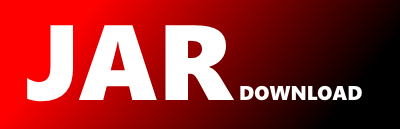
org.xlcloud.service.api.AccessTokensApi Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service.api;
import org.xlcloud.rest.exception.ObjectNotFoundException;
import org.xlcloud.service.AccessToken;
import org.xlcloud.service.Entitlement;
import org.xlcloud.service.Entitlements;
/**
* Interface for API that allows to manage {@link AccessToken} and related resources
*
* @author Tomek Adamczewski, AMG.net
* @author Konrad Król, AMG.net
*/
public interface AccessTokensApi {
/**
* Get a single access token
*
* @param tokenId
* id of the {@link AccessToken}
* @return access token with the specified id
* @throws ObjectNotFoundException if no access token exists with specified id
*/
AccessToken getAccessToken(String tokenId) throws ObjectNotFoundException;
/**
* Revoke a single access token
*
* @param tokenId
* id of the access token
* @throws ObjectNotFoundException if no access token exists with specified id
*/
void revokeAccessToken(String tokenId) throws ObjectNotFoundException;
/**
* List all entitlement assigned to an access token
*
* @param tokenId
* id of the {@link AccessToken}
* @return entitlements assigned to acceess token with the specified id
* @throws ObjectNotFoundException if no access token exists with specified id
*/
Entitlements getAccessTokenEntitlements(String tokenId) throws ObjectNotFoundException;
/**
* Creates an access token entitelment
*
* @param tokenId
* id of the {@link AccessToken}
* @param entitlement entitlement to be created for access token
* @throws ObjectNotFoundException if the object was not found
* @return created Entitlement
*/
Entitlement createAccessTokenEntitlement(String tokenId, Entitlement entitlement);
/**
* Removes a specified entitlement from a specified access token
*
* @param tokenId
* id of the {@link AccessToken}
* @param entitlementId
* @throws ObjectNotFoundException if the object was not found
*/
void removeAccessTokenEntitlement(String tokenId, Long entitlementId);
/**
* Updates entitlements for specified access token.
* Note that old entitlements are removed and replaced with a new collection.
*
* @param tokenId
* id of the {@link AccessToken}
* @param entitlements
* updated {@link Entitlements}
* @throws ObjectNotFoundException if the object was not found
* @return entitlements uddated entitlements
*/
Entitlements updateAccessTokenEntitlements(String tokenId, Entitlements entitlements);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy