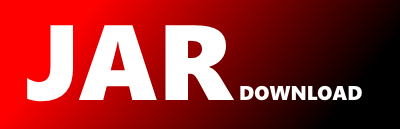
org.paxml.table.AbstractRow Maven / Gradle / Ivy
The newest version!
/**
* This file is part of PaxmlCore.
*
* PaxmlCore is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* PaxmlCore is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with PaxmlCore. If not, see .
*/
package org.paxml.table;
import java.util.AbstractMap;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.paxml.util.RangedIterator;
public abstract class AbstractRow extends AbstractMap implements IRow {
private T table;
@Override
public Object getCellValue(String name) {
ICell cell = getCell(name);
return cell == null ? null : cell.getValue();
}
@Override
public Object getCellValue(int index) {
ICell cell = getCell(index);
return cell == null ? null : cell.getValue();
}
@Override
public Object put(String key, Object value) {
IColumn col = table.getColumn(key);
if (col == null) {
col = table.addColumn(key);
}
int index = col.getIndex();
Object existing = getCellValue(index);
setCellValue(index, value);
return existing;
}
@Override
public Set> entrySet() {
Set> set = new LinkedHashSet>();
for (final IColumn col : table.getColumns()) {
set.add(new Map.Entry() {
@Override
public String getKey() {
return col.getName();
}
@Override
public Object getValue() {
return getCellValue(col.getIndex());
}
@Override
public Object setValue(Object value) {
Object existing = getValue();
setCellValue(col.getIndex(), value);
return existing;
}
});
}
return set;
}
@Override
public Iterator iterator() {
return getCells();
}
@Override
public ICell getCell(String name) {
return getCell(table.getColumn(name).getIndex());
}
@Override
public void setCellValue(String name, Object value) {
setCellValue(table.getColumn(name).getIndex(), value);
}
@Override
public void setCellValues(Map values) {
for (Map.Entry entry : values.entrySet()) {
setCellValue(entry.getKey(), entry.getValue());
}
}
@Override
public void setCellValues(int from, int to, Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy