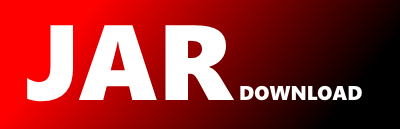
org.xlcloud.openstack.model.climate.Reservation Maven / Gradle / Ivy
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.openstack.model.climate;
import java.util.Date;
import org.apache.commons.lang.StringUtils;
import org.xlcloud.openstack.model.climate.json.OpenStackDateDeserializer;
import org.xlcloud.openstack.model.climate.json.OpenStackDateSerializer;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
/**
* Base reservation
*
* @author Michał Kamiński, AMG.net
*/
public class Reservation {
@JsonProperty( "id" )
private String id;
@JsonProperty( "resource_id" )
private String resourceId;
@JsonProperty( "status" )
private String status;
@JsonProperty( "resource_type" )
private String resourceType;
@JsonProperty( "min" )
private Long minSize;
@JsonProperty( "max" )
private Long maxSize;
@JsonProperty( "size" )
private Long size;
@JsonProperty( "lease_id" )
private String leaseId;
@JsonProperty( "created_at" )
@JsonSerialize( using = OpenStackDateSerializer.class )
@JsonDeserialize( using = OpenStackDateDeserializer.class )
@JsonInclude( Include.NON_NULL )
private Date createdAt;
@JsonProperty( "updated_at" )
@JsonSerialize( using = OpenStackDateSerializer.class )
@JsonDeserialize( using = OpenStackDateDeserializer.class )
@JsonInclude( Include.NON_NULL )
private Date updatedAt;
@JsonProperty( "hypervisor_properties" )
@JsonInclude( Include.ALWAYS )
private String hypervisorProperties = StringUtils.EMPTY;
@JsonProperty( "resource_properties" )
@JsonInclude( Include.ALWAYS )
private String resourceProperties = StringUtils.EMPTY;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getResourceId() {
return resourceId;
}
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getResourceType() {
return resourceType;
}
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
public Long getMinSize() {
return minSize;
}
public void setMinSize(Long minSize) {
this.minSize = minSize;
}
public Long getMaxSize() {
return maxSize;
}
public void setMaxSize(Long maxSize) {
this.maxSize = maxSize;
}
public Long getSize() {
return size;
}
public void setSize(Long size) {
this.size = size;
}
public String getHypervisorProperties() {
return hypervisorProperties;
}
public void setHypervisorProperties(String hypervisorProperties) {
if (hypervisorProperties != null) {
this.hypervisorProperties = hypervisorProperties;
}
}
public String getResourceProperties() {
return resourceProperties;
}
public void setResourceProperties(String resourceProperties) {
if (resourceProperties != null) {
this.resourceProperties = resourceProperties;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy