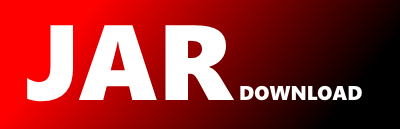
panda.std.Option Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2021 dzikoysk
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package panda.std;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import panda.std.function.ThrowingRunnable;
import panda.std.function.ThrowingSupplier;
import panda.std.reactive.Completable;
import panda.std.stream.PandaStream;
import panda.std.collection.SingletonIterator;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Stream;
public class Option implements Iterable, Serializable {
private static final Option> NONE = new Option<>(null);
protected @Nullable T value;
protected Option(@Nullable T value) {
this.value = value;
}
@SuppressWarnings("unchecked")
public static Option none() {
return (Option) NONE;
}
public static Option blank() {
return Option.of(Blank.BLANK);
}
public static Option<@NotNull T> of(@Nullable T value) {
return value != null ? new Option<>(value) : none();
}
@SuppressWarnings({ "OptionalUsedAsFieldOrParameterType" })
public static Option ofOptional(Optional optional) {
return of(optional.orElse(null));
}
public static Option> withCompleted(T value) {
return Option.of(Completable.completed(value));
}
public static Option when(boolean condition, @Nullable T value) {
return when(condition, () -> value);
}
public static Option when(boolean condition, Supplier<@Nullable T> valueSupplier) {
return condition ? of(valueSupplier.get()) : Option.none();
}
public static Option flatWhen(boolean condition, Option value) {
return condition ? value : none();
}
public static Option flatWhen(boolean condition, Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy