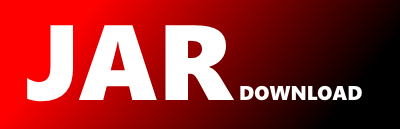
org.xlcloud.service.LayerBlueprint Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.xlcloud.service.heat.template.Template;
/**
* JAXB-annotated layer blueprint model
* @author Tomek Adamczewski, AMG.net
* @author Konrad Król, AMG.net
*/
@XmlAccessorType( XmlAccessType.FIELD )
@XmlType( name = "" )
@XmlRootElement( name = "layerBlueprint" )
public class LayerBlueprint extends LayerBase implements TemplateBasedModel {
/**
* TODO Documentation serialVersionUID
*/
private static final long serialVersionUID = -4789212356313713903L;
@XmlAttribute
private String author;
@XmlAttribute
private String license;
@XmlAttribute
private String copyright;
@XmlAttribute
private String type;
@XmlAttribute
private CatalogScopeType catalogScope;
@XmlAttribute
private Long stackBlueprintId;
public LayerBlueprint fromTemplate(Template template) {
this.template = template;
return this;
}
/** {@inheritDoc} */
@Override
public Template toTemplate() {
return this.template;
}
/**
* Gets the value of {@link #author}.
*
* @return value of {@link #author}
*/
public String getAuthor() {
return author;
}
/**
* Sets the value of {@link #author}.
*
* @param author
* - value
*/
public void setAuthor(String author) {
this.author = author;
}
/**
* Gets the value of {@link #license}.
*
* @return value of {@link #license}
*/
public String getLicense() {
return license;
}
/**
* Sets the value of {@link #license}.
*
* @param license
* - value
*/
public void setLicense(String license) {
this.license = license;
}
/**
* Gets the value of {@link #copyright}.
*
* @return value of {@link #copyright}
*/
public String getCopyright() {
return copyright;
}
/**
* Sets the value of {@link #copyright}.
*
* @param copyright
* - value
*/
public void setCopyright(String copyright) {
this.copyright = copyright;
}
/**
* Gets the value of {@link #type}.
* @return value of {@link #type}
*/
public String getType() {
return type;
}
/**
* Sets the value of {@link #type}.
* @param type - value
*/
public void setType(String type) {
this.type = type;
}
/**
* Gets the value of {@link #catalogScope}.
*
* @return value of {@link #catalogScope}
*/
public CatalogScopeType getCatalogScope() {
return catalogScope;
}
/**
* Sets the value of {@link #catalogScope}.
*
* @param catalogScope
* - value
*/
public void setCatalogScope(CatalogScopeType catalogScope) {
this.catalogScope = catalogScope;
}
@Override
protected String getResourcePrefix() {
return "/layer-blueprints";
}
/**
* Gets the value of {@link #stackBlueprintId}.
* @return value of {@link #stackBlueprintId}
*/
public Long getStackBlueprintId() {
return stackBlueprintId;
}
/**
* Sets the value of {@link #stackBlueprintId}.
* @param stackBlueprintId - value
*/
public void setStackBlueprintId(Long stackBlueprintId) {
this.stackBlueprintId = stackBlueprintId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy