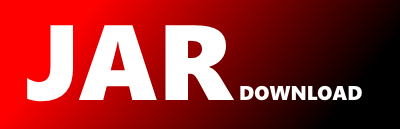
com.orgname.cruddata.data.EntityV1 Maven / Gradle / Ivy
package com.orgname.cruddata.data;
import com.fasterxml.jackson.annotation.JsonProperty;
import jakarta.persistence.*;
import org.hibernate.Hibernate;
import java.util.Objects;
import java.util.UUID;
@Entity
@Table(name = "entities", indexes = {
@Index(name = "entities_site_id", columnList = "siteId")
})
public class EntityV1 {
@Id
@Column
private String id;
public EntityV1() {
}
public EntityV1(String id, String siteId, String type, String name, String content) {
this.id = id;
this.siteId = siteId;
this.type = type;
this.name = name;
this.content = content;
}
@PrePersist
protected void onCreate() {
if (this.id == null || this.id.isEmpty())
this.id = String.valueOf(UUID.randomUUID());
}
@Column
@JsonProperty("site_id")
private String siteId;
@Column
private String type;
@Column
private String name;
@Column
private String content;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getSiteId() {
return siteId;
}
public void setSiteId(String siteId) {
this.siteId = siteId;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || Hibernate.getClass(this) != Hibernate.getClass(o)) return false;
EntityV1 entityV1 = (EntityV1) o;
return id != null && Objects.equals(id, entityV1.id);
}
@Override
public int hashCode() {
return getClass().hashCode();
}
@Override
public String toString() {
return "Employee{" +
"id=" + id +
", siteId='" + siteId + '\'' +
", name='" + name + '\'' +
", type='" + type + '\'' +
", content=" + content +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy