws JSONException;
/**
* Get an optional boolean associated with the key. It returns null if there is no such key or value is not Boolean.
* @param key
* - key to be found
* @return boolean value if exists
*/
Boolean optBooleanNull(JsonKey key);
/**
* Returns Long with the given key
* If the current json object does not contain string with the given key, then null is returned
* @param key - key of the requested element
* @return requested Long
* @throws JSONException
*/
Long optLongNull(JsonKey key) throws JSONException;
/**
* Returns Integer with the given key
* If the current json object does not contain field with the given key, then null is returned
* @param key - key of the requested element
* @return requested Integer (or null)
* @throws JSONException
*/
Integer optIntegerNull(JsonKey key) throws JSONException;
/**
* Puts the given json object with the given key to the current json object.
* If the given key is null, then {@link JSONException} is thrown.
* If the given value is null and the given key is not null and the given key already exists in the json object,
* then the given key is removed from the json object.
*
* @param key - key of the given element
* @param jsonSnippet - value to be set
* @return this wrapper
* @throws JSONException
*/
JsonObjectWrapper put(JsonKey key, JSONObject jsonSnippet) throws JSONException;
/**
* Puts the given json object with the given key to the current json object.
* If the given key is null or given value is null, then nothing happens.
*
* @param key - key of the given element
* @param jsonSnippet - value to be set
* @return this wrapper
* @throws JSONException
*/
JsonObjectWrapper putOpt(JsonKey key, JSONObject jsonSnippet) throws JSONException;
/**
* Puts the given json object with the given key to the current json object.
* If the given key is null or given value is null, then nothing happens.
*
* @param key
* @param value
* @throws JSONException
*/
JsonObjectWrapper putOpt(JsonKey key, Boolean value) throws JSONException;
/**
* Puts the given json object with the given key to the current json object.
* If the given key is null or given value is null, then nothing happens.
*
* @param key
* @param value
* @throws JSONException
*/
JsonObjectWrapper putOpt(JsonKey key, String value) throws JSONException;
/**
* Puts the Integer value with the given key to the current json object.
* If the given value is null, then nothing happens.
*
* @param key cannot be null
* @param value
* @return this wrapper instance
* @throws JSONException
*/
JsonObjectWrapper putOpt(JsonKey key, Integer value) throws JSONException;
/**
* Puts the {@link HeatTemplateValue} with the given key to the current json
* object. If the given value is null, then nothing happens.
*
* @param key
* cannot be null
* @param value
* @return this wrapper instance
* @throws JSONException
*/
JsonObjectWrapper putOptHeatTemplateValue(JsonKey key, HeatTemplateValue value) throws JSONException;
JsonObjectWrapper putHeatTemplateValue(JsonKey key, HeatTemplateValue value) throws JSONException;
/**
* Puts the given object with the given key to the current json object.
* If the given key is null, then {@link JSONException} is thrown.
* If the given value is null and the given key is not null and the given key already exists in the json object,
* then the given key is removed from the json object.
*
* @param key - key of the given element
* @param jsonSnippet - value to be set
* @return this wrapper
* @throws JSONException
*/
JsonObjectWrapper put(JsonKey key, Object jsonSnippet) throws JSONException;
/**
* Puts the given json object, with the given key to the current json object.
* If the given key is null, then {@link JSONException} is thrown.
* If the given value is null and the given key is not null and the given key already exists in the json object,
* then the given key is removed from the json object.
*
* @param key - key of the given element
* @param jsonSnippet - value to be set
* @return this wrapper
* @throws JSONException
*/
JsonObjectWrapper put(JsonKey key, JsonObjectWrapper jsonSnippet) throws JSONException;
/**
* Puts the given string with the given key to the current json object.
* If the given key is null, then {@link JSONException} is thrown.
* If the given value is null and the given key is not null and the given key already exists in the json object,
* then the given key is removed from the json object.
*
* @param key - key of the given element
* @param string - value to be set
* @return this wrapper
* @throws JSONException
*/
JsonObjectWrapper put(JsonKey key, String string) throws JSONException;
/**
* Puts the given string with the given key to the current json object.
* If the current value represents json object, then the value is casted to the {@link JSONObject}.
*
* If the given key is null, then {@link JSONException} is thrown.
* If the given value is null and the given key is not null, then nothing happens
*
* @param key - key of the given element
* @param value - value to be set
* @return this wrapper
* @throws JSONException
*/
JsonObjectWrapper putValue(JsonKey key, String value) throws JSONException;
/**
* Puts the boolean value to a current json object (under specified key)
* only if the boolean value is not null. If current json already had a
* field with same key, it'll be replaced
*
* @param key cannot be null
* @param value boolean value or null
* @return this json wrapper instance
* @throws JSONException
*/
JsonObjectWrapper putValue(JsonKey key, Boolean value) throws JSONException;
/**
* Puts the given string with the given key to the current json object.
* If the current value represents json object, then the value is casted to the {@link JSONObject}.
*
* If the given key is null, then {@link JSONException} is thrown.
* If the given value is null and the given key is not null, then empty value is set to the {@link JSONObject}
*
* @param key - key of the given element
* @param value - value to be set
* @return this wrapper
* @throws JSONException
*/
JsonObjectWrapper putValueOrEmptyString(JsonKey key, String value) throws JSONException;
/**
* Puts the given collection, with the given key to the current json object.
* If any of the element represents json object, then the value is casted to the {@link JSONObject}.
*
* If the given key is null, then {@link JSONException} is thrown.
* If the given array is null or empty, then nothing happens
*
* @param key - key of the given element
* @param value - value to be set
* @return this wrapper
* @throws JSONException
*/
JsonObjectWrapper putValue(JsonKey key, Collection array) throws JSONException;
/**
* @param key - key to be found
* @return {@code true} if the current json object contains the given key, {@code false} otherwise
*/
boolean has(JsonKey key);
/**
* @param key - key to be found
* @return {@code false} if the current json object contains the given key, {@code true} otherwise
*/
boolean hasNot(JsonKey key);
HeatTemplateValue optHeatTemplateValueNull(JsonKey key) throws JSONException;
HeatTemplateValue getHeatTemplateValue(JsonKey key) throws JSONException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy