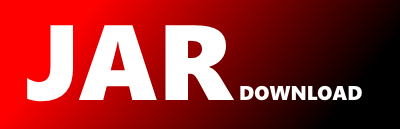
org.phoenix.model.CaseBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of phoenix_webdriver Show documentation
Show all versions of phoenix_webdriver Show documentation
update:检查点bug修复,增加js执行的驱动,commandExecutor方法bug修复,驱动更新支持最新Firefox47/chrome51/IE10/IE11/IE Edge
package org.phoenix.model;
import java.util.Date;
import java.util.Set;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.OneToMany;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
import org.hibernate.annotations.BatchSize;
import org.hibernate.annotations.Fetch;
import org.hibernate.annotations.FetchMode;
import org.hibernate.annotations.LazyCollection;
import org.hibernate.annotations.LazyCollectionOption;
import org.phoenix.enums.MsgSendType;
@Entity
@Table(name="t_web_case")
@BatchSize(size=30)
public class CaseBean {
private int id;
private String caseName;
private String codeContent;
private String className;
private String caseType;
private MsgSendType msgSendType;
private boolean isDeleteMsg;
private String remark;
private int status = 1;
private Date createDate;
private ScenarioBean scenarioBean;
private int userId;
private Set locatorBeans;
private Set dataBeans;
public CaseBean() {
// TODO Auto-generated constructor stub
}
public CaseBean(String caseName, String codeContent, String remark ) {
super();
this.caseName = caseName;
this.codeContent = codeContent;
this.remark = remark;
}
public CaseBean(String caseName, String remark, int status, Date createDate,
ScenarioBean scenarioBean, int userId) {
super();
this.caseName = caseName;
this.remark = remark;
this.status = status;
this.createDate = createDate;
this.scenarioBean = scenarioBean;
this.userId = userId;
}
public CaseBean(String caseName, String codeContent, String remark, int status ,Date createDate,
ScenarioBean scenarioBean, int userId) {
super();
this.caseName = caseName;
this.codeContent = codeContent;
this.remark = remark;
this.status = status;
this.createDate = createDate;
this.scenarioBean = scenarioBean;
this.userId = userId;
}
@Id
@GeneratedValue
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
@ManyToOne(fetch=FetchType.EAGER)
@JoinColumn(name="scenarioId")
@LazyCollection(LazyCollectionOption.FALSE)
public ScenarioBean getScenarioBean() {
return scenarioBean;
}
public void setScenarioBean(ScenarioBean scenarioBean) {
this.scenarioBean = scenarioBean;
}
@OneToMany(mappedBy="caseBean",targetEntity=LocatorBean.class)
@LazyCollection(LazyCollectionOption.FALSE)
@Fetch(FetchMode.SUBSELECT)
public Set getLocatorBeans() {
return locatorBeans;
}
public void setLocatorBeans(Set locatorBeans) {
this.locatorBeans = locatorBeans;
}
@OneToMany(mappedBy="caseBean",targetEntity=DataBean.class)
@LazyCollection(LazyCollectionOption.FALSE)
@Fetch(FetchMode.SUBSELECT)
public Set getDataBeans() {
return dataBeans;
}
public void setDataBeans(Set dataBeans) {
this.dataBeans = dataBeans;
}
public int getStatus() {
return status;
}
public void setStatus(int status) {
this.status = status;
}
@Column(unique=true,nullable=false)
public String getCaseName() {
return caseName;
}
public void setCaseName(String caseName) {
this.caseName = caseName;
}
public String getCaseType() {
return caseType;
}
public void setCaseType(String caseType) {
this.caseType = caseType;
}
@Column(name="codeContent",length=65500,nullable=true)
public String getCodeContent() {
return codeContent;
}
public void setCodeContent(String codeContent) {
this.codeContent = codeContent;
}
public int getUserId() {
return userId;
}
public void setUserId(int userId) {
this.userId = userId;
}
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className;
}
@Temporal(TemporalType.TIMESTAMP)
@Column(name="create_date")
public Date getCreateDate() {
return createDate;
}
public void setCreateDate(Date createDate) {
this.createDate = createDate;
}
public String getRemark() {
return remark;
}
public void setRemark(String remark) {
this.remark = remark;
}
public MsgSendType getMsgSendType() {
return msgSendType;
}
public void setMsgSendType(MsgSendType msgSendType) {
this.msgSendType = msgSendType;
}
public boolean isDeleteMsg() {
return isDeleteMsg;
}
public void setDeleteMsg(boolean isDeleteMsg) {
this.isDeleteMsg = isDeleteMsg;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy