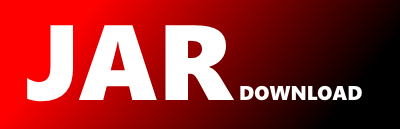
com.orgname.cruddata.services.EntitiesService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of service-cruddata-springboot Show documentation
Show all versions of service-cruddata-springboot Show documentation
Demo cruddata service project for Spring Boot
The newest version!
package com.orgname.cruddata.services;
import com.orgname.cruddata.data.EntityV1;
import com.orgname.cruddata.repositories.IEntitiesRepository;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.stereotype.Service;
import java.util.Map;
import java.util.Optional;
@Service
public class EntitiesService {
private final IEntitiesRepository repository;
Logger logger = LoggerFactory.getLogger(EntitiesService.class);
@Autowired
public EntitiesService(IEntitiesRepository repository) {
this.repository = repository;
}
public Page getEntities(String traceId, Map filter, Map paging) {
var numPage = paging != null && paging.get("page") != null ? Integer.parseInt(paging.get("page")) : 0;
var pageSize = paging != null && paging.get("size") != null ? Integer.parseInt(paging.get("size")) : 100;
var res = repository.findByFilter(filter, PageRequest.of(numPage, pageSize));
logger.info(traceId + " : " + "Found " + res.stream().count() + "entities");
return res;
}
public Optional getEntityById(String traceId, String entityId) {
var res = repository.findEntityById(entityId);
res.ifPresentOrElse(
entityV1 -> logger.info(traceId + " : " + "Entity with id: " + entityId),
() -> logger.info(traceId + " : " + "Nothing found with id: " + entityId)
);
return res;
}
public EntityV1 createEntity(String traceId, EntityV1 entity) {
var res = repository.create(entity);
logger.info(traceId + " : " + "Created entity with id: " + entity.getId());
return res;
}
public Optional updateEntity(String traceId, EntityV1 entity) {
var res = repository.update(entity);
if (res.isPresent()) {
logger.info(traceId + " : " + "Updated entity with id: " + entity.getId());
return res;
} else {
logger.info(traceId + " : " + "Entity not found, id: " + entity.getId());
return Optional.empty();
}
}
public Optional deleteEntityById(String traceId, String entityId) {
return repository.deleteEntityById(entityId);
// if (entity.isPresent()) {
// repository.deleteById(entityId);
// logger.info(traceId + " : " + "Deleted entity with id: " + entityId);
// } else {
// logger.info(traceId + " : " + "Entity not found for deletion, id: " + entityId);
// }
//
// return entity;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy