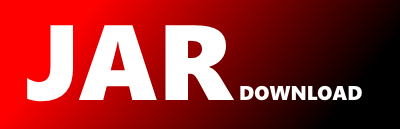
org.xlcloud.service.api.GroupsApi Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service.api;
import org.xlcloud.rest.exception.ObjectNotFoundException;
import org.xlcloud.rest.exception.ValidationException;
import org.xlcloud.service.Entitlement;
import org.xlcloud.service.Entitlements;
import org.xlcloud.service.Group;
import org.xlcloud.service.User;
import org.xlcloud.service.Users;
/**
* Interface for API that allows to manage {@link Group}'s and related resources
*
* @author Tomek Adamczewski, AMG.net
* @author Konrad Król, AMG.net
*/
public interface GroupsApi {
/**
* Returns the {@link Group} with the specified id
*
* @param groupId id of the requested {@link Group}
* @return {@link Group} with the specified id
* @throws ObjectNotFoundException if group with the specified id does not exist
*/
Group getGroup(Long groupId) throws ObjectNotFoundException;
/**
* Removes the {@link Group} with the specified id
*
* @param groupId id of the group to be removed
* @throws ObjectNotFoundException if group with the specified id does not exist
*/
void removeGroup(Long groupId) throws ObjectNotFoundException;
/**
* Creates an {@link Entitlement} for the {@link Group}
*
* @param groupId id of the requested {@link Group}
* @param entitlement {@link Entitlement} to be created
*
* @return created entitlement
*
* @throws ObjectNotFoundException if group with the specified id does not exist
* @throws ValidationException if entitlement validation fails
*/
Entitlement createGroupEntitlement(Long groupId, Entitlement entitlement) throws ObjectNotFoundException, ValidationException;
/**
* Returns a list of {@link Group} {@link Entitlements}
*
* @param groupId id of the requested group
* @return list of group entitlements wrapped into {@link Entitlements} object
* @throws ObjectNotFoundException if group with the specified id does not exist
*/
Entitlements getGroupEntitlements(Long groupId) throws ObjectNotFoundException;
/**
* Removes the {@link Group} {@link Entitlement}.
*
* @param groupId id of the requested {@link Group}
* @param entitlementId id of the {@link Entitlement} to be removed.
*
* @throws ObjectNotFoundException if group or entitlement with the specified id does not exist
*/
void removeGroupEntitlement(Long groupId, Long entitlementId) throws ObjectNotFoundException;
/**
* Returns the list of {@link Group} {@link Users}.
*
* @param groupId id of the requested {@link Group}
* @return users list wrapped into {@link Users} object
* @throws ObjectNotFoundException if group with the specified id does not exist
*/
Users getGroupUsers(Long groupId) throws ObjectNotFoundException;
/**
* Creates a new {@link User}, assigned to the requested {@link Group}.
*
* @param groupId id of the requested {@link Group}
* @param user id of the {@link User} to be created
*
* @return created user
*
* @throws ObjectNotFoundException if group or user with the specified id does not exist
* @throws ValidationException if the user validation fails
*/
User createUserInGroup(Long groupId, User user) throws ObjectNotFoundException, ValidationException;
/**
* Assign a user go the requested {@link Group}
* @param groupId id of the requested {@link Group}
* @param userId id of the {@link User} to be assigned
* @throws ObjectNotFoundException if group or user with the specified id does not exist
*/
void assignGroupUser(Long groupId, Long userId) throws ObjectNotFoundException;
/**
* Removes a {@link User} from the requested {@link Group}
* @param groupId id of the requested group
* @param userId id of the user to be removed
* @throws ObjectNotFoundException if group or user with the specified id does not exist
*/
void removeUserFromGroup(Long groupId, Long userId) throws ObjectNotFoundException;
/**
* Updates {@link Group} {@link Entitlements}.
* Note that old {@link Entitlements} are removed and replaced with a new collection.
*
* @param groupId id of the requested {@link Group}
* @param entitlements {@link Entitlements} to be updated
*
* @throws ObjectNotFoundException if group with the specified id does not exist
* @throws ValidationException if entitlement validation fails
*
* @return updated entitlements wrapped into {@link Entitlements} object
*/
Entitlements updateGroupEntitlements(Long groupId, Entitlements entitlements) throws ObjectNotFoundException, ValidationException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy