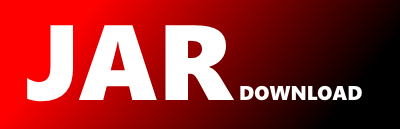
org.xlcloud.service.StackBase Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import org.xlcloud.service.heat.template.Mappings;
import org.xlcloud.service.heat.template.Outputs;
import org.xlcloud.service.heat.template.Parameter;
import org.xlcloud.service.heat.template.Parameters;
import org.xlcloud.service.heat.template.Template;
import org.xlcloud.service.heat.template.fields.ResourceType;
import org.xlcloud.service.heat.template.resources.ResourceBase;
import org.xlcloud.service.heat.template.resources.Resources;
import org.xlcloud.service.heat.template.resources.SecurityGroup;
/**
* Base model for {@link Stack} and {@link StackBlueprint}.
*
* @author Konrad Król, AMG.net
*/
@XmlAccessorType( XmlAccessType.FIELD )
@XmlSeeAlso( { StackBlueprint.class, Stack.class } )
@XmlType( name = "stackBase", propOrder = { "template" } )
public abstract class StackBase extends Parameterizable {
/**
* TODO Documentation serialVersionUID
*/
private static final long serialVersionUID = -1114839842510956815L;
private static final Set RESERVED_RESOURCE_TYPES = new HashSet(Arrays.asList(new ResourceType[] {
ResourceType.STACK, ResourceType.SECURITY_GROUP }));
@XmlAttribute
protected String name;
@XmlAttribute
protected Long accountId;
@XmlElement
protected Template template = new Template();
/**
* Gets the value of {@link #resources}.
*
* @return value of {@link #resources}
*/
public Resources getResources() {
Resources res = new Resources();
for (ResourceBase r : template.getResources().getResource()) {
if (!RESERVED_RESOURCE_TYPES.contains(r.getResourceType())) {
res.getResource().add(r);
}
}
return res;
}
public SecurityGroups getSecurityGroups() {
SecurityGroups securityGroups = new SecurityGroups();
for (ResourceBase resource : template.getResources().getResource()) {
if (ResourceType.SECURITY_GROUP.equals(resource.getResourceType())) {
securityGroups.getSecurityGroup().add((SecurityGroup) resource);
}
}
return securityGroups;
}
public void setSecurityGroups(SecurityGroups securityGroups) {
template.getResources().getResource().addAll(securityGroups.getSecurityGroup());
}
public void addSecurityGroup(SecurityGroup securityGroup) {
template.getResources().getResource().add(securityGroup);
}
public void removeSecurityGroup(SecurityGroup securityGroup) {
template.getResources().getResource().remove(securityGroup);
}
public void setResources(Resources resources) {
// this is done so because additional resources connected with layers
// are also stored
// and would be lost if setter would just override existing resources
// collection
template.getResources().getResource().addAll(resources.getResource());
}
/**
* Gets the value of {@link #description}.
*
* @return value of {@link #description}
*/
public String getDescription() {
return template.getDescription();
}
/**
* Sets the value of {@link #description}.
*
* @param description
* - value
*/
public void setDescription(String description) {
this.template.setDescription(description);
}
/**
* Gets the value of {@link #name}.
*
* @return value of {@link #name}
*/
public String getName() {
return name;
}
/**
* Sets the value of {@link #name}.
*
* @param name
* - value
*/
public void setName(String name) {
this.name = name;
}
/**
* Gets the value of {@link #parameters}.
*
* @return value of {@link #parameters}
*/
public Parameters getParameters() {
Parameters params = new Parameters();
for (Parameter p : template.getParameters().getParameter()) {
if (ReservedParameterName.isNotReservedName(p.getName())) {
params.getParameter().add(p);
}
}
return params;
}
public void setParameters(Parameters parameters) {
// this is done so because default instance type and default image id
// are stored as parameters
// and would be lost if setter would just override existing parameters
// collection
template.getParameters().getParameter().addAll(parameters.getParameter());
}
/**
* Gets the value of {@link #outputs}.
*
* @return value of {@link #outputs}
*/
public Outputs getOutputs() {
return template.getOutputs();
}
public void setOutputs(Outputs outputs) {
template.setOutputs(outputs);
}
/**
* Gets the value of {@link #mappings}.
*
* @return value of {@link #mappings}
*/
public Mappings getMappings() {
return template.getMappings();
}
public void setMappings(Mappings mappings) {
template.setMappings(mappings);
}
/**
* Gets the value of {@link #defaultInstanceType}.
*
* @return value of {@link #defaultInstanceType}
*/
public Parameter getDefaultInstanceType() {
return template.getParameters().getParameterByName(ReservedParameterName.DEFAULT_INSTANCE_TYPE_PARAM_NAME.paramName());
}
/**
* Sets the value of {@link #defaultInstanceType}.
*
* @param defaultInstanceType
* - value
*/
public void setDefaultInstanceType(Parameter defaultInstanceType) {
template.getParameters().getParameter().add(defaultInstanceType);
}
/**
* Gets the value of {@link #defaultImageId}.
*
* @return value of {@link #defaultImageId}
*/
public Parameter getDefaultImageId() {
return template.getParameters().getParameterByName(ReservedParameterName.DEFAULT_IMAGE_ID_PARAM_NAME.paramName());
}
/**
* Sets the value of {@link #defaultImageId}.
*
* @param defaultImageId
* - value
*/
public void setDefaultImageId(Parameter defaultImageId) {
template.getParameters().getParameter().add(defaultImageId);
}
/**
* Gets the value of {@link #defaultImageId}.
*
* @return value of {@link #defaultImageId}
*/
public Parameter getDefaultKeyName() {
return template.getParameters().getParameterByName(ReservedParameterName.DEFAULT_KEY_NAME_PARAM_NAME.paramName());
}
/**
* Sets the value of {@link #defaultImageId}.
*
* @param defaultImageId
* - value
*/
public void setDefaultKeyName(Parameter defaultKeyName) {
template.getParameters().getParameter().add(defaultKeyName);
}
/**
* Gets the value of {@link #accountId}.
*
* @return value of {@link #accountId}
*/
public Long getAccountId() {
return accountId;
}
/**
* Sets the value of {@link #accountId}.
*
* @param accountId
* - value
*/
public void setAccountId(Long accountId) {
this.accountId = accountId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy