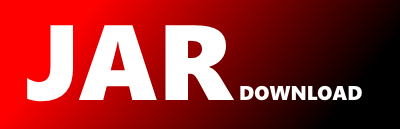
org.pepsoft.worldpainter.MerchDialog Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
/*
* DonationDialog.java
*
* Created on 5-nov-2011, 17:24:59
*/
package org.pepsoft.worldpainter;
import org.pepsoft.util.DesktopUtils;
import org.pepsoft.worldpainter.vo.EventVO;
import javax.swing.*;
import java.awt.*;
import java.net.MalformedURLException;
import java.net.URL;
import static org.pepsoft.util.swing.MessageUtils.showInfo;
/**
*
* @author pepijn
*/
@SuppressWarnings({"ConstantConditions", "Convert2Lambda", "Anonymous2MethodRef", "unused", "FieldCanBeLocal"}) // Managed by NetBeans
public final class MerchDialog extends WorldPainterDialog {
/** Creates new form DonationDialog */
public MerchDialog(Window parent) {
super(parent);
config = Configuration.getInstance();
initComponents();
// Fix JTextArea font, which uses a butt ugly non-proportional font by default on Windows
jTextArea1.setFont(UIManager.getFont("TextField.font").deriveFont(UIManager.getFont("TextField.font").getSize() + 6f));
rootPane.setDefaultButton(buttonMerchStore);
pack();
}
public static void maybeShowMerchDialog(Window parent) {
final Configuration config = Configuration.getInstance();
// Show again every so often
if ((config.getMerchStoreDialogDisplayed() == null) || (config.getLaunchCount() - config.getMerchStoreDialogDisplayed() > 40)) {
config.setMerchStoreDialogDisplayed(config.getLaunchCount());
MerchDialog dialog = new MerchDialog(parent);
dialog.setLocationRelativeTo(parent);
dialog.setVisible(true);
}
}
private void noThanks() {
showInfo(this, "Alright, no problem. You can access the merch store\nlater from the Help menu.", "No Problem");
ok();
}
private void openMerchStore() {
try {
DesktopUtils.open(new URL("https://www.worldpainter.store/"));
config.logEvent(new EventVO(Constants.EVENT_KEY_MERCH_STORE_OPENED).addTimestamp());
ok();
} catch (MalformedURLException e) {
throw new RuntimeException(e);
}
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jTextArea1 = new javax.swing.JTextArea();
buttonNoThanks = new javax.swing.JButton();
buttonMerchStore = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
setTitle("WorldPainter Has A Merch Store");
setResizable(false);
jLabel1.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel1.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/pepsoft/worldpainter/resources/about.png"))); // NOI18N
jLabel1.setBorder(javax.swing.BorderFactory.createBevelBorder(javax.swing.border.BevelBorder.LOWERED));
jTextArea1.setEditable(false);
jTextArea1.setColumns(20);
jTextArea1.setFont(jTextArea1.getFont().deriveFont(jTextArea1.getFont().getSize()+6f));
jTextArea1.setLineWrap(true);
jTextArea1.setText("WorldPainter has a merch store! Get your WorldPainter paraphernalia, be the envy of all your friends and support the development of WorldPainter.");
jTextArea1.setWrapStyleWord(true);
jTextArea1.setOpaque(false);
buttonNoThanks.setFont(buttonNoThanks.getFont().deriveFont(buttonNoThanks.getFont().getSize()+3f));
buttonNoThanks.setMnemonic('n');
buttonNoThanks.setText("No thank you");
buttonNoThanks.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
buttonNoThanksActionPerformed(evt);
}
});
buttonMerchStore.setFont(buttonMerchStore.getFont().deriveFont(buttonMerchStore.getFont().getSize()+3f));
buttonMerchStore.setText("Open merch store");
buttonMerchStore.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
buttonMerchStoreActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createSequentialGroup()
.addComponent(buttonMerchStore)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(buttonNoThanks))
.addComponent(jTextArea1))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel1)
.addGap(18, 18, 18)
.addComponent(jTextArea1)
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(buttonNoThanks)
.addComponent(buttonMerchStore))
.addContainerGap())
);
pack();
}// //GEN-END:initComponents
private void buttonNoThanksActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_buttonNoThanksActionPerformed
noThanks();
}//GEN-LAST:event_buttonNoThanksActionPerformed
private void buttonMerchStoreActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_buttonMerchStoreActionPerformed
openMerchStore();
}//GEN-LAST:event_buttonMerchStoreActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton buttonMerchStore;
private javax.swing.JButton buttonNoThanks;
private javax.swing.JLabel jLabel1;
private javax.swing.JTextArea jTextArea1;
// End of variables declaration//GEN-END:variables
private final Configuration config;
private static final long serialVersionUID = 1L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy