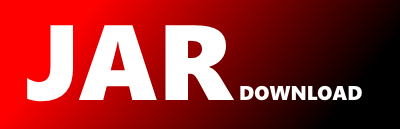
org.xlcloud.service.api.ProjectsApi Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service.api;
import org.xlcloud.rest.exception.AuthenticateException;
import org.xlcloud.rest.exception.DuplicatedEntityException;
import org.xlcloud.rest.exception.ObjectNotFoundException;
import org.xlcloud.rest.exception.ValidationException;
import org.xlcloud.service.Account;
import org.xlcloud.service.Entitlements;
import org.xlcloud.service.Layer;
import org.xlcloud.service.Lease;
import org.xlcloud.service.Leases;
import org.xlcloud.service.NetworkConfiguration;
import org.xlcloud.service.Project;
import org.xlcloud.service.Projects;
import org.xlcloud.service.Stack;
import org.xlcloud.service.StackBlueprint;
import org.xlcloud.service.Stacks;
import org.xlcloud.service.Subnet;
import org.xlcloud.service.User;
import org.xlcloud.service.Users;
import org.xlcloud.service.heat.template.Template;
/**
* Interface for API that allows to manage {@link Project}'s and related resources.
*
* @author Tomek Adamczewski, AMG.net
* @author Konrad Król, AMG.net
*/
public interface ProjectsApi {
/**
* @return the collection of all {@link Projects}
*/
Projects list();
/**
* Returns details of {@link Project} identified by the given id
*
* @param projectId
* id of the {@link Project}
* @return {@link Project} details
* @throws ObjectNotFoundException
* if project with specified ID not found
*/
Project get(Long projectId) throws ObjectNotFoundException;
/**
* Deletes the {@link Project} by id
* @param projectId
* id of the {@link Project}
* @throws ObjectNotFoundException if project with specified ID not found
*/
Project remove(Long projectId) throws ObjectNotFoundException;
/**
* List {@link Users} assigned to the {@link Project}
*
* @param projectId
* id of the {@link Project}
* @return list of project {@link User}'s wrapped in {@link Users} object (or an
* empty {@link Users} wrapper if no {@link User} exist)
* @throws ObjectNotFoundException
* if project with specified ID not found
*/
Users listUsers(Long projectId) throws ObjectNotFoundException;
/**
* Create new {@link User} and assign to a {@link Project}. {@link User} will be automatically
* assigned to {@link Account}, but won't have any {@link Entitlements} (hence, this method
* might not have much sense and can be deleted later on)
*
* @param projectId
* id of the {@link Project}
* @param user
* id of the {@link User}
* @return newly-created {@link User} details
* @throws ObjectNotFoundException
* if project with specified ID not found
* @throws ValidationException
* if user entity is not valid (e.g. missing login or password)
*/
User createUser(Long projectId, User user) throws ObjectNotFoundException, ValidationException;
/**
* Assign existing {@link User} to a {@link Project}
*
* @param projectId
* id of the {@link Project}
* @param userId
* id of the {@link User}
* @throws ObjectNotFoundException
* if project or user with specified ID not found
* @throws ValidationException
* if user is not in the same account as project (user is not
* assigned to account at all or he's assigned to different
* account)
*/
void assignUser(Long projectId, Long userId) throws ObjectNotFoundException, ValidationException;
/**
* Remove {@link User} from {@link Project}, but does not delete the {@link User} (just remove the
* relationship)
*
* @param projectId
* id of the {@link Project}
* @param userId
* id of the {@link User}
* @throws ObjectNotFoundException if project or user with specified ID not found
*/
void removeUser(Long projectId, Long userId) throws ObjectNotFoundException;
/**
* Creates a new {@link Stack} assigned to a given project.
* The template and layers are cloned from blueprint.
*
* @param projectId
* id of the {@link Project}
* @param blueprintId
* id of the {@link StackBlueprint}
* @param stack
* id of the {@link Stack}
* @return newly-created {@link Stack}
* @throws DuplicatedEntityException
* if {@link Stack} with this name already exists in the {@link Project}
* @throws ValidationException
* missing stack name
* @throws ObjectNotFoundException
* if {@link StackBlueprint} or {@link Project} does not exist
*/
Stack createStack(Long projectId, Long blueprintId, Stack stack) throws DuplicatedEntityException, ValidationException,
ObjectNotFoundException;
/**
* Creates a new {@link Stack} assigned to a given project.
*
* @param projectId
* id of the {@link Project}
* @param stack
* id of the {@link Stack}
* @return newly-created {@link Stack}
* @throws DuplicatedEntityException
* if {@link Stack} with this name already exists in the {@link Project}
* @throws ValidationException
* missing stack name
* @throws ObjectNotFoundException
* if {@link StackBlueprint} or {@link Project} does not exist
*/
Stack createStack(Long projectId, Stack stack) throws DuplicatedEntityException, ValidationException,
ObjectNotFoundException;
/**
* List all {@link Stacks} in {@link Project}
*
* @param projectId
* id of the {@link Project}
* @return list of project {@link Stack}'s wrapped in {@link Stacks} object (or an
* empty {@link Stacks} wrapper if no {@link Stack} exist)
* @throws ObjectNotFoundException
* if project with specified ID not found
*/
Stacks listStacks(Long projectId) throws ObjectNotFoundException;
/**
* Creates a new {@link Stack} assigned to a given project. The stack is
* created only on base of the given {@link Template}.
*
* @param projectId
* id of the {@link Project}
* @param template
* {@link Template} which should be used to create {@link Stack}
* @param stackName
* - name of the {@link Stack} to be created
* @return {@link Template}
*/
Stack createStackFromTemplate(Long projectId, Template template, String stackName);
/**
* Adds a {@link Subnet} to the existing {@link NetworkConfiguration} attached to the {@link Project} identified by the given projectId
*
* @param projectId
* - id of the {@link Project}
* @param subnet
* - {@link Subnet} to be added
* @return created {@link Subnet} with UUID
* @throws ObjectNotFoundException - if such {@link NetworkConfiguration} does not exists
* @throws ValidationException - if the given {@link Subnet}'s CIDR conflicts with CIDRs that already exists in the {@link Project}
* or if the {@link Subnet} name is not unique in the {@link Project}
* @throws AuthenticateException
* user could not be authenticated in OpenStack
*/
public Subnet addSubnet(Long projectId, Subnet subnet) throws ObjectNotFoundException, ValidationException, AuthenticateException;
/**
* Removes {@link Subnet} from the existing {@link NetworkConfiguration} attached to the {@link Project} identified by the given projectId
*
* @param projectId
* - id of the {@link Project}
* @param uuid
* - uuid of the {@link Subnet} to be removed
* @throws ObjectNotFoundException - if such {@link NetworkConfiguration} or {@link Subnet} does not exists
* @throws AuthenticateException
* user could not be authenticated in OpenStack
*/
public void removeSubnet(Long projectId, String uuid) throws ObjectNotFoundException, AuthenticateException;
/**
* Returns {@link NetworkConfiguration} attached to {@link Project} identified by the given projectId
* @param projectId
* - id of the {@link Project}
* @return {@link Project}'s {@link NetworkConfiguration}
* @throws ObjectNotFoundException - if {@link NetworkConfiguration} for such {@link Project} does not exists
*/
public NetworkConfiguration getNetworkConfiguration(Long projectId) throws ObjectNotFoundException;
/**
* Updates default subnet for the {@link NetworkConfiguration} attached to the {@link Project} identified by the given projectId
* @param projectId
* - id of the {@link Project}
* @param subnet
* - subnet with {@link Subnet}'s name
* @throws ObjectNotFoundException
* - if {@link NetworkConfiguration} for such {@link Project} does not exist
* @throws ValidationException
* - if {@link Subnet} with given name was not found in {@link Project}'s {@link NetworkConfiguration}
*/
public void updateDefaultSubnet(Long projectId, Subnet subnet) throws ObjectNotFoundException, ValidationException;
/**
* Creates new layer on the base of template and wrap it in a stack.
* @param template
*/
Stack createStackWrappingLayerTemplate(Long projectId, Template template, String stackName);
/**
* Creates a new {@link Lease} for the specified {@link Project}.
*
* @param projectId
* id of the {@link Project}
* @param lease
* {@link Lease} to be created
* @return newly-created lease details
* @throws ObjectNotFoundException
* project could not be found
* @throws ValidationException
* invalid lease
* @throws DuplicatedEntityException
* lease with specified name already exists in the project
*/
Lease createLease(Long projectId, Lease lease) throws ObjectNotFoundException, ValidationException, DuplicatedEntityException;
/**
* Returns details of a {@link Lease} identified by the given id
*
* @param projectId
* id of the {@link Project} the lease is associated with
* @param leaseId id of the lease
* @return {@link Lease} details
* @throws ObjectNotFoundException
* if project or lease with specified id not found
*/
Lease getLease(Long projectId, String leaseId) throws ObjectNotFoundException;
/**
* @return the collection of all {@link Lease}s in the specified project
*/
Leases listLeases(Long projectId);
/**
* Updates a specified {@link Lease}.
*
* @param projectId
* id of the {@link Project}
* @param lease
* new {@link Lease}
* @return current lease details
* @throws ObjectNotFoundException
* project or lease could not be found
* @throws ValidationException
* invalid lease, lease is active
* @throws DuplicatedEntityException
* lease with specified name already exists in the project
*/
Lease updateLease(Long projectId, String leaseId, Lease lease) throws ObjectNotFoundException, ValidationException, DuplicatedEntityException;
/**
* Deletes the {@link Lease} by the id
* @param projectId
* id of the {@link Project} the lease is associated with
* @param leaseId id of the lease
* @throws ValidationException
* invalid lease, lease is active
*/
void deleteLease(Long projectId, String leaseId) throws ValidationException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy