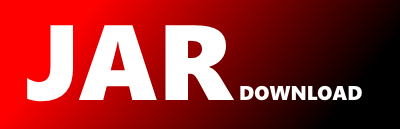
org.pepsoft.util.GUIUtils Maven / Gradle / Ivy
The newest version!
package org.pepsoft.util;
import org.pepsoft.worldpainter.Version;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.swing.*;
import javax.swing.plaf.FontUIResource;
import javax.swing.plaf.InsetsUIResource;
import java.awt.*;
import java.awt.geom.AffineTransform;
import java.awt.image.AffineTransformOp;
import java.awt.image.BufferedImage;
import java.awt.image.BufferedImageOp;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.prefs.Preferences;
import static java.awt.Image.SCALE_SMOOTH;
import static java.awt.RenderingHints.*;
import static java.awt.image.AffineTransformOp.TYPE_BICUBIC;
import static java.awt.image.AffineTransformOp.TYPE_NEAREST_NEIGHBOR;
import static java.awt.image.BufferedImage.TYPE_INT_ARGB;
import static java.lang.Math.round;
/**
* Created by pepijn on 13-Jan-17.
*/
public class GUIUtils {
/**
* Scale an image according to {@link #UI_SCALE}. Nearest neighbour scaling
* is used, in other words no smoothing or interpolation is applied.
*
* @param image The image to scale.
* @return The original image if {@code UI_SCALE} is 1, or an
* appropriately scaled copy otherwise.
*/
public static BufferedImage scaleToUI(Image image) {
return scaleToUI(image, false);
}
/**
* Scale an image according to {@link #UI_SCALE_FLOAT} or {@link #UI_SCALE}.
*
* @param image The image to scale.
* @param smooth Whether to do smooth scaling. When this is {@code true},
* the image is scaled to the floating point UI scale using
* bicubic scaling. When it is {@code false}, it is scaled to
* the UI scale rounded to the nearest integer, using nearest
* neighbour scaling.
* @return The original image if {@code UI_SCALE} is 1, or an
* appropriately scaled copy otherwise.
*/
public static BufferedImage scaleToUI(Image image, boolean smooth) {
if (((UI_SCALE_FLOAT == 1.0f) || ((! smooth) && (UI_SCALE == 1))) && (image instanceof BufferedImage)) {
return (BufferedImage) image;
} else if (image instanceof BufferedImage) {
BufferedImageOp op;
if (smooth) {
op = new AffineTransformOp(AffineTransform.getScaleInstance(getUIScale(), getUIScale()), TYPE_BICUBIC);
} else {
op = new AffineTransformOp(AffineTransform.getScaleInstance(getUIScaleInt(), getUIScaleInt()), TYPE_NEAREST_NEIGHBOR);
}
return op.filter((BufferedImage) image, null);
} else {
BufferedImage scaledImage;
if (smooth) {
scaledImage = new BufferedImage(round(image.getWidth(null) * getUIScale()), round(image.getHeight(null) * getUIScale()), TYPE_INT_ARGB);
Graphics2D g2 = scaledImage.createGraphics();
try {
g2.setRenderingHint(KEY_INTERPOLATION, VALUE_INTERPOLATION_BICUBIC);
g2.drawImage(image, AffineTransform.getScaleInstance(getUIScale(), getUIScale()), null);
} finally {
g2.dispose();
}
} else {
scaledImage = new BufferedImage(image.getWidth(null) * getUIScaleInt(), image.getHeight(null) * getUIScaleInt(), TYPE_INT_ARGB);
Graphics2D g2 = scaledImage.createGraphics();
try {
g2.setRenderingHint(KEY_INTERPOLATION, VALUE_INTERPOLATION_NEAREST_NEIGHBOR);
g2.drawImage(image, AffineTransform.getScaleInstance(getUIScaleInt(), getUIScaleInt()), null);
} finally {
g2.dispose();
}
}
return scaledImage;
}
}
public static void scaleToUI(Container container) {
if (UI_SCALE_FLOAT == 1.0f) {
return;
}
for (Component component: container.getComponents()) {
if (component instanceof JTable) {
JTable table = (JTable) component;
table.setRowHeight(Math.round(table.getRowHeight() * UI_SCALE_FLOAT));
table.setRowMargin(Math.round(table.getRowMargin() * UI_SCALE_FLOAT));
} else if (component instanceof JTextArea) {
component.setFont(UIManager.getFont("TextField.font"));
} else if (component instanceof Container) {
scaleToUI((Container) component);
}
}
}
public static void scaleWindow(Window window) {
final GraphicsConfiguration gc = window.getGraphicsConfiguration();
final Rectangle screenBounds = gc.getBounds();
final Insets insets = Toolkit.getDefaultToolkit().getScreenInsets(gc);
window.setSize(Math.min(Math.round(window.getWidth() * UI_SCALE_FLOAT), screenBounds.width - insets.left - insets.right),
Math.min(Math.round(window.getHeight() * UI_SCALE_FLOAT), screenBounds.height - insets.top - insets.bottom));
}
/**
* Adjusts the {@link UIManager} defaults to show the Java 2D UI at the
* specified scale.
*/
public static void scaleLookAndFeel(float scale) {
if (scale == 1.0f) {
return;
}
Set unknownValueTypesEncountered = new HashSet<>();
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy