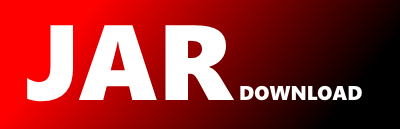
org.xlcloud.service.Repository Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Class represents repository, which can be registered by user in XMS system.
* Repository is parsed by system against cookbooks, and registered with
* {@link Cookbook} model.
*
* @author Tomek Adamczewski, AMG.net
* @author Konrad Król, AMG.net
*/
@XmlAccessorType( XmlAccessType.FIELD )
@XmlType( name = "" )
@XmlRootElement( name = "user" )
public class Repository extends Referenceable {
/**
* TODO Documentation serialVersionUID
*/
private static final long serialVersionUID = -6640668798848813734L;
private static final String RESOURCE_PREFIX = "repositories";
@XmlAttribute
private String name;
@XmlAttribute
private String uri;
@XmlAttribute
private RepositoryType type;
@XmlAttribute
private CatalogScopeType catalogScope;
@XmlAttribute
private Long accountId;
@XmlAttribute
private RepositoryStatus status;
@XmlAttribute
private String statusReason;
@XmlAttribute
private String ref;
/**
* Gets the value of {@link #name}.
*
* @return value of {@link #name}
*/
public String getName() {
return name;
}
/**
* Sets the value of {@link #name}.
*
* @param name
* - value
*/
public void setName(String name) {
this.name = name;
}
/**
* Gets the value of {@link #uri}.
*
* @return value of {@link #uri}
*/
public String getUri() {
return uri;
}
/**
* Sets the value of {@link #uri}.
*
* @param uri
* - value
*/
public void setUri(String uri) {
this.uri = uri;
}
public RepositoryType getType() {
return type;
}
public void setType(RepositoryType type) {
this.type = type;
}
/**
* Gets the value of {@link #catalogScope}.
*
* @return value of {@link #catalogScope}
*/
public CatalogScopeType getCatalogScope() {
return catalogScope;
}
/**
* Sets the value of {@link #catalogScope}.
*
* @param catalogScope
* - value
*/
public void setCatalogScope(CatalogScopeType catalogScope) {
this.catalogScope = catalogScope;
}
/**
* Gets the value of {@link #accountId}.
*
* @return value of {@link #accountId}
*/
public Long getAccountId() {
return accountId;
}
/**
* Sets the value of {@link #accountId}.
*
* @param accountId
* - value
*/
public void setAccountId(Long accountId) {
this.accountId = accountId;
}
/**
* Gets the value of {@link #status}.
*
* @return value of {@link #status}
*/
public RepositoryStatus getStatus() {
return status;
}
/**
* Sets the value of {@link #status}.
*
* @param status
* - value
*/
public void setStatus(RepositoryStatus status) {
this.status = status;
}
/**
* Gets the value of {@link #ref}.
* @return value of {@link #ref}
*/
public String getRef() {
return ref;
}
/**
* Sets the value of {@link #ref}.
* @param ref - value
*/
public void setRef(String ref) {
this.ref = ref;
}
@Override
protected String getResourcePrefix() {
return RESOURCE_PREFIX;
}
/**
* Gets the value of {@link #statusReason}.
* @return value of {@link #statusReason}
*/
public String getStatusReason() {
return statusReason;
}
/**
* Sets the value of {@link #statusReason}.
* @param statusReason - value
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy