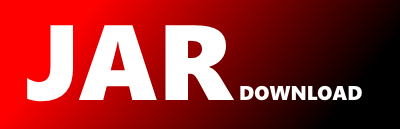
org.owasp.fileio.util.Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-file-io Show documentation
Show all versions of java-file-io Show documentation
The OWASP Java File I/O Security Project provides an easy to use library for validating and sanitizing filenames, directory paths, and uploaded files.
The newest version!
/**
* This file is part of the Open Web Application Security Project (OWASP) Java File IO Security project. For details, please see
* https://www.owasp.org/index.php/OWASP_Java_File_I_O_Security_Project.
*
* Copyright (c) 2014 - The OWASP Foundation
*
* This API is published by OWASP under the Apache 2.0 license. You should read and accept the LICENSE before you use, modify, and/or redistribute this software.
*
* @author Jeff Williams Aspect Security - Original ESAPI author
* @author August Detlefsen CodeMagi - Java File IO Security Project lead
* @created 2014
*/
package org.owasp.fileio.util;
import java.util.HashSet;
import java.util.Set;
/**
* This class provides a number of utility functions.
*
* @author August Detlefsen [augustd at codemagi dot com]
*/
public class Utils {
/**
* Converts an array of chars to a Set of Characters.
*
* @param array the contents of the new Set
* @return a Set containing the elements in the array
*/
public static Set arrayToSet(char... array) {
Set toReturn;
if (array == null) {
return new HashSet();
}
toReturn = new HashSet(array.length);
for (char c : array) {
toReturn.add(c);
}
return toReturn;
}
/**
* Helper function to check if a String is empty
*
* @param input string input value
* @return boolean response if input is empty or not
*/
public static boolean isEmpty(String input) {
return input == null || input.trim().length() == 0;
}
/**
* Helper function to check if a byte array is empty
*
* @param input string input value
* @return boolean response if input is empty or not
*/
public static boolean isEmpty(byte[] input) {
return (input == null || input.length == 0);
}
/**
* Helper function to check if a char array is empty
*
* @param input string input value
* @return boolean response if input is empty or not
*/
public static boolean isEmpty(char[] input) {
return (input == null || input.length == 0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy