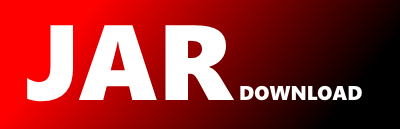
org.perfectable.introspection.bean.BeanSchema Maven / Gradle / Ivy
package org.perfectable.introspection.bean;
import java.util.Objects;
import static com.google.common.base.Preconditions.checkArgument;
import static java.util.Objects.requireNonNull;
import static org.perfectable.introspection.Introspections.introspect;
/**
* Schema of a Java Bean.
*
* This class represents view of a java class from perspective of Java Beans. This means that instead of class
* fields, methods, constructors and so on, bean schema has type and {@link PropertySchema}, and can be instantiated,
*
* @param class that this schema covers
*/
public final class BeanSchema {
private final Class beanClass;
/**
* Creates schema from provided class.
*
* @param beanClass class to create schema from
* @param type of schema
* @return Bean schema for provided class
*/
public static BeanSchema from(Class beanClass) {
requireNonNull(beanClass);
return new BeanSchema<>(beanClass);
}
private BeanSchema(Class beanClass) {
this.beanClass = beanClass;
}
/**
* Inserts bean instance into this schema, creating actual {@link Bean}.
*
* @param element element to convert to bean
* @return bean from the element
*/
public Bean put(B element) {
checkArgument(this.beanClass.isInstance(element));
return Bean.from(element);
}
/**
* Class backing this schema.
*
* @return schema class
*/
public Class type() {
return beanClass;
}
/**
* Creates new empty bean from this schema.
*
* This will actually invoke parameterless constructor of the class.
*
* @return new bean from this schema
*/
public Bean instantiate() {
B instance = introspect(beanClass).instantiate();
return Bean.from(instance);
}
/**
* Extracts property schema by name.
*
* @param name name of the schema searched
* @return property schema for provided name
* @throws IllegalArgumentException when property with this name doesn't exist in bean schema.
*/
public PropertySchema property(String name) {
return Properties.create(this.beanClass, name);
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof BeanSchema>)) {
return false;
}
BeanSchema> other = (BeanSchema>) obj;
return beanClass.equals(other.beanClass);
}
@Override
public int hashCode() {
return Objects.hash(beanClass);
}
}