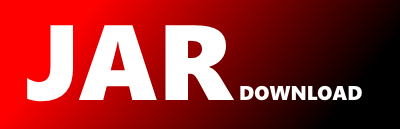
org.pepsoft.util.swing.ProgressViewer Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
/*
* ProgressComponent.java
*
* Created on Apr 19, 2012, 7:02:06 PM
*/
package org.pepsoft.util.swing;
import org.pepsoft.util.ProgressReceiver;
import org.pepsoft.util.SubProgressReceiver;
import org.pepsoft.util.mdc.MDCCapturingRuntimeException;
import static org.pepsoft.util.AwtUtils.doOnEventThreadAndWait;
/**
* A component which can show the progress of a {@link SubProgressReceiver} by
* attaching to it as a listener.
*
* @author pepijn
*/
final class ProgressViewer extends javax.swing.JPanel implements ProgressReceiver {
@SuppressWarnings("LeakingThisInConstructor") // Construction done at that point
ProgressViewer(SubProgressReceiver subProgressReceiver) {
this.subProgressReceiver = subProgressReceiver;
initComponents();
subProgressReceiver.addListener(this);
String lastMessage = subProgressReceiver.getLastMessage();
if (lastMessage != null) {
try {
setMessage(lastMessage);
} catch (OperationCancelled operationCancelled) {
throw new MDCCapturingRuntimeException("Operation cancelled");
}
}
}
ProgressViewer() {
subProgressReceiver = null;
initComponents();
}
SubProgressReceiver getSubProgressReceiver() {
return subProgressReceiver;
}
// ProgressReceiver
@Override
public void setProgress(final float progress) {
doOnEventThreadAndWait(() -> {
if (jProgressBar1.isIndeterminate()) {
jProgressBar1.setIndeterminate(false);
}
jProgressBar1.setValue(Math.round(progress * 100f));
});
}
@Override
public void exceptionThrown(final Throwable exception) {
doOnEventThreadAndWait(() -> {
if (jProgressBar1.isIndeterminate()) {
jProgressBar1.setIndeterminate(false);
}
});
}
@Override
public void done() {
doOnEventThreadAndWait(() -> {
if (jProgressBar1.isIndeterminate()) {
jProgressBar1.setIndeterminate(false);
}
jProgressBar1.setValue(100);
});
}
@Override
public void setMessage(final String message) throws OperationCancelled {
doOnEventThreadAndWait(() -> jLabel1.setText(message));
}
@Override
public void checkForCancellation() {
// Do nothing
}
@Override
public void reset() {
doOnEventThreadAndWait(() -> jProgressBar1.setIndeterminate(true));
}
@Override
public void subProgressStarted(SubProgressReceiver subProgressReceiver) {
// Do nothing
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jProgressBar1 = new javax.swing.JProgressBar();
jLabel1.setText(" ");
jProgressBar1.setIndeterminate(true);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jProgressBar1, javax.swing.GroupLayout.DEFAULT_SIZE, 318, Short.MAX_VALUE)
.addComponent(jLabel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jProgressBar1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap())
);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLabel jLabel1;
private javax.swing.JProgressBar jProgressBar1;
// End of variables declaration//GEN-END:variables
private final SubProgressReceiver subProgressReceiver;
private static final long serialVersionUID = 1L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy