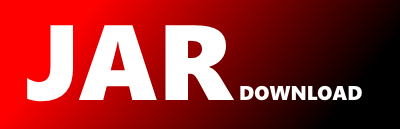
org.pepsoft.worldpainter.NoiseSettingsDialog Maven / Gradle / Ivy
/*
* Click nbfs://nbhost/SystemFileSystem/Templates/Licenses/license-default.txt to change this license
* Click nbfs://nbhost/SystemFileSystem/Templates/GUIForms/JDialog.java to edit this template
*/
package org.pepsoft.worldpainter;
import org.pepsoft.worldpainter.heightMaps.NoiseHeightMap;
import javax.swing.*;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import java.awt.*;
import java.awt.event.ComponentEvent;
import java.awt.image.BufferedImage;
import static org.pepsoft.util.MathUtils.clamp;
/**
*
* @author Pepijn
*/
public class NoiseSettingsDialog extends WorldPainterDialog implements ChangeListener {
/**
* Creates a new form NoiseSettingsDialog, with the preview based on percentages with a fixed base value that may be
* lowered or raised up to the specified range and is then restricted to be between 0% and 100%.
*/
public NoiseSettingsDialog(Window parent, NoiseSettings noiseSettings, int baseValue) {
super(parent);
this.baseValue = baseValue;
initComponents();
noiseSettingsEditor1.setNoiseSettings(noiseSettings);
noiseSettingsEditor1.addChangeListener(this);
labelPreview.addComponentListener(new java.awt.event.ComponentAdapter() {
@Override
public void componentResized(ComponentEvent e) {
updatePreview();
}
});
scaleToUI();
pack();
setLocationRelativeTo(parent);
updatePreview();
}
public NoiseSettings getNoiseSettings() {
return noiseSettingsEditor1.getNoiseSettings();
}
// ChangeListener
@Override
public void stateChanged(ChangeEvent e) {
updatePreview();
}
private void clear() {
noiseSettingsEditor1.setNoiseSettings(new NoiseSettings(getNoiseSettings().getSeed(), 0, 0, 1.0f));
}
private void updatePreview() {
// TODO offer other preview modes (such as a raw one)
final Insets insets = labelPreview.getInsets();
final BufferedImage image = GraphicsEnvironment.getLocalGraphicsEnvironment().getDefaultScreenDevice().getDefaultConfiguration().createCompatibleImage(
labelPreview.getWidth() - insets.left - insets.right,
labelPreview.getHeight() - insets.top - insets.bottom);
final NoiseHeightMap noiseHeightMap = new NoiseHeightMap(noiseSettingsEditor1.getNoiseSettings(), 0);
for (int x = 0; x < image.getWidth(); x++) {
for (int y = 0; y < image.getHeight(); y++) {
final int value = (int) clamp(0L, Math.round((baseValue + noiseHeightMap.getValue(x, y) - noiseHeightMap.getHeight() / 2) * 2.55), 255L);
image.setRGB(x, y, 0xff000000 | (value << 16) | (value << 8) | value);
}
}
labelPreview.setIcon(new ImageIcon(image));
}
/**
* This method is called from within the constructor to initialize the form. WARNING: Do NOT modify this code. The
* content of this method is always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
noiseSettingsEditor1 = new org.pepsoft.worldpainter.NoiseSettingsEditor();
labelPreview = new javax.swing.JLabel();
buttonCancel = new javax.swing.JButton();
buttonOk = new javax.swing.JButton();
buttonClear = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.DISPOSE_ON_CLOSE);
setTitle("Adjust Noise Settings");
setResizable(false);
jLabel1.setText("Adjust the noise settings:");
labelPreview.setBorder(javax.swing.BorderFactory.createBevelBorder(javax.swing.border.BevelBorder.LOWERED));
buttonCancel.setText("Cancel");
buttonCancel.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
buttonCancelActionPerformed(evt);
}
});
buttonOk.setText("OK");
buttonOk.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
buttonOkActionPerformed(evt);
}
});
buttonClear.setText("Clear");
buttonClear.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
buttonClearActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(labelPreview, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel1)
.addComponent(noiseSettingsEditor1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(0, 0, Short.MAX_VALUE))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addComponent(buttonClear)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(buttonOk)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(buttonCancel)))
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(noiseSettingsEditor1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(labelPreview, javax.swing.GroupLayout.DEFAULT_SIZE, 119, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(buttonCancel)
.addComponent(buttonOk)
.addComponent(buttonClear))
.addContainerGap())
);
pack();
}// //GEN-END:initComponents
private void buttonCancelActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_buttonCancelActionPerformed
cancel();
}//GEN-LAST:event_buttonCancelActionPerformed
private void buttonOkActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_buttonOkActionPerformed
ok();
}//GEN-LAST:event_buttonOkActionPerformed
private void buttonClearActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_buttonClearActionPerformed
clear();
}//GEN-LAST:event_buttonClearActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton buttonCancel;
private javax.swing.JButton buttonClear;
private javax.swing.JButton buttonOk;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel labelPreview;
private org.pepsoft.worldpainter.NoiseSettingsEditor noiseSettingsEditor1;
// End of variables declaration//GEN-END:variables
private final int baseValue;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy