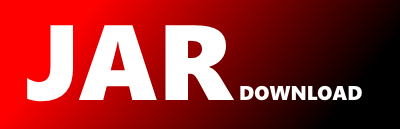
org.xlcloud.rest.exception.BaseException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-utils Show documentation
Show all versions of rest-utils Show documentation
This module is provides the common REST utils, mostly for server side of an application, like exceptions and interceptors.
The newest version!
/*
* Copyright 2012 AMG.lab, a Bull Group Company
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.xlcloud.rest.exception;
import java.io.Serializable;
import javax.ejb.ApplicationException;
import javax.ws.rs.WebApplicationException;
import javax.ws.rs.core.Response;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonSubTypes.Type;
/**
* XLcloud base exception class. Should be used to report internal application
* errors.
*
* @author Andrzej Stasiak, AMG.net
* @author Maciej Osyda, AMG.net
* @author Piotr Kulasek-Szwed, AMG.net
*/
@ApplicationException(rollback = true)
public class BaseException extends WebApplicationException {
private static final long serialVersionUID = 1334062680680159882L;
@Deprecated // For JAXB only.
public BaseException() {}
protected BaseException( int statusCode, ExceptionDetailsBase exceptionDetails ) {
super(Response.status(statusCode).entity(exceptionDetails).build());
}
@Deprecated
public BaseException( int statusCode, String message ) {
this(statusCode, new ExceptionDetails(message));
}
public String getExceptionMessage() {
try {
return ((ExceptionDetailsBase) getResponse().getEntity()).getMessage();
} catch (ClassCastException e) {
return getMessage();
}
}
public ExceptionDetailsBase getDetails() {
return ((ExceptionDetailsBase) getResponse().getEntity());
}
@XmlAccessorType(XmlAccessType.FIELD)
@XmlRootElement
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.PROPERTY, property = "type")
@JsonSubTypes({
@Type(value = BaseException.ExceptionDetails.class, name = "base"),
@Type(value = AuthenticateException.ExceptionDetails.class, name = "authentication"),
@Type(value = DuplicatedEntityException.ExceptionDetails.class, name = "duplicated"),
@Type(value = ForbiddenException.ExceptionDetails.class, name = "forbidden"),
@Type(value = InternalErrorException.ExceptionDetails.class, name = "internal"),
@Type(value = ObjectNotFoundException.ExceptionDetails.class, name = "object_not_found"),
@Type(value = QuotaException.ExceptionDetails.class, name = "quota"),
@Type(value = ValidationException.ExceptionDetails.class, name = "validation"),
})
public static abstract class ExceptionDetailsBase implements Serializable {
private static final long serialVersionUID = 8052920653304448958L;
@XmlAttribute
private String message;
@Deprecated // For JAXB only.
public ExceptionDetailsBase() {}
public ExceptionDetailsBase( String message ) {
this.message = message;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public abstract String getDetails();
}
public static class ExceptionDetails extends ExceptionDetailsBase {
private static final long serialVersionUID = 1L;
@Deprecated // For JAXB only.
public ExceptionDetails() {}
public ExceptionDetails( String message ) {
super(message);
}
public String getDetails() {
return "";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy