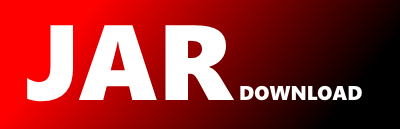
org.pac4j.saml.util.SAML2HttpClientBuilder Maven / Gradle / Ivy
package org.pac4j.saml.util;
import net.shibboleth.shared.httpclient.HttpClientBuilder;
import org.apache.hc.client5.http.auth.CredentialsProvider;
import org.apache.hc.client5.http.classic.HttpClient;
import org.pac4j.core.exception.TechnicalException;
import java.time.Duration;
/**
* This is {@link SAML2HttpClientBuilder}.
*
* @author Misagh Moayyed
*/
public class SAML2HttpClientBuilder {
private Duration connectionTimeout;
private Duration socketTimeout;
private boolean useSystemProperties;
private boolean followRedirects;
private boolean closeConnectionAfterResponse = true;
private int maxConnectionsTotal = 3;
private CredentialsProvider credentialsProvider;
public HttpClient build() {
try {
final var builder = new Pac4jHttpClientBuilder(credentialsProvider);
builder.resetDefaults();
if (this.connectionTimeout != null) {
builder.setConnectionTimeout(this.connectionTimeout);
}
builder.setUseSystemProperties(this.useSystemProperties);
if (this.socketTimeout != null) {
builder.setSocketTimeout(this.socketTimeout);
}
builder.setHttpFollowRedirects(this.followRedirects);
builder.setMaxConnectionsTotal(this.maxConnectionsTotal);
builder.setConnectionCloseAfterResponse(this.closeConnectionAfterResponse);
return builder.buildClient();
} catch (final Exception e) {
throw new TechnicalException(e);
}
}
public Duration getConnectionTimeout() {
return connectionTimeout;
}
public void setConnectionTimeout(final Duration connectionTimeout) {
this.connectionTimeout = connectionTimeout;
}
public Duration getSocketTimeout() {
return socketTimeout;
}
public void setSocketTimeout(final Duration socketTimeout) {
this.socketTimeout = socketTimeout;
}
public boolean isUseSystemProperties() {
return useSystemProperties;
}
public void setUseSystemProperties(final boolean useSystemProperties) {
this.useSystemProperties = useSystemProperties;
}
public boolean isFollowRedirects() {
return followRedirects;
}
public void setFollowRedirects(final boolean followRedirects) {
this.followRedirects = followRedirects;
}
public boolean isCloseConnectionAfterResponse() {
return closeConnectionAfterResponse;
}
public void setCloseConnectionAfterResponse(final boolean closeConnectionAfterResponse) {
this.closeConnectionAfterResponse = closeConnectionAfterResponse;
}
public int getMaxConnectionsTotal() {
return maxConnectionsTotal;
}
public void setMaxConnectionsTotal(final int maxConnectionsTotal) {
this.maxConnectionsTotal = maxConnectionsTotal;
}
public CredentialsProvider getCredentialsProvider() {
return credentialsProvider;
}
public void setCredentialsProvider(final CredentialsProvider credentialsProvider) {
this.credentialsProvider = credentialsProvider;
}
private static class Pac4jHttpClientBuilder extends HttpClientBuilder {
private final CredentialsProvider credentialsProvider;
public Pac4jHttpClientBuilder(final CredentialsProvider credentialsProvider) {
this.credentialsProvider = credentialsProvider;
}
@Override
protected CredentialsProvider buildDefaultCredentialsProvider() {
if (credentialsProvider != null) {
return credentialsProvider;
}
return super.buildDefaultCredentialsProvider();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy