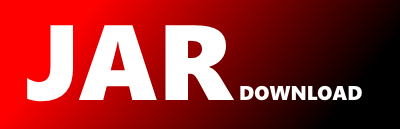
org.owasp.jbrofuzz.headers.HeaderLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbrofuzz Show documentation
Show all versions of jbrofuzz Show documentation
JBroFuzz is a stateless web application fuzzer for requests
being made over HTTP and/or HTTPS. Its purpose is to provide a single,
portable application that offers stable web protocol fuzzing capabilities.
As a tool, it emerged from the needs of penetration testing.
The newest version!
/**
* JbroFuzz 2.5
*
* JBroFuzz - A stateless network protocol fuzzer for web applications.
*
* Copyright (C) 2007 - 2010 [email protected]
*
* This file is part of JBroFuzz.
*
* JBroFuzz is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JBroFuzz is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with JBroFuzz. If not, see .
* Alternatively, write to the Free Software Foundation, Inc., 51
* Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
*
* Verbatim copying and distribution of this entire program file is
* permitted in any medium without royalty provided this notice
* is preserved.
*
*/
package org.owasp.jbrofuzz.headers;
import java.util.Enumeration;
import java.util.Map;
import javax.swing.tree.TreePath;
import org.apache.commons.lang.ArrayUtils;
import org.apache.commons.lang.StringUtils;
import org.owasp.jbrofuzz.core.Prototype;
import org.owasp.jbrofuzz.core.Verifier;
/**
* Class responsible for loading the headers
* from file.
*
* @author [email protected]
* @version 2.3
* @since 2.3
*
*/
class HeaderLoader {
private static final String HEADER = "JBroFuzz Headers Collection";
private static final int MAX_RECURSION = 1024;
private final HeaderTreeNode myNode;
private int globalCounter;
private final Map headersMap;
public HeaderLoader() {
myNode = new HeaderTreeNode(HEADER);
globalCounter = 0;
headersMap = Verifier.loadFile("headers.jbrf");
}
/**
*
* Private, recursive method used at construction to populate the master
* HeaderTreeNode
that can be accessed via the
* getMasterTreeNode
method.
*
*
* In accessing this method, do not forget to reset the
* globalCounter
as if it reaches a value of
* MAX_RECURSION
*
this method will simply return.
*
*
* @param categoriesArray
* The array of nodes to be added.
* @param headerTreeNode
* The tree node on which to be added.
*
* @see #getMasterHeader()
* @author [email protected]
* @version 1.3
* @since 1.2
*/
private void addNodes(final String[] categoriesArray,
final HeaderTreeNode headerTreeNode) {
if (categoriesArray.length == 0) {
return;
}
if (globalCounter > MAX_RECURSION) {
return;
}
globalCounter++;
// Find the first element
final String firstElement = StringUtils.stripStart(StringUtils.stripEnd(
categoriesArray[0], " "), " ");
// Use the index to know its position
int index = 0;
boolean exists = false;
for (final Enumeration e = extracted(headerTreeNode); e.hasMoreElements()
&& !exists;) {
final String currentElement = e.nextElement().toString();
if (currentElement.equalsIgnoreCase(firstElement)) {
exists = true;
} else {
// If firstElement not current, increment
index++;
}
}
// Does the first element exist?
if (!exists) {
headerTreeNode.add(new HeaderTreeNode(firstElement));
}
// Remove the first element, start again
final String[] temp = (String[]) ArrayUtils.subarray(categoriesArray, 1,
categoriesArray.length);
final HeaderTreeNode nemp = (HeaderTreeNode) headerTreeNode.getChildAt(index);
addNodes(temp, nemp);
}
@SuppressWarnings("unchecked")
private Enumeration extracted(final HeaderTreeNode headerTreeNode) {
return headerTreeNode.children();
}
protected Header getHeader(final TreePath treePath) {
if (!((HeaderTreeNode) treePath.getLastPathComponent()).isLeaf()) {
return Header.ZERO;
}
for(final String headerName : headersMap.keySet()) {
final Prototype proto = headersMap.get(headerName);
final int catLength = proto.getNoOfCategories();
final String[] categories = new String[catLength];
proto.getCategories().toArray(categories);
final Object[] path = treePath.getPath();
int success = path.length - 1;
for (int i = 0; i < path.length; i++) {
try {
if(path[i + 1].toString().equalsIgnoreCase(categories[i])) {
success--;
} else {
i = 32;
}
} catch (final ArrayIndexOutOfBoundsException exp) {
i = 32;
}
}
// We have found the header we were looking for
if(success == 0) {
final int noOfFields = proto.size();
final String[] output = new String[noOfFields];
proto.getPayloads().toArray(output);
final StringBuffer myBuffer = new StringBuffer();
for(final String payload : output) {
myBuffer.append(payload);
myBuffer.append('\n');
}
myBuffer.append('\n');
final String commentL = proto.getComment();
return new Header(noOfFields, myBuffer.toString(), commentL);
}
}
return Header.ZERO;
}
public HeaderTreeNode getMasterTreeNode() {
return myNode;
}
protected void load() {
for (final String hd : headersMap.keySet()) {
final Prototype pt = headersMap.get(hd);
final String [] catArray = new String[pt.getNoOfCategories()];
pt.getCategories().toArray(catArray);
addNodes(catArray, myNode);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy