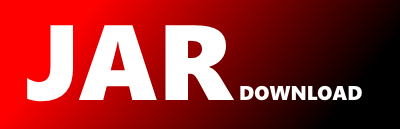
com.orgname.cruddata.repositories.EntitiesMongoDbRepository Maven / Gradle / Ivy
package com.orgname.cruddata.repositories;
import com.orgname.cruddata.data.EntityV1;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.context.annotation.Primary;
import org.springframework.data.domain.Example;
import org.springframework.data.domain.ExampleMatcher;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.data.repository.CrudRepository;
import org.springframework.data.repository.PagingAndSortingRepository;
import org.springframework.data.repository.query.QueryByExampleExecutor;
import org.springframework.stereotype.Repository;
import java.util.Map;
import java.util.Optional;
@Repository
//@Primary
//@ConditionalOnProperty(prefix = "app", value = "repository", havingValue = "mongo")
public interface EntitiesMongoDbRepository extends MongoRepository,
CrudRepository, IEntitiesRepository,
PagingAndSortingRepository, QueryByExampleExecutor {
default Example _composeFilter(Map filter) {
if (filter != null) {
var matcher = ExampleMatcher.matching();
var matcherObject = new EntityV1();
var id = filter.get("id");
var siteId = filter.get("site_id");
var name = filter.get("name");
if (id != null && !id.isEmpty()) {
matcher.withMatcher("id", ExampleMatcher.GenericPropertyMatchers.exact());
matcherObject.setId(id);
}
if (siteId != null && !siteId.isEmpty()) {
matcher.withMatcher("site_id", ExampleMatcher.GenericPropertyMatchers.exact());
matcherObject.setSiteId(siteId);
}
if (name != null && !name.isEmpty()) {
matcher.withMatcher("name", ExampleMatcher.GenericPropertyMatchers.exact());
matcherObject.setName(name);
}
return Example.of(matcherObject, matcher);
}
return null;
}
@Override
default Page findByFilter(Map filter, Pageable paging) {
var filterObj = _composeFilter(filter);
if (filterObj != null)
return findAll(_composeFilter(filter), paging);
else
return findAll(paging);
}
@Override
default Optional update(EntityV1 entity) {
if (this.existsById(entity.getId()))
return Optional.of(this.save(entity));
else
return Optional.empty();
}
@Override
default EntityV1 create(EntityV1 entity) {
return this.save(entity);
}
@Override
default Optional findEntityById(String entityId) {
return this.findById(entityId);
}
@Override
default Optional deleteEntityById(String entityId) {
var entity = this.findById(entityId);
if (entity.isPresent()) {
this.deleteById(entityId);
return entity;
}
else
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy