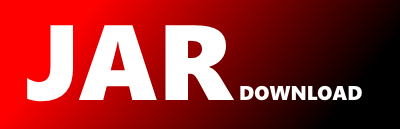
org.pepstock.charba.client.utils.JSON Maven / Gradle / Ivy
/**
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package org.pepstock.charba.client.utils;
import java.util.HashSet;
import java.util.Locale;
import java.util.Set;
import org.pepstock.charba.client.commons.Array;
import org.pepstock.charba.client.commons.Constants;
import org.pepstock.charba.client.commons.JsHelper;
import org.pepstock.charba.client.commons.NativeObject;
import org.pepstock.charba.client.commons.ObjectType;
import org.pepstock.charba.client.dom.BaseAttribute;
import org.pepstock.charba.client.dom.BaseElement;
import org.pepstock.charba.client.dom.NamedNodeMap;
import org.pepstock.charba.client.dom.enums.NodeType;
import jsinterop.annotations.JsFunction;
import jsinterop.annotations.JsMethod;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsPackage;
import jsinterop.annotations.JsType;
/**
* This is the wrapper to JSON java script object.
* The JSON object contains methods for parsing JSON and converting values to JSON.
*
* @author Andrea "Stock" Stocchero
*/
@JsType(isNative = true, namespace = JsPackage.GLOBAL)
public final class JSON {
/**
* A function that alters the behavior of the stringification process. If this value is null or not provided, all properties of the object are included in the resulting JSON
* string.
* Must be an interface with only 1 method.
*
* @author Andrea "Stock" Stocchero
*/
@JsFunction
public interface Replacer {
/**
* Method of function to alter the behavior of the stringification process.
*
* @param key property key of object.
* @param value object related to the key.
* @return the value to show in the string.
*/
Object call(String key, Object value);
}
/**
* To avoid any instantiation
*/
private JSON() {
// do nothing
}
/**
* Converts a JavaScript object or value to a JSON string, optionally replacing values if a replacer function is specified or optionally including only the specified properties
* if a replacer array is specified.
* it can throw an exception, TypeError ("cyclic object value") exception when a circular reference is found.
*
* @param obj The value to convert to a JSON string.
* @param function A function that alters the behavior of the stringification process, or an array of String and Number objects that serve as a whitelist for
* selecting/filtering the properties of the value object to be included in the JSON string. If this value is null or not provided, all properties of the object are
* included in the resulting JSON string.
* @param spaces it indicates the number of space characters to use as white space; this number is capped at 10 (if it is greater, the value is just 10). Values less than 1
* indicate that no space should be used.
* @return A JSON string representing the given value.
*/
static native String stringify(Object obj, Replacer function, int spaces);
/**
* Parses a JSON string, constructing the JavaScript value or object described by the string.
*
* @param text the string to parse as JSON.
* @return the object corresponding to the given JSON text.
*/
@JsMethod(name = "parse")
private static native NativeObject parseToNativeObject(String text);
/**
* Parses a JSON string, constructing the JavaScript array described by the string.
*
* @param text the string to parse as JSON.
* @param type of native array
* @return the array corresponding to the given JSON text.
*/
@JsMethod(name = "parse")
private static native T parseToArray(String text);
/**
* Parses a JSON string, constructing the JavaScript value or object described by the string.
*
* @param text the string to parse as JSON.
* @return the object corresponding to the given JSON text.
*/
@JsOverlay
public static NativeObject parseForObject(String text) {
// checks text argument is consistent
if (text != null && text.trim().startsWith(Constants.OPEN_BRACE)) {
return parseToNativeObject(text);
}
// if here, the argument is not consistent to parse to a object
// then returns null
return null;
}
/**
* Parses a JSON string, constructing the JavaScript array described by the string.
*
* @param text the string to parse as JSON.
* @param type of native array
* @return the array corresponding to the given JSON text.
*/
@JsOverlay
public static T parseForArray(String text) {
// checks text argument is consistent
if (text != null && text.trim().startsWith(Constants.OPEN_SQUARE_BRACKET)) {
return parseToArray(text);
}
// if here, the argument is not consistent to parse to a object
// then returns null
return null;
}
/**
* Converts a JavaScript object or value to a JSON string. By default, the space value is set to -1 that no space should be used.
*
* @param obj The value to convert to a JSON string.
* @return A JSON string representing the given value.
*/
@JsOverlay
public static String stringify(Object obj) {
// checks if object id consistent
if (obj != null) {
// format in JSON
return stringify(obj, null, -1);
}
// if here, the argument is null
// then returns an empty JSON
return JSONReplacerConstants.EMPTY_JSON_OBJECT;
}
/**
* Converts a JavaScript object or value to a JSON string.
*
* @param obj The value to convert to a JSON string.
* @param spaces it indicates the number of space characters to use as white space; this number is capped at 10 (if it is greater, the value is just 10). Values less than 1
* indicate that no space should be used.
* @return A JSON string representing the given value.
*/
@JsOverlay
public static String stringify(Object obj, int spaces) {
// checks if object id consistent
if (obj != null) {
// format in JSON
return stringify(obj, null, spaces);
}
// if here, the argument is null
// then returns an empty JSON
return JSONReplacerConstants.EMPTY_JSON_OBJECT;
}
/**
* Converts a JavaScript object or value to a JSON string, the space value is set to -1 that no space should be used and uses a default replacer to avoid
* TypeError: cyclic object value
.
*
* @param obj The value to convert to a JSON string.
* @return A JSON string representing the given value.
*/
@JsOverlay
public static String stringifyWithReplacer(Object obj) {
return stringifyWithReplacer(obj, -1);
}
/**
* Converts a JavaScript object or value to a JSON string, using a default replacer to avoid TypeError: cyclic object value
.
*
* @param obj The value to convert to a JSON string.
* @param spaces it indicates the number of space characters to use as white space; this number is capped at 10 (if it is greater, the value is just 10). Values less than 1
* indicate that no space should be used.
* @return A JSON string representing the given value.
*/
@JsOverlay
public static String stringifyWithReplacer(Object obj, int spaces) {
// checks if object id consistent
if (obj != null) {
// creates a cached to checks if an object was already parsed
final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy