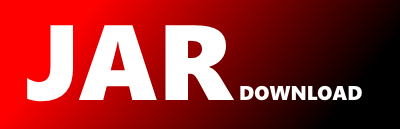
org.pepsoft.worldpainter.ColourEditor Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package org.pepsoft.worldpainter;
import java.awt.Color;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import javax.swing.JColorChooser;
/**
*
* @author pepijn
*/
public class ColourEditor extends javax.swing.JPanel implements MouseListener {
/**
* Creates new form ColourEditor
*/
public ColourEditor() {
initComponents();
setLabelColour();
}
public int getColour() {
return colour;
}
public void setColour(int colour) {
this.colour = colour;
setLabelColour();
}
@Override
public void setEnabled(boolean enabled) {
super.setEnabled(enabled);
labelColour.setOpaque(enabled);
buttonSelectColour.setEnabled(enabled);
}
@Override
public int getBaseline(int width, int height) {
return labelColour.getBaseline(width, height);
}
// MouseListener
@Override
public void mouseClicked(MouseEvent e) {
if (isEnabled()) {
pickColour();
}
}
@Override public void mousePressed(MouseEvent e) {}
@Override public void mouseReleased(MouseEvent e) {}
@Override public void mouseEntered(MouseEvent e) {}
@Override public void mouseExited(MouseEvent e) {}
private void setLabelColour() {
labelColour.setBackground(new Color(colour));
}
private void pickColour() {
Color pick = JColorChooser.showDialog(this, "Select Colour", new Color(colour));
if (pick != null) {
int newColour = pick.getRGB();
if (newColour != colour) {
int oldColour = colour;
colour = pick.getRGB();
setLabelColour();
firePropertyChange("colour", oldColour, newColour);
}
}
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
labelColour = new javax.swing.JLabel();
buttonSelectColour = new javax.swing.JButton();
labelColour.setBackground(new java.awt.Color(255, 128, 0));
labelColour.setLabelFor(buttonSelectColour);
labelColour.setText(" ");
labelColour.setToolTipText("Click to select a colour");
labelColour.setBorder(javax.swing.BorderFactory.createLineBorder(new java.awt.Color(0, 0, 0)));
labelColour.setOpaque(true);
buttonSelectColour.setText("...");
buttonSelectColour.setToolTipText("Click to select a colour");
buttonSelectColour.addActionListener(this::buttonSelectColourActionPerformed);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(labelColour)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(buttonSelectColour))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(labelColour)
.addComponent(buttonSelectColour))
);
}// //GEN-END:initComponents
private void buttonSelectColourActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_buttonSelectColourActionPerformed
pickColour();
}//GEN-LAST:event_buttonSelectColourActionPerformed
private int colour = Color.ORANGE.getRGB();
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton buttonSelectColour;
private javax.swing.JLabel labelColour;
// End of variables declaration//GEN-END:variables
private static final long serialVersionUID = 1L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy