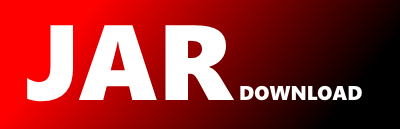
panda.std.reactive.Completable Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2021 dzikoysk
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package panda.std.reactive;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* Observable container for value that could be computed in the future.
* This container is similar to {@link java.util.concurrent.CompletableFuture}, but without the concurrency layer.
*
* @param type of value to store
*/
public class Completable implements Publisher, VALUE> {
private VALUE value;
private boolean ready;
private List> subscribers = new ArrayList<>(3);
public Completable() {
this.value = null;
}
public static Completable completed(VALUE value) {
return new Completable().complete(value);
}
public static Completable create() {
return new Completable<>();
}
public boolean isReady() {
return ready;
}
public boolean isUnprepared() {
return !isReady();
}
public VALUE get() {
if (isReady()) {
return value;
}
throw new IllegalStateException("Option has not been completed");
}
public VALUE orThrow(Supplier exception) throws ERROR {
if (isReady()) {
return value;
}
throw exception.get();
}
@Override
public Completable subscribe(Subscriber super VALUE> subscriber) {
if (isReady()) {
subscriber.onComplete(get());
}
else {
subscribers.add(subscriber);
}
return this;
}
public Completable complete(VALUE value) {
if (isReady()) {
return this;
}
this.ready = true;
this.value = Objects.requireNonNull(value);
for (Subscriber super VALUE> subscriber : subscribers) {
subscriber.onComplete(value);
}
subscribers = null;
return this;
}
public Completable then(Consumer super VALUE> consumer) {
subscribe(consumer::accept);
return this;
}
public Completable thenApply(Function super VALUE, R> map) {
Completable mappedOption = new Completable<>();
subscribe(completedValue -> mappedOption.complete(map.apply(completedValue)));
return mappedOption;
}
public Completable thenCompose(Function super VALUE, ? extends Completable> map) {
Completable mappedOption = new Completable<>();
subscribe(completedValue -> map.apply(completedValue).then(mappedOption::complete));
return mappedOption;
}
public CompletableFuture toFuture() {
CompletableFuture future = new CompletableFuture<>();
then(future::complete);
return future;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy