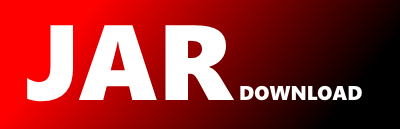
org.partiql.lang.eval.builtins.ToStringExprFunction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partiql-lang-kotlin Show documentation
Show all versions of partiql-lang-kotlin Show documentation
An implementation of PartiQL for the JVM written in Kotlin.
/*
* Copyright 2019 Amazon.com, Inc. or its affiliates. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at:
*
* http://aws.amazon.com/apache2.0/
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific
* language governing permissions and limitations under the License.
*/
package org.partiql.lang.eval.builtins
import org.partiql.lang.errors.ErrorCode
import org.partiql.lang.errors.Property
import org.partiql.lang.errors.PropertyValueMap
import org.partiql.lang.eval.EvaluationException
import org.partiql.lang.eval.EvaluationSession
import org.partiql.lang.eval.ExprFunction
import org.partiql.lang.eval.ExprValue
import org.partiql.lang.eval.ExprValueFactory
import org.partiql.lang.eval.stringValue
import org.partiql.lang.eval.timestampValue
import org.partiql.lang.types.FunctionSignature
import org.partiql.lang.types.StaticType
import org.partiql.lang.util.stringValue
import java.time.DateTimeException
import java.time.format.DateTimeFormatter
import java.time.temporal.UnsupportedTemporalTypeException
class ToStringExprFunction(private val valueFactory: ExprValueFactory) : ExprFunction {
override val signature = FunctionSignature(
name = "to_string",
requiredParameters = listOf(StaticType.TIMESTAMP, StaticType.STRING),
returnType = StaticType.STRING
)
override fun callWithRequired(session: EvaluationSession, required: List): ExprValue {
val pattern = required[1].stringValue()
val formatter: DateTimeFormatter = try {
DateTimeFormatter.ofPattern(pattern)
} catch (ex: IllegalArgumentException) {
errInvalidFormatPattern(pattern, ex)
}
val timestamp = required[0].timestampValue()
val temporalAccessor = TimestampTemporalAccessor(timestamp)
try {
return valueFactory.newString(formatter.format(temporalAccessor))
} catch (ex: UnsupportedTemporalTypeException) {
errInvalidFormatPattern(pattern, ex)
} catch (ex: DateTimeException) {
errInvalidFormatPattern(pattern, ex)
}
}
private fun errInvalidFormatPattern(pattern: String, cause: Exception): Nothing {
val pvmap = PropertyValueMap()
pvmap[Property.TIMESTAMP_FORMAT_PATTERN] = pattern
throw EvaluationException(
"Invalid DateTime format pattern",
ErrorCode.EVALUATOR_INVALID_TIMESTAMP_FORMAT_PATTERN,
pvmap,
cause,
internal = false
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy