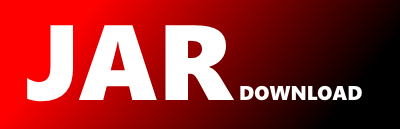
org.xlcloud.openstack.climate.client.OpenstackClimateClient Maven / Gradle / Ivy
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.openstack.climate.client;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.PostConstruct;
import javax.inject.Inject;
import org.apache.log4j.Logger;
import org.xlcloud.config.ConfigParam;
import org.xlcloud.openstack.client.WebResourceRequestBuilderDecorator;
import org.xlcloud.openstack.climate.api.ReservationClient;
import org.xlcloud.openstack.model.climate.Lease;
import org.xlcloud.openstack.model.climate.Leases;
import org.xlcloud.openstack.model.climate.client.GetLeaseResponse;
import org.xlcloud.openstack.model.exceptions.OpenStackException;
import org.xlcloud.openstack.rest.OpenStackRestClient;
import org.xlcloud.openstack.rest.RestClientType;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.WebResource;
/**
* This interface provides a concise way to manipulate Climate API
*
* @author Michał Kamiński, AMG.net
*/
public class OpenstackClimateClient extends WebResourceRequestBuilderDecorator implements ReservationClient {
private static final Logger LOG = Logger.getLogger(OpenstackClimateClient.class);
private static final String LEASES = "leases";
// private static final String HOSTS = "os-hosts";
@Inject
@OpenStackRestClient(RestClientType.HEAT)
private Client resourceClient;
@Inject
@ConfigParam
private String climateUrl;
private WebResource resource;
@PostConstruct
public void init() {
LOG.debug("Using Jersey client " + resourceClient.hashCode());
resource = resourceClient.resource(climateUrl);
}
/** {@inheritDoc} */
@Override
public Lease createLease(Lease lease) {
GetLeaseResponse leaseResponse = post(GetLeaseResponse.class, lease, resource.path(LEASES));
if (leaseResponse == null || leaseResponse.getLease() == null) {
throw new OpenStackException(500, "Could not create lease");
}
return leaseResponse.getLease();
}
/** {@inheritDoc} */
@Override
public List listAllLeases() {
return get(Leases.class, resource.path(LEASES)).getLeases();
}
/** {@inheritDoc} */
@Override
public List listLeasesByTenant(String tenantId) {
List allLeases = listAllLeases();
List tenantLeases = new ArrayList();
for (Lease lease : allLeases) {
if (tenantId.equals(lease.getTenantId())) {
tenantLeases.add(lease);
}
}
return tenantLeases;
}
/** {@inheritDoc} */
@Override
public Lease getLease(String leaseId) {
GetLeaseResponse leaseResponse = get(GetLeaseResponse.class, resource.path(LEASES).path(leaseId));
if (leaseResponse == null) {
throw new OpenStackException(404, "Lease not found");
}
return leaseResponse.getLease();
}
/** {@inheritDoc} */
@Override
public Lease updateLease(String leaseId, Lease lease) {
GetLeaseResponse leaseResponse = put(GetLeaseResponse.class, lease, resource.path(LEASES).path(leaseId));
if (leaseResponse == null) {
throw new OpenStackException(500, "Could not update lease");
}
return leaseResponse.getLease();
}
/** {@inheritDoc} */
@Override
public void removeLease(String leaseId) {
delete(resource.path(LEASES).path(leaseId));
}
// @Override
// public List listHosts() {
// return get(Hosts.class, resource.path(HOSTS)).getHosts();
// }
//
// @Override
// public Host getHosts(String hostId) {
// return get(Host.class, resource.path(HOSTS));
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy