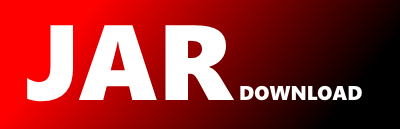
org.perfectable.introspection.proxy.Invocations Maven / Gradle / Ivy
package org.perfectable.introspection.proxy;
import java.util.concurrent.Callable;
import java.util.function.Supplier;
final class Invocations {
static final class Returning implements Invocation {
private final T result;
Returning(T result) {
this.result = result;
}
@Override
public T invoke() {
return result;
}
}
static final class Throwing implements Invocation {
private final Supplier thrownSupplier;
Throwing(Supplier thrownSupplier) {
this.thrownSupplier = thrownSupplier;
}
@Override
public Void invoke() throws X {
throw thrownSupplier.get();
}
}
static final class CallableAdapter implements Invocation {
private final Callable callable;
CallableAdapter(Callable callable) {
this.callable = callable;
}
@Override
public R invoke() throws Exception {
return callable.call();
}
}
static final class RunnableAdapter implements Invocation {
private final Runnable runnable;
RunnableAdapter(Runnable runnable) {
this.runnable = runnable;
}
@Override
public Void invoke() {
runnable.run();
return null;
}
}
static final class Casting implements Invocation {
private final Invocation parent;
private final Class targetCast;
Casting(Invocation parent, Class targetCast) {
this.parent = parent;
this.targetCast = targetCast;
}
@Override
public S invoke() throws X {
@SuppressWarnings("assignment")
R result = parent.invoke();
return targetCast.cast(result);
}
}
private Invocations() {
// utility class
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy