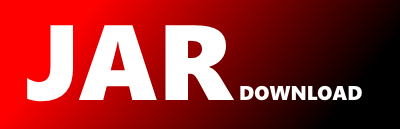
com.orgname.cruddata.controllers.EntitiesHttpController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of service-cruddata-springboot Show documentation
Show all versions of service-cruddata-springboot Show documentation
Demo cruddata service project for Spring Boot
The newest version!
package com.orgname.cruddata.controllers;
import com.orgname.cruddata.data.CustomPage;
import com.orgname.cruddata.data.EntityV1;
import com.orgname.cruddata.services.EntitiesService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.http.HttpStatus;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.*;
import java.util.Map;
import java.util.Optional;
@RestController
@RequestMapping(path = "v1/entities")
//@ConditionalOnProperty(value = "components.http-controller.type", havingValue = "entities")
public class EntitiesHttpController {
@Autowired
private EntitiesService entitiesService;
public EntitiesHttpController(EntitiesService entitiesService) {
this.entitiesService = entitiesService;
}
protected EntitiesHttpController() {
}
@PostMapping(path = "/get_entities")
@ResponseStatus(HttpStatus.OK)
public CustomPage getEntities(@RequestHeader(value = "trace_id", required = false) String traceId,
@RequestBody Map> body) {
var paging = body.get("paging");
var filter = body.get("filter");
Page page = entitiesService.getEntities(traceId, filter, paging);
return new CustomPage<>(page.getContent(), page.getTotalPages(), page.getTotalElements(), page.getSize(), page.getNumber());
}
@GetMapping(path = "/get_entity_by_id/{entity_id}")
@ResponseStatus(HttpStatus.OK)
public Optional getEntityById(@RequestHeader(value = "trace_id", required = false) String traceId,
@PathVariable String entity_id) {
return entitiesService.getEntityById(traceId, entity_id);
}
@PostMapping(path = "/create_entity")
@ResponseStatus(HttpStatus.CREATED)
public EntityV1 createEntity(@RequestHeader(value = "trace_id", required = false) String traceId,
@RequestBody @Validated EntityV1 entity) {
return entitiesService.createEntity(traceId, entity);
}
@PutMapping(path = "/update_entity")
@ResponseStatus(HttpStatus.OK)
public Optional updateEntity(@RequestHeader(value = "trace_id", required = false) String traceId,
@RequestBody EntityV1 entity) {
return entitiesService.updateEntity(traceId, entity);
}
@DeleteMapping(path = "/delete_entity_by_id/{entity_id}")
@ResponseStatus(HttpStatus.OK)
public Optional deleteEntityById(@RequestHeader(value = "trace_id") String traceId,
@PathVariable String entity_id) {
return entitiesService.deleteEntityById(traceId, entity_id);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy