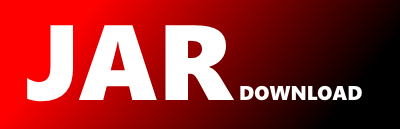
org.xlcloud.service.parsers.ChefFileParser Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.service.parsers;
import static org.xlcloud.service.heat.template.commons.HeatTemplateValueBuilder.fnJoin;
import static org.xlcloud.service.heat.template.commons.HeatTemplateValueBuilder.string;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.lang.StringUtils;
import org.json.JSONException;
import org.xlcloud.rest.exception.ValidationException;
import org.xlcloud.rest.exception.ValidationException.ValidationFailureType;
import org.xlcloud.service.ChefCookbook;
import org.xlcloud.service.ChefFile;
import org.xlcloud.service.heat.template.commons.HeatTemplateValue;
import org.xlcloud.service.heat.template.commons.HeatTemplateValueBuilder;
import org.xlcloud.service.heat.template.commons.StringHeatTemplateValue;
import org.xlcloud.service.heat.template.commons.functions.FnJoin;
/**
* Parser for {@link ChefFile} based on {@link HeatTemplateValue}
*
* @author Michał Kamiński, AMG.net
*/
public class ChefFileParser {
public HeatTemplateValue fromCheffile(ChefFile cheffile) throws JSONException {
List fnJoinValues = new ArrayList<>();
fnJoinValues.add(string("#!/usr/bin/env ruby"));
fnJoinValues.add(string("site 'http://community.opscode.com/api/v1'"));
if (cheffile != null) {
for (ChefCookbook cookbook : cheffile.getCookbooks()) {
fnJoinValues.add(string("cookbook '" + cookbook.getCookbook() + "'"));
if (StringUtils.isNotBlank(cookbook.getGit())) {
fnJoinValues.add(string(" :git => '" + cookbook.getGit() + "',"));
}
fnJoinValues.add(string(" :path => '" + cookbook.getCookbook() + "'"));
}
}
return fnJoin("\n", fnJoinValues.toArray(new HeatTemplateValue[fnJoinValues.size()]));
}
public ChefFile toChefFile(HeatTemplateValue value) throws JSONException {
if (value instanceof StringHeatTemplateValue) {
return fromString((StringHeatTemplateValue) value);
} else if (value instanceof FnJoin) {
return fromFnJoin((FnJoin) value);
} else {
throw new ValidationException("format: " + value.getClass() + " is not supported", ValidationFailureType.CHEFFILE_FORMAT);
}
}
private ChefFile fromFnJoin(FnJoin fnJoin) throws JSONException {
String delimiter = fnJoin.getDelimiter();
List values = fnJoin.getContent();
List stringValues = new ArrayList<>();
for (HeatTemplateValue fnJoinValue : values) {
stringValues.add(fnJoinValue.get().toString());
}
String stringRepresentation = StringUtils.join(stringValues, delimiter);
return fromString(HeatTemplateValueBuilder.string(stringRepresentation));
}
private ChefFile fromString(StringHeatTemplateValue value) {
ChefFile chefFile = new ChefFile();
String[] lines = StringUtils.split(value.get(), "\n");
ChefCookbook cookbook = null;
for (String line : lines) {
line = StringUtils.trim(line);
if (line.startsWith("cookbook")) {
if (cookbook != null) {
chefFile.getCookbooks().add(cookbook);
}
cookbook = new ChefCookbook();
cookbook.setCookbook(StringUtils.substringBetween(line, "'", "'"));
}
if (line.startsWith(":git")) {
cookbook.setGit(StringUtils.substringBetween(line, "'", "'"));
}
}
if (cookbook != null) {
chefFile.getCookbooks().add(cookbook);
}
return chefFile;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy