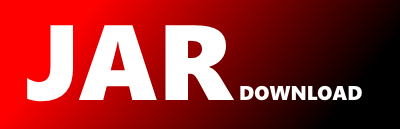
org.pepsoft.worldpainter.ExportProgressDialog Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
/*
* ExportWorldDialog.java
*
* Created on Mar 29, 2011, 5:09:50 PM
*/
package org.pepsoft.worldpainter;
import org.pepsoft.minecraft.ChunkFactory;
import org.pepsoft.util.*;
import org.pepsoft.util.Version;
import org.pepsoft.util.ProgressReceiver.OperationCancelled;
import org.pepsoft.util.swing.ProgressTask;
import org.pepsoft.worldpainter.exporting.WorldExportSettings;
import org.pepsoft.worldpainter.exporting.WorldExporter;
import org.pepsoft.worldpainter.plugins.PlatformManager;
import org.pepsoft.worldpainter.util.FileInUseException;
import javax.swing.*;
import java.awt.*;
import java.awt.event.WindowEvent;
import java.awt.event.WindowListener;
import java.io.File;
import java.io.IOException;
import java.text.NumberFormat;
import java.util.Map;
import static org.pepsoft.minecraft.Constants.*;
import static org.pepsoft.util.ExceptionUtils.chainContains;
import static org.pepsoft.worldpainter.Constants.V_1_17;
import static org.pepsoft.worldpainter.DefaultPlugin.*;
/**
*
* @author pepijn
*/
public class ExportProgressDialog extends MultiProgressDialog
© 2015 - 2025 Weber Informatics LLC | Privacy Policy