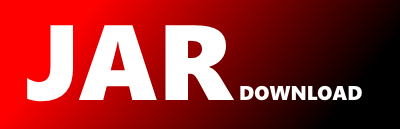
org.xlcloud.service.api.StacksApi Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service.api;
import org.xlcloud.rest.exception.ObjectNotFoundException;
import org.xlcloud.service.Layer;
import org.xlcloud.service.LayerBlueprint;
import org.xlcloud.service.ParametersValues;
import org.xlcloud.service.Session;
import org.xlcloud.service.Stack;
import org.xlcloud.service.Stacks;
import org.xlcloud.service.heat.template.Template;
import org.xlcloud.service.model.stack.Event;
import org.xlcloud.service.model.stack.Events;
/**
* Interface implemented by XMS {@link Stack} resource in XMS api
*
* @author Tomek Adamczewski, AMG.net
* @author Piotr Kulasek-Szwed, AMG.net
* @author Konrad Król, AMG.net
*/
public interface StacksApi {
/**
* Returns all {@link Stacks}
* @return {@link Stack} list wrapped in {@link Stacks} object.
*/
Stacks list();
/**
* Return the {@link Stack} by id
* @param stackId requested {@link Stack} id
* @return {@link Stack} details
* @throws ObjectNotFoundException if {@link Stack} with the specified id does not exist
*/
Stack get(Long stackId) throws ObjectNotFoundException;
/**
* Removes {@link Stack} and it's session (if any).
* @param stackId
* - requested {@link Stack} id
*/
void remove(Long stackId);
/**
* Creates {@link Stack} {@link Session}
* @param stackId requested {@link Stack} id
* @return created {@link Session}
* @throws ObjectNotFoundException if {@link Stack} with the specified id does not exist
*/
Session createSession(Long stackId) throws ObjectNotFoundException;
/**
* Removes the {@link Stack} session
* @param stackId requested {@link Stack} id
* @throws ObjectNotFoundException if {@link Stack} with the specified id does not exist
*/
void removeSession(Long stackId) throws ObjectNotFoundException;
/**
* Returns the {@link Stack} session.
*
* @param stackId requested {@link Stack} id
* @return {@link Stack} session
* @throws ObjectNotFoundException if {@link Stack} with the specified id does not exist
*/
Session getSession(Long stackId) throws ObjectNotFoundException;
/**
* Updates {@link Stack}'s {@link Session}
* @param stackId
* requested {@link Stack} id
* @param session
* {@link Session} with new status
* @return {@link Session} after update
* @throws ObjectNotFoundException if {@link Stack} with the specified id does not exist
*/
Session updateSessionStatus(Session session, Long stackId) throws ObjectNotFoundException;
/**
* Returns current values for {@link Stack} parameters
* @param stackId
* - id of the {@link Stack}
* @return collection of current values for {@link Stack} parameters
*/
ParametersValues getParametersValues(Long stackId);
/**
* Updates current values for {@link Stack} parameters
* @param stackId
* - id of the {@link Stack}
* @param parametersValues
* - collection of current values for {@link Stack} parameters
* @return collection of current values for {@link Stack} parameters
*/
ParametersValues updateParametersValues(Long stackId, ParametersValues parametersValues);
/**
* Updates stack template
* @param stackId
* id of the {@link Stack}
* @param template - template object
*/
Template updateStackTemplate(Long stackId, Template templateObject);
/**
* Creates a new {@link Layer} assigned to the given {@link Stack}.
* The template is cloned from the blueprint. If the layer has current values they will be used.
* @param stackId
* id of the {@link Stack}
* @param blueprintId
* id of the {@link LayerBlueprint}
* @param layer
* {@link Layer} details
* @return newly created {@link Layer}
*/
Layer createStackLayer(Long stackId, Long blueprintId, Layer layer);
/**
* Creates a new {@link Layer} assigned to the given {@link Stack}.
* If the layer has current values they will be used.
* @param stackId
* id of the {@link Stack}
* @param layer
* {@link Layer} details
* @return newly created {@link Layer}
*/
Layer createStackLayer(Long stackId, Layer layer);
/**
* List {@link Event} from {@link Stack} and all {@link Layer}
* @param stackId
* id of the {@link Stack}
* @param layerId
* id of the {@link Layer}
*/
Events listEvents(Long stackId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy