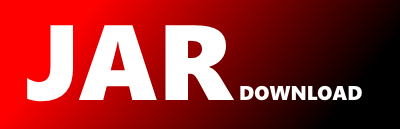
org.partiql.parser.antlr.PartiQLVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of partiql-parser Show documentation
Show all versions of partiql-parser Show documentation
PartiQL's experimental Parser
// Generated from PartiQL.g4 by ANTLR 4.10.1
package org.partiql.parser.antlr;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link PartiQLParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface PartiQLVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link PartiQLParser#root}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRoot(PartiQLParser.RootContext ctx);
/**
* Visit a parse tree produced by the {@code QueryDql}
* labeled alternative in {@link PartiQLParser#statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryDql(PartiQLParser.QueryDqlContext ctx);
/**
* Visit a parse tree produced by the {@code QueryDml}
* labeled alternative in {@link PartiQLParser#statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryDml(PartiQLParser.QueryDmlContext ctx);
/**
* Visit a parse tree produced by the {@code QueryDdl}
* labeled alternative in {@link PartiQLParser#statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryDdl(PartiQLParser.QueryDdlContext ctx);
/**
* Visit a parse tree produced by the {@code QueryExec}
* labeled alternative in {@link PartiQLParser#statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryExec(PartiQLParser.QueryExecContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#explainOption}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExplainOption(PartiQLParser.ExplainOptionContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#asIdent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAsIdent(PartiQLParser.AsIdentContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#atIdent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAtIdent(PartiQLParser.AtIdentContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#byIdent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitByIdent(PartiQLParser.ByIdentContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#symbolPrimitive}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSymbolPrimitive(PartiQLParser.SymbolPrimitiveContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#dql}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDql(PartiQLParser.DqlContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#execCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExecCommand(PartiQLParser.ExecCommandContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#tableName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableName(PartiQLParser.TableNameContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#tableConstraintName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableConstraintName(PartiQLParser.TableConstraintNameContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#columnName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnName(PartiQLParser.ColumnNameContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#columnConstraintName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnConstraintName(PartiQLParser.ColumnConstraintNameContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#ddl}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDdl(PartiQLParser.DdlContext ctx);
/**
* Visit a parse tree produced by the {@code CreateTable}
* labeled alternative in {@link PartiQLParser#createCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCreateTable(PartiQLParser.CreateTableContext ctx);
/**
* Visit a parse tree produced by the {@code CreateIndex}
* labeled alternative in {@link PartiQLParser#createCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCreateIndex(PartiQLParser.CreateIndexContext ctx);
/**
* Visit a parse tree produced by the {@code DropTable}
* labeled alternative in {@link PartiQLParser#dropCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDropTable(PartiQLParser.DropTableContext ctx);
/**
* Visit a parse tree produced by the {@code DropIndex}
* labeled alternative in {@link PartiQLParser#dropCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDropIndex(PartiQLParser.DropIndexContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#tableDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableDef(PartiQLParser.TableDefContext ctx);
/**
* Visit a parse tree produced by the {@code ColumnDeclaration}
* labeled alternative in {@link PartiQLParser#tableDefPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnDeclaration(PartiQLParser.ColumnDeclarationContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#columnConstraint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColumnConstraint(PartiQLParser.ColumnConstraintContext ctx);
/**
* Visit a parse tree produced by the {@code ColConstrNotNull}
* labeled alternative in {@link PartiQLParser#columnConstraintDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColConstrNotNull(PartiQLParser.ColConstrNotNullContext ctx);
/**
* Visit a parse tree produced by the {@code ColConstrNull}
* labeled alternative in {@link PartiQLParser#columnConstraintDef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitColConstrNull(PartiQLParser.ColConstrNullContext ctx);
/**
* Visit a parse tree produced by the {@code DmlBaseWrapper}
* labeled alternative in {@link PartiQLParser#dml}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDmlBaseWrapper(PartiQLParser.DmlBaseWrapperContext ctx);
/**
* Visit a parse tree produced by the {@code DmlDelete}
* labeled alternative in {@link PartiQLParser#dml}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDmlDelete(PartiQLParser.DmlDeleteContext ctx);
/**
* Visit a parse tree produced by the {@code DmlInsertReturning}
* labeled alternative in {@link PartiQLParser#dml}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDmlInsertReturning(PartiQLParser.DmlInsertReturningContext ctx);
/**
* Visit a parse tree produced by the {@code DmlBase}
* labeled alternative in {@link PartiQLParser#dml}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDmlBase(PartiQLParser.DmlBaseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#dmlBaseCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDmlBaseCommand(PartiQLParser.DmlBaseCommandContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#pathSimple}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathSimple(PartiQLParser.PathSimpleContext ctx);
/**
* Visit a parse tree produced by the {@code PathSimpleLiteral}
* labeled alternative in {@link PartiQLParser#pathSimpleSteps}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathSimpleLiteral(PartiQLParser.PathSimpleLiteralContext ctx);
/**
* Visit a parse tree produced by the {@code PathSimpleSymbol}
* labeled alternative in {@link PartiQLParser#pathSimpleSteps}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathSimpleSymbol(PartiQLParser.PathSimpleSymbolContext ctx);
/**
* Visit a parse tree produced by the {@code PathSimpleDotSymbol}
* labeled alternative in {@link PartiQLParser#pathSimpleSteps}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathSimpleDotSymbol(PartiQLParser.PathSimpleDotSymbolContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#replaceCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReplaceCommand(PartiQLParser.ReplaceCommandContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#upsertCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpsertCommand(PartiQLParser.UpsertCommandContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#removeCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRemoveCommand(PartiQLParser.RemoveCommandContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#insertCommandReturning}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertCommandReturning(PartiQLParser.InsertCommandReturningContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#insertStatement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertStatement(PartiQLParser.InsertStatementContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#onConflict}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOnConflict(PartiQLParser.OnConflictContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#insertStatementLegacy}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInsertStatementLegacy(PartiQLParser.InsertStatementLegacyContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#onConflictLegacy}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOnConflictLegacy(PartiQLParser.OnConflictLegacyContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#conflictTarget}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConflictTarget(PartiQLParser.ConflictTargetContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#constraintName}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConstraintName(PartiQLParser.ConstraintNameContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#conflictAction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConflictAction(PartiQLParser.ConflictActionContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#doReplace}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDoReplace(PartiQLParser.DoReplaceContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#doUpdate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDoUpdate(PartiQLParser.DoUpdateContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#updateClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUpdateClause(PartiQLParser.UpdateClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#setCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetCommand(PartiQLParser.SetCommandContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#setAssignment}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetAssignment(PartiQLParser.SetAssignmentContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#deleteCommand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDeleteCommand(PartiQLParser.DeleteCommandContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#returningClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReturningClause(PartiQLParser.ReturningClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#returningColumn}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReturningColumn(PartiQLParser.ReturningColumnContext ctx);
/**
* Visit a parse tree produced by the {@code FromClauseSimpleExplicit}
* labeled alternative in {@link PartiQLParser#fromClauseSimple}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFromClauseSimpleExplicit(PartiQLParser.FromClauseSimpleExplicitContext ctx);
/**
* Visit a parse tree produced by the {@code FromClauseSimpleImplicit}
* labeled alternative in {@link PartiQLParser#fromClauseSimple}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFromClauseSimpleImplicit(PartiQLParser.FromClauseSimpleImplicitContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#whereClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhereClause(PartiQLParser.WhereClauseContext ctx);
/**
* Visit a parse tree produced by the {@code SelectAll}
* labeled alternative in {@link PartiQLParser#selectClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectAll(PartiQLParser.SelectAllContext ctx);
/**
* Visit a parse tree produced by the {@code SelectItems}
* labeled alternative in {@link PartiQLParser#selectClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectItems(PartiQLParser.SelectItemsContext ctx);
/**
* Visit a parse tree produced by the {@code SelectValue}
* labeled alternative in {@link PartiQLParser#selectClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectValue(PartiQLParser.SelectValueContext ctx);
/**
* Visit a parse tree produced by the {@code SelectPivot}
* labeled alternative in {@link PartiQLParser#selectClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectPivot(PartiQLParser.SelectPivotContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#projectionItems}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitProjectionItems(PartiQLParser.ProjectionItemsContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#projectionItem}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitProjectionItem(PartiQLParser.ProjectionItemContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#setQuantifierStrategy}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSetQuantifierStrategy(PartiQLParser.SetQuantifierStrategyContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#letClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLetClause(PartiQLParser.LetClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#letBinding}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLetBinding(PartiQLParser.LetBindingContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#orderByClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrderByClause(PartiQLParser.OrderByClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#orderSortSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOrderSortSpec(PartiQLParser.OrderSortSpecContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#groupClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGroupClause(PartiQLParser.GroupClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#groupAlias}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGroupAlias(PartiQLParser.GroupAliasContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#groupKey}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGroupKey(PartiQLParser.GroupKeyContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#over}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOver(PartiQLParser.OverContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#windowPartitionList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowPartitionList(PartiQLParser.WindowPartitionListContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#windowSortSpecList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWindowSortSpecList(PartiQLParser.WindowSortSpecListContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#havingClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitHavingClause(PartiQLParser.HavingClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#fromClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFromClause(PartiQLParser.FromClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#whereClauseSelect}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWhereClauseSelect(PartiQLParser.WhereClauseSelectContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#offsetByClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOffsetByClause(PartiQLParser.OffsetByClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#limitClause}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLimitClause(PartiQLParser.LimitClauseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#gpmlPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGpmlPattern(PartiQLParser.GpmlPatternContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#gpmlPatternList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGpmlPatternList(PartiQLParser.GpmlPatternListContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#matchPattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMatchPattern(PartiQLParser.MatchPatternContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#graphPart}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGraphPart(PartiQLParser.GraphPartContext ctx);
/**
* Visit a parse tree produced by the {@code SelectorBasic}
* labeled alternative in {@link PartiQLParser#matchSelector}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectorBasic(PartiQLParser.SelectorBasicContext ctx);
/**
* Visit a parse tree produced by the {@code SelectorAny}
* labeled alternative in {@link PartiQLParser#matchSelector}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectorAny(PartiQLParser.SelectorAnyContext ctx);
/**
* Visit a parse tree produced by the {@code SelectorShortest}
* labeled alternative in {@link PartiQLParser#matchSelector}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSelectorShortest(PartiQLParser.SelectorShortestContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#patternPathVariable}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPatternPathVariable(PartiQLParser.PatternPathVariableContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#patternRestrictor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPatternRestrictor(PartiQLParser.PatternRestrictorContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#node}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNode(PartiQLParser.NodeContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeWithSpec}
* labeled alternative in {@link PartiQLParser#edge}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeWithSpec(PartiQLParser.EdgeWithSpecContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeAbbreviated}
* labeled alternative in {@link PartiQLParser#edge}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeAbbreviated(PartiQLParser.EdgeAbbreviatedContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#pattern}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPattern(PartiQLParser.PatternContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#patternQuantifier}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPatternQuantifier(PartiQLParser.PatternQuantifierContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeSpecRight}
* labeled alternative in {@link PartiQLParser#edgeWSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeSpecRight(PartiQLParser.EdgeSpecRightContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeSpecUndirected}
* labeled alternative in {@link PartiQLParser#edgeWSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeSpecUndirected(PartiQLParser.EdgeSpecUndirectedContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeSpecLeft}
* labeled alternative in {@link PartiQLParser#edgeWSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeSpecLeft(PartiQLParser.EdgeSpecLeftContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeSpecUndirectedRight}
* labeled alternative in {@link PartiQLParser#edgeWSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeSpecUndirectedRight(PartiQLParser.EdgeSpecUndirectedRightContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeSpecUndirectedLeft}
* labeled alternative in {@link PartiQLParser#edgeWSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeSpecUndirectedLeft(PartiQLParser.EdgeSpecUndirectedLeftContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeSpecBidirectional}
* labeled alternative in {@link PartiQLParser#edgeWSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeSpecBidirectional(PartiQLParser.EdgeSpecBidirectionalContext ctx);
/**
* Visit a parse tree produced by the {@code EdgeSpecUndirectedBidirectional}
* labeled alternative in {@link PartiQLParser#edgeWSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeSpecUndirectedBidirectional(PartiQLParser.EdgeSpecUndirectedBidirectionalContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#edgeSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeSpec(PartiQLParser.EdgeSpecContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#patternPartLabel}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPatternPartLabel(PartiQLParser.PatternPartLabelContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#edgeAbbrev}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEdgeAbbrev(PartiQLParser.EdgeAbbrevContext ctx);
/**
* Visit a parse tree produced by the {@code TableWrapped}
* labeled alternative in {@link PartiQLParser#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableWrapped(PartiQLParser.TableWrappedContext ctx);
/**
* Visit a parse tree produced by the {@code TableCrossJoin}
* labeled alternative in {@link PartiQLParser#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableCrossJoin(PartiQLParser.TableCrossJoinContext ctx);
/**
* Visit a parse tree produced by the {@code TableQualifiedJoin}
* labeled alternative in {@link PartiQLParser#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableQualifiedJoin(PartiQLParser.TableQualifiedJoinContext ctx);
/**
* Visit a parse tree produced by the {@code TableRefBase}
* labeled alternative in {@link PartiQLParser#tableReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableRefBase(PartiQLParser.TableRefBaseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#tableNonJoin}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableNonJoin(PartiQLParser.TableNonJoinContext ctx);
/**
* Visit a parse tree produced by the {@code TableBaseRefSymbol}
* labeled alternative in {@link PartiQLParser#tableBaseReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableBaseRefSymbol(PartiQLParser.TableBaseRefSymbolContext ctx);
/**
* Visit a parse tree produced by the {@code TableBaseRefClauses}
* labeled alternative in {@link PartiQLParser#tableBaseReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableBaseRefClauses(PartiQLParser.TableBaseRefClausesContext ctx);
/**
* Visit a parse tree produced by the {@code TableBaseRefMatch}
* labeled alternative in {@link PartiQLParser#tableBaseReference}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableBaseRefMatch(PartiQLParser.TableBaseRefMatchContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#tableUnpivot}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTableUnpivot(PartiQLParser.TableUnpivotContext ctx);
/**
* Visit a parse tree produced by the {@code JoinRhsBase}
* labeled alternative in {@link PartiQLParser#joinRhs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJoinRhsBase(PartiQLParser.JoinRhsBaseContext ctx);
/**
* Visit a parse tree produced by the {@code JoinRhsTableJoined}
* labeled alternative in {@link PartiQLParser#joinRhs}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJoinRhsTableJoined(PartiQLParser.JoinRhsTableJoinedContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#joinSpec}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJoinSpec(PartiQLParser.JoinSpecContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#joinType}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitJoinType(PartiQLParser.JoinTypeContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpr(PartiQLParser.ExprContext ctx);
/**
* Visit a parse tree produced by the {@code Intersect}
* labeled alternative in {@link PartiQLParser#exprBagOp}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntersect(PartiQLParser.IntersectContext ctx);
/**
* Visit a parse tree produced by the {@code QueryBase}
* labeled alternative in {@link PartiQLParser#exprBagOp}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitQueryBase(PartiQLParser.QueryBaseContext ctx);
/**
* Visit a parse tree produced by the {@code Except}
* labeled alternative in {@link PartiQLParser#exprBagOp}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExcept(PartiQLParser.ExceptContext ctx);
/**
* Visit a parse tree produced by the {@code Union}
* labeled alternative in {@link PartiQLParser#exprBagOp}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnion(PartiQLParser.UnionContext ctx);
/**
* Visit a parse tree produced by the {@code SfwQuery}
* labeled alternative in {@link PartiQLParser#exprSelect}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSfwQuery(PartiQLParser.SfwQueryContext ctx);
/**
* Visit a parse tree produced by the {@code SfwBase}
* labeled alternative in {@link PartiQLParser#exprSelect}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSfwBase(PartiQLParser.SfwBaseContext ctx);
/**
* Visit a parse tree produced by the {@code Or}
* labeled alternative in {@link PartiQLParser#exprOr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOr(PartiQLParser.OrContext ctx);
/**
* Visit a parse tree produced by the {@code ExprOrBase}
* labeled alternative in {@link PartiQLParser#exprOr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprOrBase(PartiQLParser.ExprOrBaseContext ctx);
/**
* Visit a parse tree produced by the {@code ExprAndBase}
* labeled alternative in {@link PartiQLParser#exprAnd}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprAndBase(PartiQLParser.ExprAndBaseContext ctx);
/**
* Visit a parse tree produced by the {@code And}
* labeled alternative in {@link PartiQLParser#exprAnd}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAnd(PartiQLParser.AndContext ctx);
/**
* Visit a parse tree produced by the {@code Not}
* labeled alternative in {@link PartiQLParser#exprNot}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNot(PartiQLParser.NotContext ctx);
/**
* Visit a parse tree produced by the {@code ExprNotBase}
* labeled alternative in {@link PartiQLParser#exprNot}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprNotBase(PartiQLParser.ExprNotBaseContext ctx);
/**
* Visit a parse tree produced by the {@code PredicateIn}
* labeled alternative in {@link PartiQLParser#exprPredicate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPredicateIn(PartiQLParser.PredicateInContext ctx);
/**
* Visit a parse tree produced by the {@code PredicateBetween}
* labeled alternative in {@link PartiQLParser#exprPredicate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPredicateBetween(PartiQLParser.PredicateBetweenContext ctx);
/**
* Visit a parse tree produced by the {@code PredicateBase}
* labeled alternative in {@link PartiQLParser#exprPredicate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPredicateBase(PartiQLParser.PredicateBaseContext ctx);
/**
* Visit a parse tree produced by the {@code PredicateComparison}
* labeled alternative in {@link PartiQLParser#exprPredicate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPredicateComparison(PartiQLParser.PredicateComparisonContext ctx);
/**
* Visit a parse tree produced by the {@code PredicateIs}
* labeled alternative in {@link PartiQLParser#exprPredicate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPredicateIs(PartiQLParser.PredicateIsContext ctx);
/**
* Visit a parse tree produced by the {@code PredicateLike}
* labeled alternative in {@link PartiQLParser#exprPredicate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPredicateLike(PartiQLParser.PredicateLikeContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#mathOp00}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMathOp00(PartiQLParser.MathOp00Context ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#mathOp01}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMathOp01(PartiQLParser.MathOp01Context ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#mathOp02}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMathOp02(PartiQLParser.MathOp02Context ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#valueExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValueExpr(PartiQLParser.ValueExprContext ctx);
/**
* Visit a parse tree produced by the {@code ExprPrimaryPath}
* labeled alternative in {@link PartiQLParser#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprPrimaryPath(PartiQLParser.ExprPrimaryPathContext ctx);
/**
* Visit a parse tree produced by the {@code ExprPrimaryBase}
* labeled alternative in {@link PartiQLParser#exprPrimary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprPrimaryBase(PartiQLParser.ExprPrimaryBaseContext ctx);
/**
* Visit a parse tree produced by the {@code ExprTermWrappedQuery}
* labeled alternative in {@link PartiQLParser#exprTerm}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprTermWrappedQuery(PartiQLParser.ExprTermWrappedQueryContext ctx);
/**
* Visit a parse tree produced by the {@code ExprTermCurrentUser}
* labeled alternative in {@link PartiQLParser#exprTerm}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprTermCurrentUser(PartiQLParser.ExprTermCurrentUserContext ctx);
/**
* Visit a parse tree produced by the {@code ExprTermBase}
* labeled alternative in {@link PartiQLParser#exprTerm}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprTermBase(PartiQLParser.ExprTermBaseContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#nullIf}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNullIf(PartiQLParser.NullIfContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#coalesce}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCoalesce(PartiQLParser.CoalesceContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#caseExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCaseExpr(PartiQLParser.CaseExprContext ctx);
/**
* Visit a parse tree produced by the {@code values}
* labeled alternative in {@link PartiQLParser#statementstatementstatementstatementcreateCommandcreateCommanddropCommanddropCommandtableDefPartcolumnConstraintDefcolumnConstraintDefdmldmldmldmlpathSimpleStepspathSimpleStepspathSimpleStepsfromClauseSimplefromClauseSimpleselectClauseselectClauseselectClauseselectClausematchSelectormatchSelectormatchSelectoredgeedgeedgeWSpecedgeWSpecedgeWSpecedgeWSpecedgeWSpecedgeWSpecedgeWSpectableReferencetableReferencetableReferencetableReferencetableBaseReferencetableBaseReferencetableBaseReferencejoinRhsjoinRhsexprBagOpexprBagOpexprBagOpexprBagOpexprSelectexprSelectexprOrexprOrexprAndexprAndexprNotexprNotexprPredicateexprPredicateexprPredicateexprPredicateexprPredicateexprPredicateexprPrimaryexprPrimaryexprTermexprTermexprTermaggregateaggregatewindowFunctionfunctionCallfunctionCallpathSteppathSteppathSteppathStepvarRefExprvarRefExprliteralliteralliteralliteralliteralliteralliteralliteralliteralliteraltypetypetypetypetypetype}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValues(PartiQLParser.ValuesContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#valueRow}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValueRow(PartiQLParser.ValueRowContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#valueList}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitValueList(PartiQLParser.ValueListContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#sequenceConstructor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSequenceConstructor(PartiQLParser.SequenceConstructorContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#substring}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSubstring(PartiQLParser.SubstringContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#position}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPosition(PartiQLParser.PositionContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#overlay}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOverlay(PartiQLParser.OverlayContext ctx);
/**
* Visit a parse tree produced by the {@code CountAll}
* labeled alternative in {@link PartiQLParser#aggregate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCountAll(PartiQLParser.CountAllContext ctx);
/**
* Visit a parse tree produced by the {@code AggregateBase}
* labeled alternative in {@link PartiQLParser#aggregate}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAggregateBase(PartiQLParser.AggregateBaseContext ctx);
/**
* Visit a parse tree produced by the {@code LagLeadFunction}
* labeled alternative in {@link PartiQLParser#windowFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLagLeadFunction(PartiQLParser.LagLeadFunctionContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#cast}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCast(PartiQLParser.CastContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#canLosslessCast}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCanLosslessCast(PartiQLParser.CanLosslessCastContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#canCast}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCanCast(PartiQLParser.CanCastContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#extract}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExtract(PartiQLParser.ExtractContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#trimFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrimFunction(PartiQLParser.TrimFunctionContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#dateFunction}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDateFunction(PartiQLParser.DateFunctionContext ctx);
/**
* Visit a parse tree produced by the {@code FunctionCallReserved}
* labeled alternative in {@link PartiQLParser#functionCall}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionCallReserved(PartiQLParser.FunctionCallReservedContext ctx);
/**
* Visit a parse tree produced by the {@code FunctionCallIdent}
* labeled alternative in {@link PartiQLParser#functionCall}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunctionCallIdent(PartiQLParser.FunctionCallIdentContext ctx);
/**
* Visit a parse tree produced by the {@code PathStepIndexExpr}
* labeled alternative in {@link PartiQLParser#pathStep}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathStepIndexExpr(PartiQLParser.PathStepIndexExprContext ctx);
/**
* Visit a parse tree produced by the {@code PathStepIndexAll}
* labeled alternative in {@link PartiQLParser#pathStep}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathStepIndexAll(PartiQLParser.PathStepIndexAllContext ctx);
/**
* Visit a parse tree produced by the {@code PathStepDotExpr}
* labeled alternative in {@link PartiQLParser#pathStep}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathStepDotExpr(PartiQLParser.PathStepDotExprContext ctx);
/**
* Visit a parse tree produced by the {@code PathStepDotAll}
* labeled alternative in {@link PartiQLParser#pathStep}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPathStepDotAll(PartiQLParser.PathStepDotAllContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#exprGraphMatchMany}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprGraphMatchMany(PartiQLParser.ExprGraphMatchManyContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#exprGraphMatchOne}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExprGraphMatchOne(PartiQLParser.ExprGraphMatchOneContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#parameter}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParameter(PartiQLParser.ParameterContext ctx);
/**
* Visit a parse tree produced by the {@code VariableIdentifier}
* labeled alternative in {@link PartiQLParser#varRefExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVariableIdentifier(PartiQLParser.VariableIdentifierContext ctx);
/**
* Visit a parse tree produced by the {@code VariableKeyword}
* labeled alternative in {@link PartiQLParser#varRefExpr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVariableKeyword(PartiQLParser.VariableKeywordContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#nonReservedKeywords}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNonReservedKeywords(PartiQLParser.NonReservedKeywordsContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#collection}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCollection(PartiQLParser.CollectionContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#array}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArray(PartiQLParser.ArrayContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#bag}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBag(PartiQLParser.BagContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#tuple}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTuple(PartiQLParser.TupleContext ctx);
/**
* Visit a parse tree produced by {@link PartiQLParser#pair}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPair(PartiQLParser.PairContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralNull}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralNull(PartiQLParser.LiteralNullContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralMissing}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralMissing(PartiQLParser.LiteralMissingContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralTrue}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralTrue(PartiQLParser.LiteralTrueContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralFalse}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralFalse(PartiQLParser.LiteralFalseContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralString}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralString(PartiQLParser.LiteralStringContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralInteger}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralInteger(PartiQLParser.LiteralIntegerContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralDecimal}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralDecimal(PartiQLParser.LiteralDecimalContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralIon}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralIon(PartiQLParser.LiteralIonContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralDate}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralDate(PartiQLParser.LiteralDateContext ctx);
/**
* Visit a parse tree produced by the {@code LiteralTime}
* labeled alternative in {@link PartiQLParser#literal}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLiteralTime(PartiQLParser.LiteralTimeContext ctx);
/**
* Visit a parse tree produced by the {@code TypeAtomic}
* labeled alternative in {@link PartiQLParser#type}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeAtomic(PartiQLParser.TypeAtomicContext ctx);
/**
* Visit a parse tree produced by the {@code TypeArgSingle}
* labeled alternative in {@link PartiQLParser#type}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeArgSingle(PartiQLParser.TypeArgSingleContext ctx);
/**
* Visit a parse tree produced by the {@code TypeVarChar}
* labeled alternative in {@link PartiQLParser#type}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeVarChar(PartiQLParser.TypeVarCharContext ctx);
/**
* Visit a parse tree produced by the {@code TypeArgDouble}
* labeled alternative in {@link PartiQLParser#type}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeArgDouble(PartiQLParser.TypeArgDoubleContext ctx);
/**
* Visit a parse tree produced by the {@code TypeTimeZone}
* labeled alternative in {@link PartiQLParser#type}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeTimeZone(PartiQLParser.TypeTimeZoneContext ctx);
/**
* Visit a parse tree produced by the {@code TypeCustom}
* labeled alternative in {@link PartiQLParser#type}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTypeCustom(PartiQLParser.TypeCustomContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy