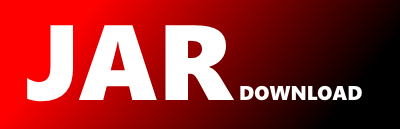
org.xlcloud.openstack.client.OpenStackHeatClient Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.openstack.client;
import javax.annotation.PostConstruct;
import javax.inject.Inject;
import javax.ws.rs.core.MediaType;
import org.apache.log4j.Logger;
import org.xlcloud.config.ConfigParam;
import org.xlcloud.openstack.api.HeatClient;
import org.xlcloud.openstack.model.context.HeatContext;
import org.xlcloud.openstack.model.exceptions.OpenStackException;
import org.xlcloud.openstack.model.exceptions.OpenStackNotFoundException;
import org.xlcloud.openstack.model.heat.HeatStack;
import org.xlcloud.openstack.model.heat.StackAction;
import org.xlcloud.openstack.model.heat.StackAction.StackActionType;
import org.xlcloud.openstack.model.heat.StackData;
import org.xlcloud.openstack.model.heat.StackResources;
import org.xlcloud.openstack.rest.OpenStackRestClient;
import org.xlcloud.openstack.rest.RestClientType;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.UniformInterfaceException;
import com.sun.jersey.api.client.WebResource;
/**
* This interface provides a concise way to manipulate stacks - supporting
* create, delete and get details operations. This class is the main
* implementation of the {@link HeatClient}.
*
* @author Ksiazczyk Grzegorz, AMG.net
* @author Konrad Król, AMG.net
*/
public class OpenStackHeatClient extends WebResourceRequestBuilderDecorator implements HeatClient {
private static final Logger LOG = Logger.getLogger(OpenStackHeatClient.class);
private static final String STACKS = "stacks";
@Inject
@OpenStackRestClient(RestClientType.HEAT)
private Client resourceClient;
@Inject
@ConfigParam
private String heatUrl;
@Inject
HeatContext heatContext;
private WebResource resource;
@PostConstruct
public void init() {
LOG.debug("Using Jersey client " + resourceClient.hashCode());
resource = resourceClient.resource(heatUrl);
}
/** {@inheritDoc} */
@Override
public void createStack(HeatStack stack) {
post(stack, resource.path(heatContext.getOsProjectId()).path(STACKS), MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML);
}
/** {@inheritDoc} */
@Override
public void updateStack(String stackName, String stackId, HeatStack stack) {
put(stack, resource.path(heatContext.getOsProjectId()).path(STACKS).path(stackName).path(stackId));
}
/** {@inheritDoc} */
@Override
public void deleteStack(String stackNameOrId) {
LOG.info("deleting stack with name or id: " + stackNameOrId);
delete(resource.path(heatContext.getOsProjectId()).path(STACKS).path(stackNameOrId));
}
/** {@inheritDoc} */
@Override
public StackData getStackDetails(String stackName) {
StackData stackData = get(StackData.class, resource.path(heatContext.getOsProjectId()).path(STACKS).path(stackName));
heatContext.setHeatStackId(stackData.getId());
heatContext.setHeatStackName(stackData.getStack_name());
return stackData;
}
/** {@inheritDoc} */
@Override
public StackResources getStackResources(String stackId) {
return get(StackResources.class, resource.path(heatContext.getOsProjectId()).path(STACKS).path(stackId).path("resources"));
}
/** {@inheritDoc} */
@Override
public void validateTemplate(String template) throws UniformInterfaceException, OpenStackException {
post("{ \"template\": " + template + "}", resource.path("validate"));
}
/** {@inheritDoc} */
@Override
public void suspendStack(String stackName) {
sendStackAction(stackName, new StackAction(StackActionType.SUSPEND));
}
/** {@inheritDoc} */
@Override
public void resumeStack(String stackName) {
sendStackAction(stackName, new StackAction(StackActionType.RESUME));
}
private void sendStackAction(String stackName, StackAction action) {
StackData stack = getStackDetails(stackName);
if (stack == null) {
throw new OpenStackNotFoundException("Stack with name" + stackName + "not found");
}
post(action, resource.path(heatContext.getOsProjectId()).path(STACKS).path(stackName).path(stack.getId()).path("actions"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy