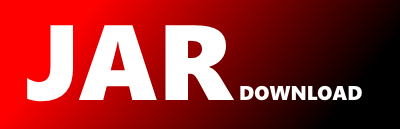
org.pipservices4.config.auth.CredentialResolver Maven / Gradle / Ivy
Show all versions of pip-services4-config Show documentation
package org.pipservices4.config.auth;
import org.pipservices4.components.config.ConfigParams;
import org.pipservices4.components.config.IConfigurable;
import org.pipservices4.commons.errors.ApplicationException;
import org.pipservices4.components.context.IContext;
import org.pipservices4.components.refer.Descriptor;
import org.pipservices4.components.refer.IReferenceable;
import org.pipservices4.components.refer.IReferences;
import org.pipservices4.components.refer.ReferenceException;
import java.util.ArrayList;
import java.util.List;
/**
* Helper class to retrieve component credentials.
*
* If credentials are configured to be retrieved from ICredentialStore,
* it automatically locates {@link ICredentialStore} in component references
* and retrieve credentials from there using store_key
parameter.
*
* ### Configuration parameters ###
*
* - credential:
*
* - store_key: (optional) a key to retrieve the credentials from {@link ICredentialStore}
*
- ... other credential parameters
*
* - credentials: alternative to credential
*
* - [credential params 1]: first credential parameters
*
- ...
*
- [credential params N]: Nth credential parameters
*
- ...
*
*
*
* ### References ###
*
* - *:credential-store:*:*:1.0 (optional) Credential stores to resolve credentials
*
*
* ### Example ###
*
* {@code
* ConfigParams config = ConfigParams.fromTuples(
* "credential.user", "jdoe",
* "credential.pass", "pass123"
* );
*
* CredentialResolver credentialResolver = new CredentialResolver();
* credentialResolver.configure(config);
* credentialResolver.setReferences(references);
*
* credentialResolver.lookup(ContextResolver.fromTraceId("123"));
* }
*
*
* @see CredentialParams
* @see ICredentialStore
*/
public class CredentialResolver implements IConfigurable, IReferenceable {
private final List _credentials = new ArrayList<>();
private IReferences _references = null;
/**
* Creates a new instance of credentials resolver.
*/
public CredentialResolver() {
}
/**
* Creates a new instance of credentials resolver.
*
* @param config (optional) component configuration parameter.
*/
public CredentialResolver(ConfigParams config) {
configure(config);
}
/**
* Creates a new instance of credentials resolver.
*
* @param config (optional) component configuration parameters
* @param references (optional) component references
*/
public CredentialResolver(ConfigParams config, IReferences references) {
if (config != null)
this.configure(config);
if (references != null)
this.setReferences(references);
}
/**
* Configures component by passing configuration parameters.
*
* @param config configuration parameters to be set.
* @param configAsDefault boolean parameter for default configuration. If "true"
* the default value will be added to the result.
*/
public void configure(ConfigParams config, boolean configAsDefault) {
_credentials.addAll(CredentialParams.manyFromConfig(config, configAsDefault));
}
/**
* Configures component by passing configuration parameters.
*
* @param config configuration parameters to be set.
*/
public void configure(ConfigParams config) {
configure(config, false);
}
/**
* Sets references to dependent components.
*
* @param references references to locate the component dependencies.
*/
public void setReferences(IReferences references) {
_references = references;
}
/**
* Gets all credentials configured in component configuration.
*
* Redirect to CredentialStores is not done at this point. If you need fully
* fleshed credential use lookup() method instead.
*
* @return a list with credential parameters
*/
public List getAll() {
return _credentials;
}
/**
* Adds a new credential to component credentials
*
* @param connection new credential parameters to be added
*/
public void add(CredentialParams connection) {
_credentials.add(connection);
}
private CredentialParams lookupInStores(IContext context, CredentialParams credential)
throws ApplicationException {
if (!credential.useCredentialStore())
return null;
String key = credential.getStoreKey();
if (_references == null)
return null;
List