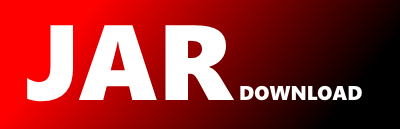
patterntesting.tool.aspectj.Logger Maven / Gradle / Ivy
/*
*========================================================================
*
* Copyright 2005 Vincent Massol & Matt Smith.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express orimplied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*========================================================================
*/
package patterntesting.tool.aspectj;
import java.io.*;
import java.util.*;
import org.apache.commons.io.IOUtils;
import org.aspectj.lang.reflect.SourceLocation;
import org.slf4j.LoggerFactory;
import patterntesting.runtime.io.FileHelper;
/**
* @author Matt Smith
*
* @version $Id: Logger.java,v 1.6 2012/01/02 20:40:10 oboehm Exp $
*/
public final class Logger
{
private static org.slf4j.Logger log = LoggerFactory.getLogger(Logger.class);
/**
* Lists of errors that were logged. Array of {@link AjcFileResult} objects.
*/
private Hashtable errors;
private final Hashtable results = new Hashtable();
private File resultFile;
private static final Logger LOGGER = new Logger();
private Logger() {
this.reset();
try {
OutputStream ostream = new FileOutputStream(this.resultFile);
this.write(ostream);
IOUtils.closeQuietly(ostream);
} catch (FileNotFoundException fnfe) {
log.warn("can't write to " + this.resultFile, fnfe);
}
}
/**
* @return the only instance
*/
public static Logger getInstance() {
return LOGGER;
}
/**
* Reset error table.
*/
public void reset() {
errors = new Hashtable();
if (this.resultFile == null) {
this.setResultFile();
}
this.createResultDir();
}
private void createResultDir() {
File parent = this.getResultFile().getParentFile();
if (!parent.exists()) {
if (parent.mkdir()) {
log.info("directory " + parent + " created for result file");
} else {
log.warn("can't create directory " + parent);
}
}
}
/**
* Sets the result file to a temporary directoy.
* "/patterntesting/patterntesting.xml" as default result file
* is no longer supported.
*/
private void setResultFile() {
File dir = FileHelper.getTmpdir("patterntesting");
if (!dir.mkdirs()) {
log.error(dir + ": mkdir failed");
}
this.setResultFile(new File(dir, "patterntesting.xml"));
}
/**
* Set result file.
* @param file the result fi.e
*/
public void setResultFile(final File file)
{
this.resultFile = file;
}
/**
* @return the result file
*/
public File getResultFile() {
return this.resultFile;
}
/**
* @return the list of errors that were logged as
* a List of AjcResult objects
*/
public Enumeration getErrors()
{
return this.errors.elements();
}
/**
* @return the number of counted errors
*/
public int countErrors() {
int n = 0;
for (Enumeration e = getErrors(); e.hasMoreElements(); e
.nextElement()) {
n++;
}
return n;
}
/**
* @return report results
*/
public Enumeration getReportResults() {
return this.results.elements();
}
/**
*
* @param location the SourceLocation of the error
* @param message the message to log
*/
public synchronized void logPTViolation(final SourceLocation location, final String message)
{
if(location != null)
{
String path = location.getFileName();
AjcFileResult fileResult = errors.get(path);
if(fileResult == null)
{
fileResult = new AjcFileResult(path);
errors.put(path, fileResult);
}
fileResult.addError(new AjcResult(location.getLine(), message));
FileOutputStream out = null;
try
{
out = new FileOutputStream(resultFile);
this.write(out);
out.flush();
}
catch(Exception e)
{
log.warn("can't log to " + resultFile, e);
}
finally
{
IOUtils.closeQuietly(out);
}
}
}
/**
* @param resultStream where to write the result
*/
public void write(final OutputStream resultStream)
{
XMLFormatter formatter = new XMLFormatter();
try
{
resultStream.write(formatter.format(
getReportResults(),
getErrors()).getBytes());
}
catch(IOException ioe)
{
log.warn("can't write to " + resultStream, ioe);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy