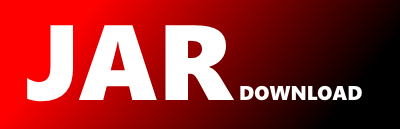
org.xlcloud.service.ApplicationDeployment Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.xlcloud.service.adapter.DateAdapter;
/**
* Class that represents application deployment model
* @author Konrad Król, AMG.net
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"runlistAttributes"
})
@XmlRootElement(name = "applicationDeployment")
public class ApplicationDeployment extends Referenceable {
/**
* TODO Documentation serialVersionUID
*/
private static final long serialVersionUID = 8442106540309413166L;
private static final String RESOURCE_PREFIX = "/deployments";
private static final String LAYERS = "layers/";
private List runlistAttributes = new ArrayList();
@XmlAttribute
private String name;
@XmlAttribute
private Long layerId;
@XmlAttribute
@XmlJavaTypeAdapter(DateAdapter.class)
private Date deployedAt;
@XmlAttribute
private ApplicationDeploymentStatus status;
/**
* Gets the value of {@link #runlistAttributes}.
* @return value of {@link #runlistAttributes}
*/
public List getRunlistAttributes() {
if(runlistAttributes == null){
runlistAttributes = new ArrayList<>();
}
return runlistAttributes;
}
/**
* Gets the value of {@link #layerId}.
* @return value of {@link #layerId}
*/
public Long getLayerId() {
return layerId;
}
/**
* Sets the value of {@link #layerId}.
* @param layerId - value
*/
public void setLayerId(Long layerId) {
this.layerId = layerId;
}
/** {@inheritDoc} */
@Override
protected String getResourcePrefix() {
return LAYERS + layerId + RESOURCE_PREFIX;
}
/**
* Gets the value of {@link #deployedAt}.
* @return value of {@link #deployedAt}
*/
public Date getDeployedAt() {
return deployedAt;
}
/**
* Sets the value of {@link #deployedAt}.
* @param deployedAt - value
*/
public void setDeployedAt(Date deployedAt) {
this.deployedAt = deployedAt;
}
/**
* Gets the value of {@link #name}.
* @return value of {@link #name}
*/
public String getName() {
return name;
}
/**
* Sets the value of {@link #name}.
* @param name - value
*/
public void setName(String name) {
this.name = name;
}
/**
* Gets the value of {@link #status}.
* @return value of {@link #status}
*/
public ApplicationDeploymentStatus getStatus() {
return status;
}
/**
* Sets the value of {@link #status}.
* @param status - value
*/
public void setStatus(ApplicationDeploymentStatus status) {
this.status = status;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy