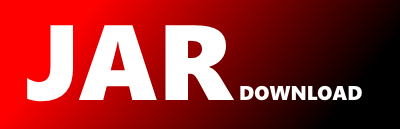
org.xlcloud.service.builders.InstanceBuilder Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service.builders;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.lang.StringUtils;
import org.json.JSONException;
import org.json.JSONObject;
import org.xlcloud.service.Instance;
import org.xlcloud.service.InstanceType;
import org.xlcloud.service.Instances;
import org.xlcloud.service.heat.template.fields.ResourceType;
import org.xlcloud.service.heat.template.resources.Eip;
import org.xlcloud.service.heat.template.resources.EipAssociation;
import org.xlcloud.service.heat.template.resources.LaunchConfiguration;
import org.xlcloud.service.heat.template.resources.ResourceBase;
import org.xlcloud.service.heat.template.resources.Resources;
import org.xlcloud.service.heat.template.resources.ScalingGroupBase;
public class InstanceBuilder {
private Instances object;
protected InstanceBuilder(Instances obj) {
this.object = obj;
}
public static InstanceBuilder newInstance() {
return new InstanceBuilder(new Instances());
}
public InstanceBuilder fromResources(Resources resources) {
for(ResourceBase resource : resources.getResource()) {
if(ResourceType.LAUNCH_CONFIGURATION.equals(resource.getResourceType())) {
this.object.getInstance().add(parseScalableInstance((LaunchConfiguration)resource,resources));
} else if(ResourceType.INSTANCE.equals(resource.getResourceType())) {
this.object.getInstance().add(parseNonScalableInstance((org.xlcloud.service.heat.template.resources.Instance)resource,resources));
}
}
return this;
}
private Instance parseScalableInstance(LaunchConfiguration launchConfigResource, Resources resources) {
Instance instance = new Instance();
instance.setName(launchConfigResource.getName());
instance.setInstanceType(InstanceType.SCALABLE);
instance.getResources().getResource().add(launchConfigResource);
for (ResourceBase resource : resources.getResource()) {
if (resource.getResourceType().equals(ResourceType.AUTO_SCALING_GROUP)
|| resource.getResourceType().equals(ResourceType.INSTANCE_GROUP)) {
ScalingGroupBase scalingGroup = (ScalingGroupBase) resource;
if (referencesLaunchConfiguration(scalingGroup.getLaunchConfigurationName(), launchConfigResource)) {
instance.getScalingGroups().getScalingGroup().add(scalingGroup);
}
}
}
return instance;
}
private Instance parseNonScalableInstance(org.xlcloud.service.heat.template.resources.Instance templateInstance, Resources resources) {
Instance instance = new Instance();
instance.setName(templateInstance.getName());
instance.setInstanceType(InstanceType.NON_SCALABLE);
instance.getResources().getResource().add(templateInstance);
// First, find all EIPs.
List eipNames = new ArrayList<>();
for (ResourceBase resource : resources.getResource()) {
if (resource.getResourceType().equals(ResourceType.EIP)) {
eipNames.add(resource.getName());
}
}
// Then, find EIP Associations associated with this instance for which corresponding EIP exists.
List referencedEipNames = new ArrayList<>();
for (ResourceBase resource : resources.getResource()) {
if (resource.getResourceType().equals(ResourceType.EIP_ASSOCIATION)) {
EipAssociation eipAssociation = (EipAssociation) resource;
if (instance.getName().equals(parseReference(eipAssociation.getInstanceId()))
&& eipNames.contains(parseReference(eipAssociation.getEip()))) {
instance.getEipAssociations().getEipAssociation().add(eipAssociation);
referencedEipNames.add(parseReference(eipAssociation.getEip()));
}
}
}
// Finally, find EIPs associated with this instance either directly or through an EIP Association.
for (ResourceBase resource : resources.getResource()) {
if (resource.getResourceType().equals(ResourceType.EIP)) {
Eip eip = (Eip) resource;
if (referencedEipNames.contains(eip.getName()) || instance.getName().equals(parseReference(eip.getInstanceId()))) {
instance.getEips().getEip().add(eip);
}
}
}
return instance;
}
public Instances build() {
return object;
}
private boolean referencesLaunchConfiguration(String reference, LaunchConfiguration launchConfigResource) {
return StringUtils.equals(reference, launchConfigResource.getName()) ||
StringUtils.equals(parseReference(reference), launchConfigResource.getName()) ;
}
private String parseReference(String reference) {
if (StringUtils.isBlank(reference)) {
return "";
}
try {
return new JSONObject(reference).getString("Ref");
} catch (JSONException e) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy