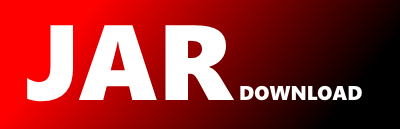
org.perro.functions.mapper.LongTransformUtils Maven / Gradle / Ivy
package org.perro.functions.mapper;
import org.perro.functions.collector.CollectorUtils;
import org.perro.functions.stream.LongStreamUtils;
import org.perro.functions.stream.StreamUtils;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import java.util.function.LongFunction;
import java.util.function.LongUnaryOperator;
import java.util.stream.Collector;
import static java.util.stream.Collectors.toList;
import static java.util.stream.Collectors.toSet;
import static org.perro.functions.collector.CollectorUtils.toMapFromEntry;
import static org.perro.functions.mapper.LongMapperUtils.*;
import static org.perro.functions.stream.LongStreamUtils.defaultLongStream;
import static org.perro.functions.stream.StreamUtils.defaultStream;
/**
* Methods to transform an array of longs to a collection of elements of an arbitrary type, or to transform a collection
* of elements of an arbitrary type to an array of longs.
*/
public final class LongTransformUtils {
private LongTransformUtils() {
}
/**
* Given an array of longs, and a LongUnaryOperator
that transforms each of those longs to another long,
* returns an array of the resulting long
values.
*
* @param longs An array of ints to be transformed.
* @param operator A LongUnaryOperator that takes a long and transforms it to another long.
* @return An array of primitive transformed longs.
*/
public static long[] longUnaryTransform(long[] longs, LongUnaryOperator operator) {
return defaultLongStream(longs)
.map(operator)
.toArray();
}
/**
* Given an array of longs, and a LongUnaryOperator
that transforms each of those longs to another
* long, returns an array of the resulting long
values, which will contain only distinct values.
*
* @param longs An array of ints to be transformed.
* @param operator A LongUnaryOperator that takes a long and transforms it to another long.
* @return An array of distinct, primitive, transformed longs.
*/
public static long[] longUnaryTransformDistinct(long[] longs, LongUnaryOperator operator) {
return defaultLongStream(longs)
.map(operator)
.distinct()
.toArray();
}
/**
* Given an array of longs, and a LongFunction<R>
that transforms those longs into elements of
* type <R>, returns a List<R>
of those elements.
*
* @param longs An array of longs to be transformed.
* @param transformer A LongFunction<R> that takes a long and transforms it to an element of type <R>.
* @param The type of the elements in the resulting List.
* @return A List<R> of elements transformed from an array of primitive longs.
*/
public static List longTransform(long[] longs, LongFunction transformer) {
return longTransform(longs, LongTransformerCollector.of(transformer, toList()));
}
/**
* Given an array of longs, and a LongFunction<R>
that transforms those longs into elements of
* type <R>, returns a Set<R>
of those elements.
*
* @param longs An array of longs to be transformed.
* @param transformer A LongFunction<R> that takes a long and transforms it to an element of type <R>.
* @param The type of the elements in the resulting Set.
* @return A Set<R> of elements transformed from an array of primitive longs.
*/
public static Set longTransformToSet(long[] longs, LongFunction transformer) {
return longTransform(longs, LongTransformerCollector.of(transformer, toSet()));
}
/**
* Given an array of longs, and a Collector<Long, ?, C>
, returns a
* Collection<C>
of Long
elements.
*
* @param longs An array of longs to be collected.
* @param collector A Collector<Long, ?, C> that appends Long values into a collection of type <C>.
* @param The type of the collection of Long values returned.
* @return A collection of type <C> of Long values.
*/
public static > C longTransform(long[] longs, Collector collector) {
return longTransform(longs, LongTransformerCollector.of(Long::valueOf, collector));
}
/**
* Given an array of longs, and a LongFunction<R>
that transforms those longs into elements of
* type <R>, returns a List<R>
of those elements, which will contain only unique values
* (according to Object.equals(Object)
).
*
* @param longs An array of longs to be transformed.
* @param transformer A LongFunction<R> that takes a long and transforms it to an element of type <R>.
* @param The type of the elements in the resulting List.
* @return A List<R> of elements transformed from an array of primitive longs, containing only distinct
* elements.
*/
public static List longTransformDistinct(long[] longs, LongFunction transformer) {
return defaultLongStream(longs)
.mapToObj(longMapper(transformer))
.distinct()
.collect(toList());
}
/**
* Given an array of longs, and a LongTransformerCollector<U, C>
that contains a
* LongFunction<U>
to transform long values into elements of type <U>, and a
* Collector<U, ?, C>
, returns a collection of type <C> of those elements. The
* transformerCollection
passed into this method should be created via a call to
* {@link #longTransformAndThen(LongFunction, Collector)}.
*
* @param longs An array of longs to be transformed.
* @param transformerCollector A object containing a transformer LongFunction<U> to transform long values into
* elements of type <U>, and a Collector<U, ?, C> to put those elements into
* a resulting collection.
* @param The type of the elements in the resulting Collection.
* @param The type of the Collection of elements of type <U> returned.
* @return A collection of type <C> of elements <U>, transformed from an array of primitive longs.
*/
public static > C longTransform(long[] longs, LongTransformerCollector transformerCollector) {
return defaultLongStream(longs)
.mapToObj(transformerCollector.getTransformer())
.collect(transformerCollector.getCollector());
}
/**
* Given an array of longs, and a LongFunction<int[]>
(a function that takes a long and returns
* an array of longs), returns an array of longs.
*
* @param longs An array of primitive longs to be flat-mapped into another array of longs.
* @param function A LongFunction that takes a long and returns an array of longs.
* @return An array of flat-mapped primitive longs.
*/
public static long[] longFlatMap(long[] longs, LongFunction function) {
return defaultLongStream(longs)
.flatMap(longFlatMapper(function))
.toArray();
}
/**
* Given an array of longs, and a LongFunction<long[]>
(a function that takes a long and returns
* an array of longs), returns an array of distinct primitive long values.
*
* @param longs An array of primitive longs to be flat-mapped into another array of longs.
* @param function A LongFunction that takes a long and returns an array of longs.
* @return An array of distinct, primitive, flat-mapped longs.
*/
public static long[] longFlatMapDistinct(long[] longs, LongFunction function) {
return defaultLongStream(longs)
.flatMap(longFlatMapper(function))
.distinct()
.toArray();
}
/**
* Given a collection of objects of type <T>, and a Function<T, long[]>
, returns an array
* of primitive longs.
*
* @param objects A collection of objects of type <T>, to be flat-mapped into an array of primitive longs.
* @param function A Function that takes an element of type <T> and returns a primitive long array.
* @param The type of the elements in the given objects collection.
* @return An array of primitive flat-mapped longs.
*/
public static long[] flatMapToLong(Collection objects, Function function) {
return defaultStream(objects)
.flatMapToLong(flatMapperToLong(function))
.toArray();
}
/**
* Given a collection of objects of type <T>, and a Function<T, long[]>
, returns an array
* of distinct primitive longs.
*
* @param objects A collection of objects of type <T>, to be flat-mapped into an array of primitive longs.
* @param function A Function that takes an element of type <T> and returns a primitive long array.
* @param The type of the elements in the given objects collection.
* @return An array of distinct, primitive, flat-mapped longs.
*/
public static long[] flatMapToLongDistinct(Collection objects, Function function) {
return defaultStream(objects)
.flatMapToLong(flatMapperToLong(function))
.distinct()
.toArray();
}
/**
* Builds a LongTransformerCollector<U, C>
that contains a LongFunction<U>
to
* transform primitive long values into elements of type <U>, and a Collector<U, ?, C>
to
* collect those elements into a collection of type <C>. Used to build the second parameter to
* {@link #longTransform(long[], LongTransformerCollector)}.
*
* @param transformer A LongFunction<U>
to transform primitive long values into elements of type
* <U>.
* @param collector A Collector<U, ?, C> to append elements into a collection of type <C>.
* @param The type of the elements into which int values are to be transformed.
* @param The type of a collection of elements of type <U>, into which a given collector will
* append elements.
* @return An object containing a LongFunction<U>
to transform primitive long values into
* elements of type <U>, and a Collector<U, ?, C>
to append those elements into a
* collection of type <C>.
*/
public static > LongTransformerCollector longTransformAndThen(LongFunction transformer, Collector collector) {
return LongTransformerCollector.of(transformer, collector);
}
/**
* Given an array of primitive longs, and a LongKeyValueMapper
containing a LongFunction
* to transform a long to a key of type <K>, and another LongFunction
to transform a long to a
* value of type <V>, returns a Map<K, V>
.
*
* @param longs An array of primitive longs to be transformed into keys and values for the resulting
* Map<K, V>.
* @param keyValueMapper An object containing a LongFunction to transform a long to a key of type <K>,
* and another LongFunction to transform a long to a value of type <V>.
* @param The type of the key of the resulting Map<K, V>.
* @param The type of the value of the resulting Map<K, V>.
* @return A map whose keys and values are transformed from an array of longs.
*/
public static Map longTransformToMap(long[] longs, LongKeyValueMapper keyValueMapper) {
return defaultLongStream(longs)
.mapToObj(longPairOf(keyValueMapper))
.collect(toMapFromEntry());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy