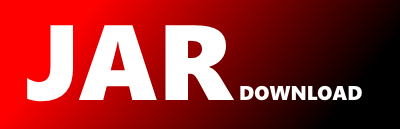
org.paxml.util.PaxmlUtils Maven / Gradle / Ivy
The newest version!
/**
* This file is part of PaxmlCore.
*
* PaxmlCore is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* PaxmlCore is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with PaxmlCore. If not, see .
*/
package org.paxml.util;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.nio.file.Paths;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Map;
import java.util.Properties;
import java.util.regex.Pattern;
import org.apache.commons.io.IOUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.paxml.core.PaxmlParseException;
import org.paxml.core.PaxmlRuntimeException;
import org.paxml.launch.LaunchPoint;
import org.springframework.core.io.DefaultResourceLoader;
import org.springframework.core.io.Resource;
import org.springframework.dao.DataAccessException;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.jdbc.core.ResultSetExtractor;
import com.thoughtworks.xstream.core.util.Base64Encoder;
public class PaxmlUtils {
private static final Log log = LogFactory.getLog(PaxmlUtils.class);
public static final String PAXML_HOME_ENV_KEY = "PAXML_HOME";
public static File getPaxmlHome(boolean assert_PAXML_HOME) {
String paxmlHome = getSystemProperty(PAXML_HOME_ENV_KEY);
if (paxmlHome == null) {
if (assert_PAXML_HOME) {
throw new PaxmlRuntimeException("System environment variable 'PAXML_HOME' not set!");
} else {
return null;
}
}
return new File(paxmlHome);
}
/**
* Get resource from path.
*
* @param path
* if path has prefix, the path will be directly used; if not,
* the path will be treated as a relative path based on the
* "base" resource parameter.
* @param base
* the base resource when path has no prefix. If null given and
* base resource has no prefix, then the path is assumed to be a
* file system resource if it exists, and if it doesn't exist, it
* will be assumed as a classpath resource.
* @return the Spring resource.
*/
public static Resource getResource(String path, Resource base) {
final String filePrefix = "file:";
final String classpathPrefix = "classpath:";
if (!path.startsWith(filePrefix) && !path.startsWith(classpathPrefix)) {
if (base == null) {
File file = getFile(path);
if (file.isFile()) {
path = filePrefix + file.getAbsolutePath();
} else {
// assume to be a file
path = classpathPrefix + path;
}
} else {
try {
path = base.createRelative(path).getURI().toString();
} catch (IOException e) {
throw new PaxmlParseException("Cannot create relative path '" + path + "' from base resource: "
+ base + ", because: " + e.getMessage());
}
}
}
if (log.isDebugEnabled()) {
log.debug("Taking resource from computed path: " + path);
}
return new DefaultResourceLoader().getResource(path);
}
public static Resource getResource(String path) {
return getResource(path, null);
}
public static Resource getResource(File file) {
return getResource(file.getAbsolutePath());
}
/**
* Create a file object.
*
* @param pathWithoutPrefix
* the path without spring resource prefix.
* @return if the given path is relative, try the following locations in
* order: - current working dir - user.home dir - dir pointed by
* PAXML_HOME system property - if file not found in all above
* locations, then return {@code}new File(pathWithoutPrefix);{@code}
*/
public static File getFile(String pathWithoutPrefix) {
File file = new File(pathWithoutPrefix);
if (!file.isAbsolute()) {
file = getFileUnderCurrentDir(pathWithoutPrefix);
if (!file.exists()) {
file = getFileUnderUserHome(pathWithoutPrefix);
}
if (!file.exists()) {
File f = getFileUnderPaxmlHome(pathWithoutPrefix, false);
if (f != null && f.exists()) {
file = f;
}
}
}
return file;
}
public static File getFileUnderPaxmlHome(String file, boolean assert_PAXML_HOME) {
File home = getPaxmlHome(assert_PAXML_HOME);
if (home == null) {
return null;
}
return new File(home, file);
}
public static File getFileUnderCurrentDir(String file) {
return new File(new File("."), file);
}
public static File getFileUnderUserHome(String file) {
File userHomeDir = new File(System.getProperty("user.home"));
return new File(userHomeDir, file);
}
/**
* Trim the property names and values and return in a new Properties object.
*
* @param props
* properties
* @return the new Properties object contained the trimmed names and values.
*/
public static Properties trimProperties(Properties props) {
Properties result = new Properties();
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy