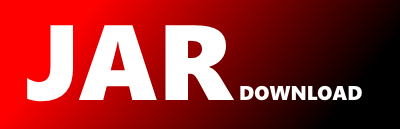
org.xlcloud.openstack.model.climate.Lease Maven / Gradle / Ivy
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.openstack.model.climate;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.xlcloud.openstack.model.climate.json.OpenStackDateDeserializer;
import org.xlcloud.openstack.model.climate.json.OpenStackDateSerializer;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
/**
* Entity enables to create basic reservation of physical resources
*
* @author Michał Kamiński, AMG.net
*/
public class Lease {
@JsonProperty( "id" )
private String id;
@JsonProperty( "user_id" )
private String userId;
@JsonProperty( "name" )
private String name;
@JsonProperty( "start_date" )
@JsonSerialize( using = OpenStackDateSerializer.class )
@JsonDeserialize( using = OpenStackDateDeserializer.class )
@JsonInclude( Include.NON_NULL )
private Date startDate;
@JsonProperty( "end_date" )
@JsonSerialize( using = OpenStackDateSerializer.class )
@JsonDeserialize( using = OpenStackDateDeserializer.class )
@JsonInclude( Include.NON_NULL )
private Date endDate;
@JsonProperty( "created_at" )
@JsonSerialize( using = OpenStackDateSerializer.class )
@JsonDeserialize( using = OpenStackDateDeserializer.class )
@JsonInclude( Include.NON_NULL )
private Date createdAt;
@JsonProperty( "updated_at" )
@JsonSerialize( using = OpenStackDateSerializer.class )
@JsonDeserialize( using = OpenStackDateDeserializer.class )
@JsonInclude( Include.NON_NULL )
private Date updatedAt;
@JsonProperty( "reservations" )
List reservations;
@JsonProperty( "events" )
List events;
@JsonProperty( "tenant_id" )
private String tenantId;
@JsonProperty( "trust_id" )
private String trustId;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getStartDate() {
return startDate;
}
public void setStartDate(Date startDate) {
this.startDate = startDate;
}
public Date getEndDate() {
return endDate;
}
public void setEndDate(Date endDate) {
this.endDate = endDate;
}
public List getReservations() {
if (reservations == null) {
reservations = new ArrayList();
}
return reservations;
}
public void setReservations(List reservations) {
this.reservations = reservations;
}
public List getEvents() {
if (events == null) {
events = new ArrayList();
}
return events;
}
public String getTenantId() {
return tenantId;
}
public void setTenantId(String tenantId) {
this.tenantId = tenantId;
}
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
public Date getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(Date updatedAt) {
this.updatedAt = updatedAt;
}
public String getTrustId() {
return trustId;
}
public void setTrustId(String trustId) {
this.trustId = trustId;
}
@JsonIgnore
public Event getStartEvent() {
return getEventByType(EventType.START);
}
@JsonIgnore
public Event getEndEvent() {
return getEventByType(EventType.END);
}
@JsonIgnore
private Event getEventByType(EventType type) {
for (Event event : getEvents()) {
if (type.equals(event.getEventType())) {
return event;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy