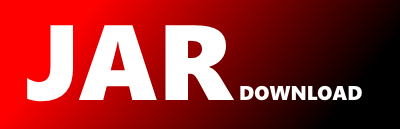
patterntesting.concurrent.junit.RecordedStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of patterntesting-concurrent Show documentation
Show all versions of patterntesting-concurrent Show documentation
PatternTesting Concurrent (patterntesting-concurrent) is a collection
of useful thread aspects. It has support for testing, for
sychnronization and for concurrent programming.
Some of the ideas used in this library comes from reading
Brian Goetz's book "Java Concurrency in Practice".
/*
* $Id: RecordedStatement.java,v 1.8 2010/07/21 16:29:45 oboehm Exp $
*
* Copyright (c) 2010 by Oliver Boehm
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express orimplied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* (c)reated 21.03.2010 by oliver ([email protected])
*/
package patterntesting.concurrent.junit;
import org.apache.commons.logging.*;
import org.junit.runners.model.*;
import patterntesting.runtime.junit.internal.ProfiledStatement;
/**
* @author oliver
* @since 1.0 (21.03.2010)
*/
public final class RecordedStatement extends ProfiledStatement {
private static final Log log = LogFactory.getLog(RecordedStatement.class);
private Throwable thrown;
/**
* The default constructor for this class if the call of the
* frameworkMethod was ok.
*
* @param testClass the test class
* @param frameworkMethod the FrameworkMethod
*/
public RecordedStatement(final TestClass testClass, final FrameworkMethod frameworkMethod) {
super(testClass, frameworkMethod);
}
/**
* The default constructor for this class if the call of the
* frameworkMethod failed with an exception.
*
* @param testClass the test class
* @param frameworkMethod the FrameworkMethod
* @param thrown the exception to be recorded
*/
public RecordedStatement(final TestClass testClass, final FrameworkMethod frameworkMethod,
final Throwable thrown) {
this(testClass, frameworkMethod);
this.thrown = thrown;
}
/**
* If the called test method throws an exception this exception can be set
* here.
*
* @param t the thrown exception
*/
public void setThrown(final Throwable t) {
this.thrown = t;
}
/**
* Plays the recorded action, throwing a {@code Throwable} if anything
* went wrong. Additionally the measured times will be printed to the
* log. As logger we will use the logger of ParallelRunner because this
* is an internal class and the user probably expects this output for
* logger of ParallelRunner.
*
* @throws Throwable the recorded Throwable
*/
@Override
public void evaluate() throws Throwable {
if (this.thrown != null) {
if (log.isTraceEnabled()) {
log.trace("recorded " + this.getMethodName() + " throws "
+ thrown);
}
throw thrown;
}
}
/**
* If the expected exception matches the recorded exception the test is
* marked as "ok" (i.e. the thrown exception is removed from the record).
*
* If the expected exception does not match the same AssertionError as
* in {@link org.junit.internal.runners.statements.ExpectException} is
* stored.
*
* @param expected the expected exception
*/
public void setExpected(final Class extends Throwable> expected) {
if (this.thrown == null) {
this.thrown = new AssertionError("Expected exception: " + expected.getName());
} else {
if (expected.isAssignableFrom(this.thrown.getClass())) {
this.thrown = null;
} else {
this.thrown = new AssertionError(
"Unexpected exception, expected<" + expected.getName()
+ "> but was<"
+ this.thrown.getClass().getName() + ">");
}
}
}
/**
* Returns true if no exception was thrown.
* @return true if recorded statement has no exception.
*/
public boolean success() {
return this.thrown == null;
}
/**
* This is the opposite of {@link #success()}.
* @return true if recorded statement has an exception.
*/
public boolean failed() {
return this.thrown != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy